Adapter Class in Java
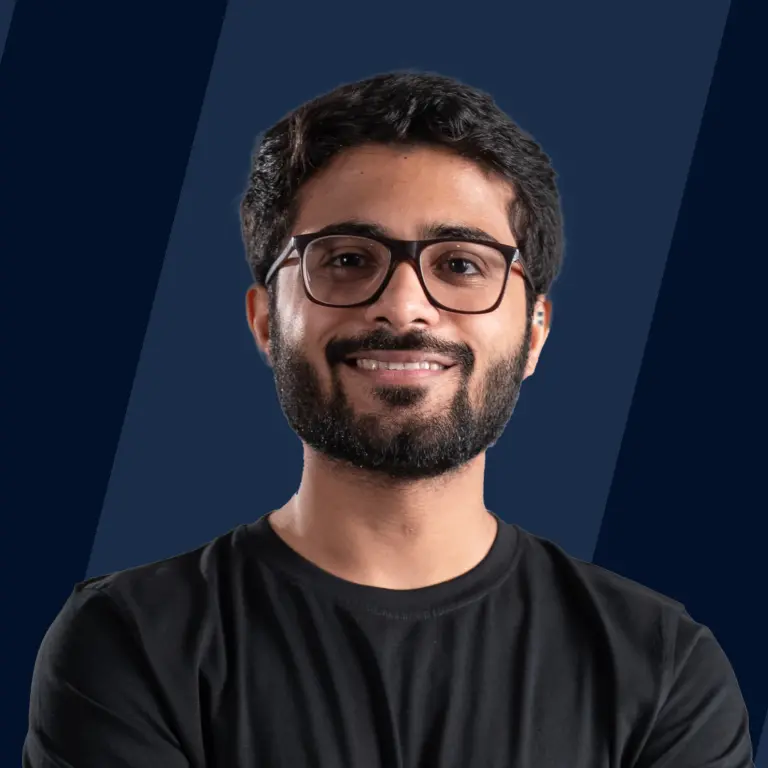
Overview
In this article, we are going to learn about the adapter class in java. Before getting started with the topic let us get a short overview of what is an adapter class in Java.
Adapter class in Java: Basically, an adapter class is a class in java, which is used to simplify the process of event handling. It usually provides an empty implementation of all the methods which are in the event listener interface. Without the adapter class, if we are implementing any event listener interface in our code for event handling, we need to define all the methods inside the interface manually, but with the help of the adapter class we do not need to implement manually, it will be automatically handled by the adapter class.
So let us now begin with the main agenda of our article, adapter class in java.
What Is an Adapter Class in Java?
In Java, for event handling, we use different interfaces. Still, for implementing the interface we must override all its methods manually by us, and sometimes it is a hectic process to do. Because let's say there are 5 methods in an interface, in that case, we need to override all the 5 methods of the interface, and if we only need one method, then, in that case, we override all the 5 methods and we define that method which we need. We left the rest of the methods blank. Which is not an ideal situation. So, for this purpose for the simplification of the event handling task, we use the adapter class in Java. In formal definition -
- An adapter class is a class in Java, which is used to simplify the process of event handling.
- It provides an empty implementation of all the methods which are in the event listener interface. Let us suppose if there is an interface MouseListener and if it has n methods. So, there will be an adapter class for this interface let us suppose MouseAdapter, and this adapter class will contain n empty methods of the MouseListener interface. So, if we extend this MouseAdapter class, we will not have to define all the methods of the interface, because it is already been defined by default in the adapter class.
- You just have to define a new class to act as an event listener by extending one of the adapter classes and implement only those methods which you want to use in your code.
Relationship Between Adapter Class in Java And Listener Interface
We use event listeners in our code when we intend to utilize most of the methods listed under the event listener interface. However, if we implement any listener interface directly in a class, then we have to implement all the methods within that interface, which makes the code unreasonably large. With the help of an adapter class, we can resolve this kind of complexity. An adapter class is an optimal way to implement an event listener when we are using only specific methods.
We just have to create a subclass for the adapter class and override the interest methods to use an adapter class. Adapter classes are, therefore, beneficial for listener interfaces in JAVA having more than one method. To better understand this, let us consider the example of the MouseListener interface. The main purpose of this interface is that it is notified whenever there is a change in the state of the mouse. It has five methods, mouse clicked, mouseExited, mouseEntered, mousePressed, and mouseReleased. When notified of an event, even if only of these methods is necessary, all of them need to be implemented. The methods that are not needed are kept empty. An adapter class is used to avoid this unnecessary implementation of an empty method body.
