Javascript 2D Array
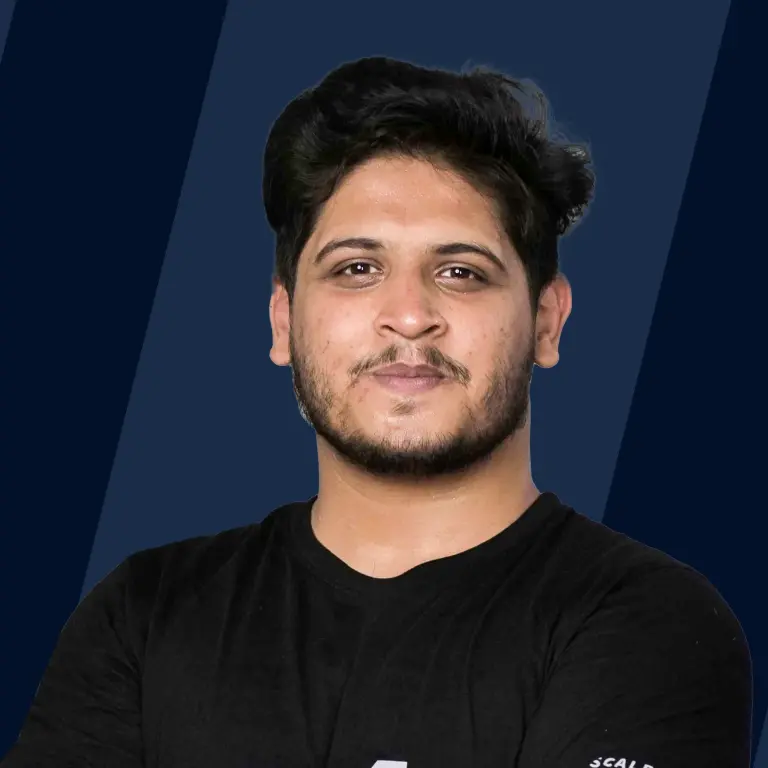
Overview
The javascript 2D array is a collection of the same type of elements that can be stored as a matrix using the various rows and columns. Each of the elements of the array is stored in these rows and columns using some specific index value. These values represent the position of an element in the matrix. The rows and columns are generally represented by i and j. Technically, a 2D array in javascript does not exist. But in javascript one or more than one 1-dimensional array is combined to form the javascript 2D array, known as a jagged array or ragged array.
Introduction to 2D Arrays in JavaScript
A javascript 2d array is a type of multidimensional array in which we can have multiple arrays and these arrays can store elements of the same data type. All the elements of an array are stored in the form of rows and columns. A 2d array in javascript stores its element in the heap memory. A javascript 2d array can be understood by dividing the concept into three parts.
-
Declaration of 2d array:
For declaring a 2d array, we use square brackets. First, we declare the data type of the array and then we use the square bracket. In a 2d array, there are two square brackets used for declaration. The first square bracket is known as the first dimensional or row of the javascript 2d array. And the second bracket is used for specifying the number of elements that we are going to store in that array. -
Initializing a 2d array:
Now we have declared the 2d array in javascript, but not initialized them It means the array is created but the values are not assigned to them. Currently, the array is containing only default values.For example, if we have declared the array of int type, then the default value is zero. Now we need to initialize them. For doing this, we need to use the loops or we can also initialize an array by using the array literals at the time of the creation of the array.
-
Reading a 2d array:
Now the array has been declared and initialized which means the array has its elements at their specific position. For reading these elements we need to know the index value of the elements. We use the loop and get the elements of a javascript 2d array. Generally, the rows are represented by i, and columns are represented by j. The indexing in javascript starts from 0. The first element is represented by index value 0, the second element by 1, and so on.
The Concept of Jagged Arrays
The jagged array is defined as an array of an array. In the jagged array, each element of an array functions as an array. The jagged array is a special kind of multi-dimensional array where there are several columns present in each of the given rows of the array.
The jagged arrays are also known as the Ragged arrays. Let us take an example where we create a 2-D array and there are two rows present in it. In each of the rows, there can be several columns present. The rows and columns in a jagged array need not be equal in number or length. The jagged array uses the heap memory concept to store its elements. Each of the elements of the array functions as a one-dimensional array.
