Access Modifiers in Python
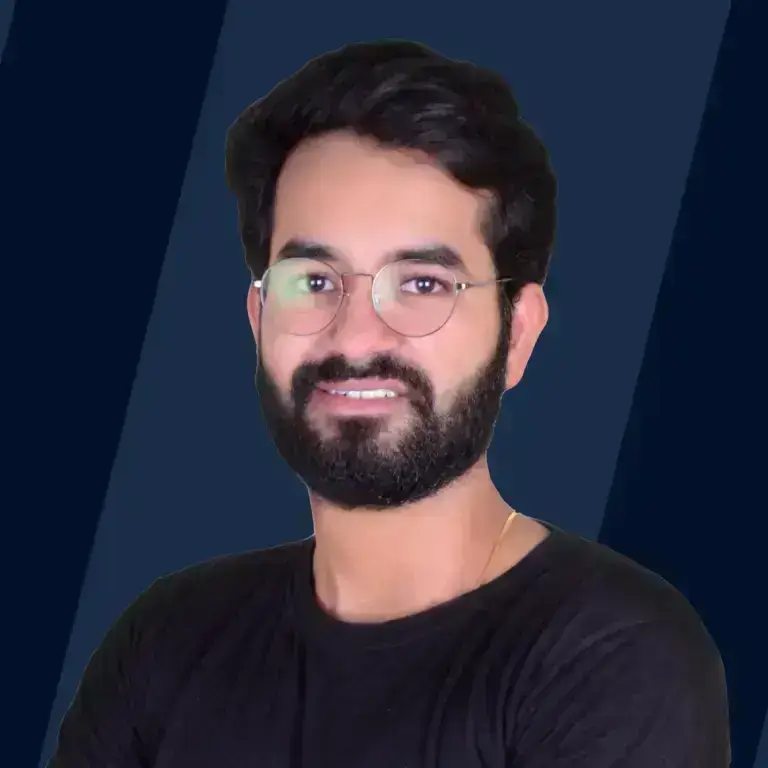
Overview
Access modifiers in Python control the visibility and accessibility of class members (attributes and methods) from outside the class. They determine whether other classes and objects can access, modify, or inherit a particular member. Python provides three types of access modifiers:
- Public Access Modifier
- Protected Access Modifier
- Private Access Modifier
Acess Modifier conventions:
- In Python, access modifiers are conventions, not strict enforcement.
- Developers can access protected and private members if necessary.
- However, directly accessing protected and private members is generally not considered good practice.
Inheritance and access modifiers:
Access modifiers like public, private, and protected control the visibility of class members in object-oriented programming. Public members are accessible anywhere, private ones only within the defining class, and protected members within the class and its subclasses. Protected members strike a balance, allowing subclasses to inherit and extend superclass behavior while maintaining encapsulation.
This promotes code reuse, flexibility, and maintainability, as changes in the superclass can propagate to subclasses. Inheritance and access modifiers work together to structure object-oriented code, ensuring controlled access to members for better code organization and security.
This article will explore these access modifiers in detail, along with their syntax and examples.
Prerequisites
Before diving into access modifiers in Python, you should have a basic understanding of the following concepts:
- Classes and objects in Python.
- How do you define class members (attributes and methods)?
- Inheritance in Python.
Public Access Modifier
The public access modifier allows class members to be accessible anywhere in the program. By default, all members in a Python class are public unless explicitly specified otherwise.
Syntax:
Example - 1
In this example, we create a class MyClass with a public attribute and a public method. We then create an object of the class and access both the attribute and the method.
Code:
Output:
Explanation of the code:
The code defines a class MyClass with a constructor that initializes a public attribute my_public_attribute and a public method my_public_method. We then create an object obj of this class and access the attribute and the method using the object.
Example - 2
In this example, we are creating a class Rectangle with public attributes and a method to calculate the area of the rectangle.
Code:
Output:
Explanation of the code:
This code defines a Rectangle class with public attributes length and width. We also have a public method, calculate_area, to compute the area of the rectangle. We then create an instance of the class, set its dimensions, and calculate the area.
Protected Access Modifier
The protected access modifier restricts the access of class members within the class and its subclasses. To denote a member as protected, you can prefix it conventionally with a single underscore (_).
Syntax:
Example - 1
In this example, we create a base class, MyBaseClass, with a protected attribute and a protected method. We then derive a class MyDerivedClass from the base class and access the protected members in the derived class.
Code:
Output:
Explanation of the code:
The code defines a base class MyBaseClass with a protected attribute _my_protected_attribute and a protected method _my_protected_method. We then create a derived class MyDerivedClass that inherits from MyBaseClass. In the access_protected_member method of MyDerivedClass, we access both the protected attribute and the protected method.
Example - 2
In this example, we are creating a base class Animal with a protected attribute and a derived class Dog that accesses and displays the protected attribute.
Code:
Output:
Explanation of the code:
This code defines an Animal base class with a protected attribute _name. We also define a protected method _make_sound. We then create a Dog class that inherits from Animal and overrides _make_sound to make the dog bark. We create an instance of Dog and access the protected attribute and method within the derived class.
Private Access Modifier
The private access modifier restricts the access of class members to within the class only. To denote a member as private, you can prefix it conventionally with double underscores (__).
Syntax:
Example - 1
In this example, we create a class MyClass with a private attribute and method. We attempt to access these private members from outside the class, resulting in AttributeError because private members are inaccessible outside the class.
Code:
Output:
Explanation of the code:
In the code, we define a class MyClass with a private attribute __my_private_attribute and a private method __my_private_method. We attempt to access these private members from outside the class, which results in AttributeError as private members are not accessible outside the class.
Example - 2
In this example, we create a Person class with private attributes and a private method to display personal information.
Code:
Output:
Explanation of the code:
This code defines a Person class with private attributes __name and __age. We also define a private method __display_info to display personal information. The introduce method is used to access and display a person's private information. We create an instance of the Person class and call introduce to display the private information.
Conclusion
- Public Access Modifier makes members accessible anywhere in the program without restrictions.
- Protected Access Modifier restricts access within the class and its subclasses, denoted by a single underscore.
- Private Access Modifier confines access to within the class only, indicated by a double underscore.
- Access modifiers are essential tools for managing the visibility and accessibility of class members in Python.
- Access modifiers help encapsulate and protect a class's internal state.