JavaScript Statements
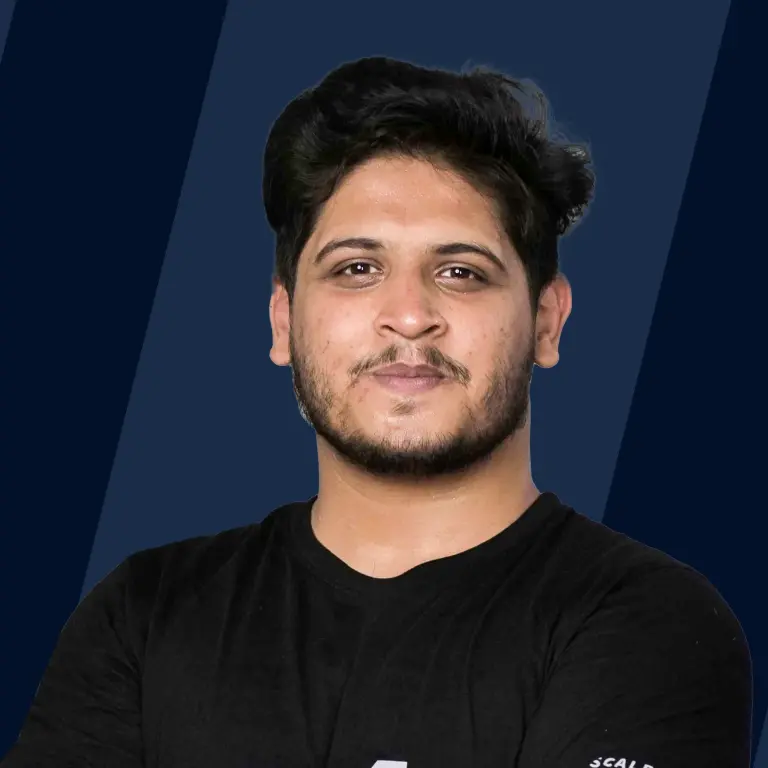
Statements in Javascript comprise Values, Operators, Keywords, Expressions, and Comments. They execute sequentially, adhering to the order of appearance in the code. Values represent data, Operators perform operations, Keywords control flow, and Expressions combine elements to produce results. Comments provide non-executable notes or documentation within the code.
JavaScript Statements
Semicolons
Semicolons serve to separate statements in javascript, denoting the end of each statement.
This yields the output:
Additionally, JavaScript allows multiple statements on a single line if separated by semicolons, as illustrated here:
Which results in:
JavaScript Code Blocks
Code Blocks in JavaScript serve to unite statements within curly brackets, allowing multiple statements to be executed as a cohesive entity. Consider the following example:
This results in the following output:
Additionally, code blocks can enclose various javascript statements, as demonstrated below:
Here, if the condition is true, both "Statement 1" and "Statement 2" will be executed together.
JavaScript White Space
In JavaScript, multiple white spaces are disregarded, maintaining code readability without affecting functionality. For instance:
Outputs:
Despite the varying white spaces around the assignment, the output remains consistent. JavaScript treats them as equivalent, preserving code clarity while being lenient with spacing.
JavaScript Line Length and Line Breaks
In JavaScript coding practices, it's commonly preferred to limit lines to 80 characters for better readability. When a line exceeds this limit, the recommended approach is to break it after an operator. For instance:
Breaking the line after operators ensures that the code remains tidy and comprehensible, especially in complex calculations or expressions. This practice promotes maintainable and easily understandable code.
JavaScript Keywords
Keywords in JavaScript are predetermined reserved terms that carry specific functionalities and cannot be repurposed as variable names. These words dictate the nature of operations to be executed within the code.
Some Commonly Used Keywords
Here's a brief description of each commonly used JavaScript keyword:
- break and continue: Used within loops (such as for or while) to control the flow of iteration. break terminates the loop prematurely, while continue skips the current iteration and proceeds to the next one.
- do… while: Executes a block of code once before checking the condition. It continues to execute the block while the specified condition remains true.
- for: Creates a loop that consists of three optional expressions enclosed in parentheses, defining initialization, condition, and iteration. It continuously runs a block of code as long as the condition holds true.
- function: Declares a reusable block of code that can be invoked multiple times throughout the program. Functions may accept parameters and can return values.
- return: Terminates the execution of a function and returns a value to the caller. It's used to output a value from a function.
- switch: Provides a way to execute different code blocks based on different conditions. It evaluates an expression, matching its value to a case clause, and executes the corresponding block of code.
- var, let, and const: Keywords used for variable declaration. var is used to declare variables with function scope, let declares block-scoped variables, and const declares variables with constant values, whose value cannot be reassigned.
These keywords are fundamental to structuring and controlling the flow of JavaScript programs.
Conclusion
- JavaScript Statements Overview: Statements in JavaScript comprise various elements and execute sequentially.
- Semicolons and Code Blocks: Semicolons separate statements in javascript, while code blocks group javascript statements for cohesive execution.
- White Space Handling: JavaScript disregards multiple white spaces, maintaining readability.
- Common Keywords: Familiarize with keywords like break, continue, for, function, return, switch, var, let, and const for effective programming.