For loop in C
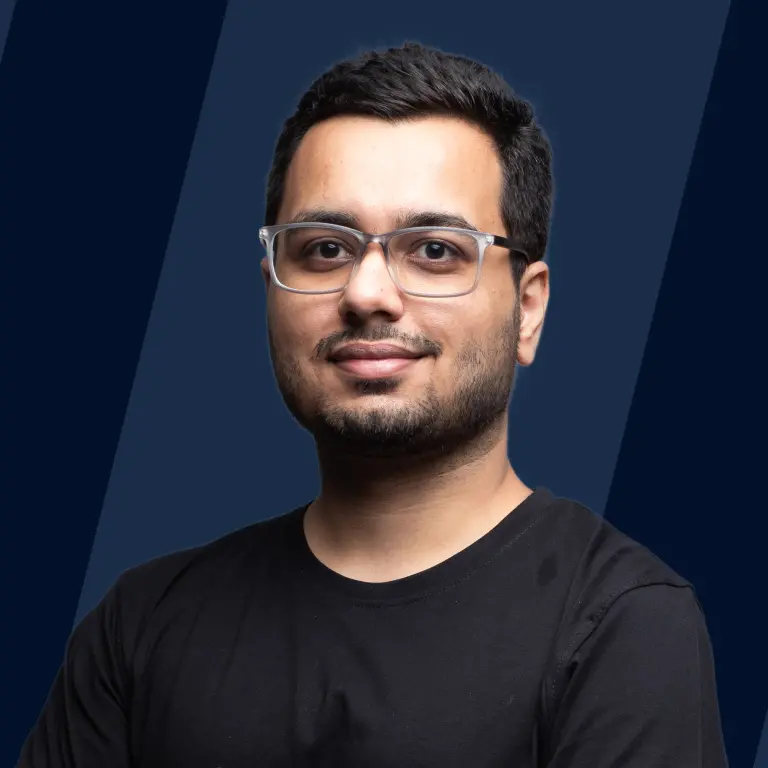
A for loop in C is a control structure that is used to run a block of instructions multiple times until a certain condition fails. In this article, we will be learning about loops in c.
Syntax of for loop in C
We have discussed, what is for loop in C?
Now let's discuss the Syntax of for loop in C is as follows:
Structure of For Loop in C
Aside from the above syntax, there are many other ways we can run for loops. Some don't require an initialization, some don't require an updation, and some don't require both! Here are a few ways to run for loops:
A) NO INITIALIZATION: Initialization can be skipped, as shown below:
B) NO UPDATION: We can run a for loop without needing an updation in the following way:
C) NO INITIALIZATION AND UPDATE STATEMENT: We can avoid both initialization and update statements too!
We can see that this syntax is similar to the while loop if we notice carefully. Using a while loop is a better choice than running a for loop with this syntax.
How for Loop Work?
Here's how the for loop works in C:
- Step 1: Initialization - At the beginning of the loop, variables are declared or initialized with a starting value. This step occurs only once.
- Step 2: Condition Check - Before each iteration, the condition statement is checked, and if the condition is true, then only the loop continues, otherwise, the loop will break and the control exits the loop.
- Step 3: Execution of Statements - All the statements inside the loop body are executed.
- Step 4: Update - After executing the statements inside the loop, the loop control variable(s) are updated as defined in the loop (e.g., incrementing or decrementing a counter variable).
- Continue to Step 2: After completing all the steps, the loop control returns to Step 2, where the condition is rechecked.
- If the condition is still true, the loop continues; otherwise, it exits.
- This cycle continues until the condition evaluates to false, at which point the loop terminates.
Flow Chart of for Loop in C
for Loop Example in C
Let's discuss the for loop example in C.
For the case of the company wanting to calculate the salary and bonus of their employees we have taken the above:
OUTPUT:
Special Conditions with C For Loops
1. for loop without curly braces
In C, it's possible to use a for loop without enclosing the loop body within curly braces if there's only a single statement to execute. For example:
This code snippet prints numbers from 0 to 4. While it's allowed in C, it's generally considered good practice to enclose loop bodies within curly braces for clarity and to avoid potential issues with unintended behaviour.
2. Infinite for loop / Null Parameter Loop
An infinite loop in C can be created using a for loop with no initialization, condition, or iteration step specified. For example:
This loop will continue to execute indefinitely until it's terminated externally (e.g., by interrupting the program). Infinite loops are typically avoided in most programming scenarios, but they can be useful in situations where continuous execution is required, such as embedded systems or server applications. However, caution must be practised to prevent consequences, such as resource exhaustion or system crashes.
Advantages of For Loops
-
Readability: for Loops offer a clear and concise syntax, enhancing code readability compared to while or do-while loops, especially for iterating over sequences or collections.
-
Controlled Iteration: They provide precise control over loop iteration, with explicit initialization, termination conditions, and iteration steps, making it easier to manage loop behaviour.
-
Compactness: for loops typically require fewer lines of code than equivalent while loops, simplifying code maintenance and reducing the likelihood of errors.
-
Efficiency: for loops often result in faster execution compared to other loop types due to their optimized structure, especially when iterating over arrays or other indexed data structures.
-
Scope Management: Variables declared within the for loop initialization are confined to the loop's scope, preventing unintentional variable exposure and potential conflicts outside the loop.
Limitations
- Initialization Overhead: for loops require explicit initialization, which can sometimes lead to additional overhead, especially when dealing with complex initialization expressions.
- Fixed Iteration: For loops are primarily suited for situations where the number of iterations is known beforehand. They may be unsuitable for cases where the iteration count depends on dynamic conditions.
- Complexity for Non-Linear Iteration: While for loops excel at linear iteration (e.g., iterating over arrays), they can become cumbersome when dealing with non-linear iteration patterns or when skipping or repeating iterations.
- Scope Leakage: Variables declared within the initialization expression of a for loop remain accessible after the loop completes its execution, potentially leading to unintended side effects or scope leakage.
- Potential for Infinite Loops: Incorrectly defined termination conditions or iteration steps can lead to infinite loops, causing the program to hang or crash. Careful attention is required to ensure proper termination conditions are set.
Conclusion
- for loops are used to iterate over a sequence.
- It iterates a preset number of times and stops as soon as conditions are met.
- Without a condition, the loop will iterate indefinitely until it encounters the break command.
- Loops run to produce a result or to satisfy a condition or set of requirements.