Syntax:
The syntax of a jagged array is as follows:
To create a jagged array we need to declare the name of the array along with its data type. We use the keyword new each time to create a new object as shown in the example. After that, we declare the number of rows and columns in the array. Given below is an example of a jagged array.
True 2D Arrays vs Jagged Arrays
There are variations in the various programming language for using and declaring the multidimensional array. Programming languages such as C, C++, Fortan, etc can support the true 2-D array. But language like JavaScript uses the concept of a jagged array to imitate a multidimensional array. The multidimensional arrays use a different type of storage consumption. As we know, the true 2D array has m number of rows and n number of elements in each of the rows, a jagged array consists of any number of rows with any number of a column.
The number of rows and columns in a jagged array does not need to be the same. This makes it possible to consume less storage space and prevents wastage of space for the data set. Given below is the representation of a true 2D array:
And given below is the representation of the same data set in a jagged array:
How to Create 2D Arrays in JavaScript?
A two-dimensional array consists of elements that are stored in a matrix form. All the elements are organized in the form of rows and columns inside the matrix. When we create a 2D array, the rows, and columns are generally represented by the i and j. The 'i' and 'j' are used to represent the position of a particular element in an array. The i represents the row number and the j represents the column number. Suppose in a matrix, we need to get the element that is in the second row and at the third position in the columns, then the indexing of the matrix will be like i = 2 and j = 3. Now, let us see two different methods of creating the javascript 2D array.
Using Square Brackets
The square bracket is used to create a javascript 2D array. This method is used to create an array when we have a finite number of elements to store in the array. Given below is the syntax for creating a 2D array using the square bracket:
Given below is an example of creating a javascript 2D array using the square bracket according to the above syntax.
Output:
Explanation:
In the above example, first, we declare a variable namely chessBoard, and then we use the square bracket to create an array. Then we again create an array inside the array by using the square brackets again. Then it becomes a 2d array. And inside this array, we declare the elements that we need to keep inside the matrix.
Using new()constructor
The new() constructor method is used to create a javascript 2D array when we do not exactly know the elements before declaring the array. For creating a 2D array using the new() constructor method we need to use the loop for iteration. Then the javascript will initialize each element of the array with a value undefined.
Avoid the new() operator:
Using the new() operator is not always a good idea while creating a javascript 2d array. Because when we create a javascript 2d array using the new() operator, the method calls the interpreter to create an array constructor. This additional work increases the workload of the interpreter because the interpreter needs to search for the array constructor globally and then call it.
Given below is an example of the javascript 2D array using the new() constructor method.
Output:
Explanation:
In the above example, we first declared an array namely array_2D. Then we use the keyword new to create an object. Then we used the for loop for making the iteration until the condition was satisfied. Inside the for loop, we initialized the loop from i=0 until the value of i is less than or equal to 5. Then we used the console.log to print the output and assigned it the value of the variable namely array_2D. Hence the resultant output is 5 empty items whose value is undefined.
Operations on 2D Arrays in JavaScript
Till now, we have learned how to create a javascript 2d array. Now, let us discuss the various operations that can be performed on the 2d array. As we know a javascript 2d array consists of various elements stored in the form of rows and columns. We sometimes need to access, remove, insert, and update these elements. Here we will discuss all these operations. For this let us first create a javascript 2d array and provide them some values.
Output:
Access Elements
In a javascript 2d array, we use the square brackets to access the elements from the given array. As we know, javascript only supports the index system that is based on the integer type. If we want to use a string-based index, the variables are changed from an array to an object. this makes the array lose some of its properties. Given below is an example of how we can access the elements from a javascript 2d array.
Access First and Last Element
Accessing the first and the last element of a 2d array is very simple. For doing this, we just need to know the index value of that particular element. The index of the first element of a javascript 2d array will be . In the case of the last element, if we do not know the index value of the last element then we can use the length property of an array in javascript to access the last element. In javascript, the indexing starts from 0, so the index of the first element of the array will be 0 and the index of the last element of the array will be length-1. To learn more about the length property of an array in javascript, click here:
Output:
Looping through All the Elements
Using either a for loop or a forEach loop, we may iterate through every entry in the array. We already generated and initialized our array using a loop. Now let's use a forEach loop to attempt to calculate the sum of all the elements in our array.
Output:
Insert/Remove Elements – Push & Pop
Now we will discuss how we can insert a new element in the javascript 2d array. We use the push() method to insert a new element in the given 2d array. Let us see this using an example.
Output:
Explanation:
In the above example, we first created a javascript 2d array and initialized it to add 5 elements in the array. After that, we started a for loop to make the iteration in the program. We provided to continue the iteration until it is less than the length of the array. For each element, we used the increment operator to increase each element by 1. This will create a 2d array with 5 elements in it. Now we used the push() method to insert the new element in the array. And we also provided the index value length-1 where we need to insert the new element. In the push() method we passed the value 20 as the argument to be inserted at the given index.
Removing from the End of Array
The pop() method is used to remove the last element provided in the javascript 2d array. The pop() method also alters the length of an array after execution gets done. Suppose we have five elements in an array and we use the pop() to get an element. Then after the pop() method works, the new length of the array will be 4. Let us see an example.
Output:
Explanation:
In the above example, we first created a javascript 2d array and initialized it to add 5 elements in the array. After that, we started a for loop to make the iteration in the program. We provided to continue the iteration until it is less than the length of the array. For each element, we used the increment operator to increase each element by 1. This will create a 2d array with 5 elements in it. Then we used the pop() method to remove the element from the array. Again we removed the element from the index value length-1. And then we used the console.log to print the output which is the element that has been removed from the array.
Insert/Remove Elements – slice()
The slice() method is used to modify an array by adding, removing, or replacing an element from a 2d array. The returned array works as a new array object. The slice() method is used for slicing single or multiple elements from a string index value to the end index provided by the user in the method. And after getting access to that element, we can either add, remove or replace that element according to the user's choice. If we provide a negative index then the method returns that element from the ending of the array. The syntax of the slice() method is as follows:
Inserting at a Given Index
Now let us see how we can add a new element in the javascript 2d array. For adding the element, we need to specify the deleteCount property as 0 and provide this deleteCount to the slice() method. The element that is specified in the argument gets added to the given array. The new element gets added to the index that was provided in the parameter. Let us see this by using an example.
Output:
Explanation:
In the above example, we first created a javascript 2d array and initialized it to add 5 elements in the array. After that, we started a for loop to make the iteration in the program. We provided to continue the iteration until it is less than the length of the array. For each element, we used the increment operator to increase each element by 1. This will create a 2d array with 5 elements in it.
Removing from a Given Index
In the same way, we can also remove the elements from an array using the slice method. For doing this, we need to specify the index value in the deleteCounter parameter and then we need to specify the number of elements that we want to remove from the given array. If the deleteCounter is not specified by the user, then all the elements from start to end get removed. Given below is an example.
Output:
Explanation:
In the above example, we first created a javascript 2d array and initialized it to add 5 elements in the array. After that, we started a for loop to make the iteration in the program. We provided to continue the iteration until it is less than the length of the array. For each element, we used the increment operator to increase each element by 1. This will create a 2d array with 5 elements in it. After that, we used the slice method to remove the elements from the array. Inside the slice() method we passed the index value of the elements that need to be removed from the javascript 2d array.
Update Elements
As we can get access to any specified element in the javascript 2d array. We can also update or modify these elements in the given array. All we need to know is the index of that specific element that needs to be updated. Given below is an example.
Output:
Explanation:
In the above example, we first created a javascript 2d array and initialized it to add 5 elements in the array. After that, we started a for loop to make the iteration in the program. We provided to continue the iteration until it is less than the length of the array. For each element, we used the increment operator to increase each element by 1. This will create a 2d array with 5 elements in it. Then we used console.log to print the 2d array. After that, we again used a for loop to update the existing array. After that, we used the console.log to again print the new updated 2d array.
Conclusion
- The javascript 2D array is a collection of the same type of elements that can be stored as a matrix using the various rows and columns.
- The rows and the columns of an array are represented by i and j respectively.
- A javascript 2d array can be divided into three parts that are a declaration of the array, initialization of the array, and then reading or accessing that array.
- The jagged array is a special kind of multi-dimensional array where there are several columns present in each of the given rows of the array.
- There is various method of creating a javascript 2d array such as by using the new() operator, using the square bracket, etc.
- The square bracket method is used to create an array when we have a finite number of elements to store in the array.
- The new() constructor method is used to create a javascript 2D array when we do not exactly know the elements before declaring the array.
- Various operations that can be performed on a javascript 2d array are accessing the elements, accessing the first and the last element from an array, inserting elements in the array, updating the elements in the array, and removing the elements from the array.
- These methods are performed using theslice() method and pop() method.