Types of Adapter Class in Java
Basically, there are three types of adapter class in Java, they are mentioned below :
- java.awt. event | Adapter Class in Java
- java.awt.dnd | Adapter Class in Java
- java.swing. event | Adapter Class in Java
Let us discuss them in detail.
java.awt. event | Adapter Class in Java
All the classes that are needed for event handling are included in this java.awt.event package. There is user interfaces in every event class that can be used for creating handlers for it. The entire Java interface is made up of abstract methods. If you are creating a class that is implementing an interface, you must implement all of its methods, whether you use it or not (the traditional practice is to provide the method with an empty block if you are not using it).
To resolve this problem, and to make programming easier, The classes that are included in the java.awt.event package already implement the interfaces in it, and also provide default overriding for their methods (usually keeping it blank). In the Java API, the event handling interface is termed the listner interface.
Basically, an event occurs when the object's state is changed. For example, dragging the mouse, clicking a button, and so forth. The java.awt.event package includes a number of event classes and Listener interfaces for event handling. They are shown below :
Adapter Class | Listener Interface |
---|---|
WindowAdapter | WindowListener |
KeyAdapter | KeyListener |
MouseAdapter | MouseListener |
MouseMotionAdapter | MouseMotionAdapter |
FocusAdapter | FocusListener |
ComponentAdapter | ComponentListener |
ContainerAdapter | ContainerListener |
HierarchyBoundsAdapter | HierarchyBoundsListener |
java.awt.dnd | Adapter Class in Java
In Java 1.2, there is a package called as the java.awt.dnd package. The java.awt.dnd package majorly consists of various interfaces and classes, which enables the usage of the drag and drop features, hence making data transfer possible. This drag-and-drop feature is built upon the data transfer framework of the java.awt.datatransfer package. The proxy for any object which needs to receive the drops is the DropTarget object. Similarly, the DragSource object is also a proxy that is responsible for the drag. The java.awt.dnd package also provides multiple event classes which are responsible for handling all the communications occuring within inter-objects, throughout the drag operation.
Adapter Class | Listener Interface |
---|---|
DragSourceAdapter | DragSourceListener |
DragTargetAdapter | DragTargetListener |
java.swing.event | Adapter Class in Java
The javax.swing.event package is an extension of the java.awt.event package. The javax.swing.event package provides us the different Swing-specific event objects, listeners, and adapters. The event types are basically defined through the names of the classes ending with "Event". The methods and the fields of the classes provide us the information about the occurrence. Whereas, the interfaces whose name ends with "Listener" are the event listeners.
Whenever any particular event occurs, the above-mentioned interface methods alert the interested objects. A listener implementation that ends with the word "Adapter" provides a useful no-op implementation. It is way more complicated to create an equivalent listener interface than by subclassing an adapter class.
Adapter Class | Listener Interface |
---|---|
MouseInputAdapter | MouseInputListener |
InternalFrameAdapter | InternalFrameListener |
Examples of Adapter Class in Java
Now, let us look at some examples of adapter classes in Java, for better understanding.
Window Adapter Class in Java
In the following example, we are implementing the WindowAdapter class of AWT. Basically, Adapter classes in Java that receive window events are called WindowsAdapter. Here, by "window events", we mean events that indicate that a window has changed its status. These events are generated usually when the window is opened, closed, activated, deactivated, iconified, or deiconified, or when the focus is transferred into or out of the Window. The methods of this class are all empty. For constructing listener objects this utility class is used. We are also using one of its methods windowClosing() to close the frame window.
Code:
Output:
Explanation: Let us break down the above code and understand it in detail. In the above code,
- Firstly, we are importing all the necessary libraries.
- After that, we are creating objects of frame, label, and panel.
- Inside the AwtAdapterDemo class we have created its constructor, which is called the prepareGUI() method.
- Inside the main method we are creating the AwtAdapterDemo class object instance, and we are calling it the showWindowAdapterDemo() method.
- Inside the prepareGUI() method we are creating a frame (container that consist of title bar and border and can have menu bars) with the title "Java AWT Examples" and then we are adding the WindowListener to the frame
- Since, we are extending the WindowAdapter class from the java.awt.event library, we are not required to override all the methods inside the WindowListener interface, and we are just overriding the windowClosing() method.
- After that, we are labeling the window by setting its alignment, and size.
- Inside the showWindowAdapterDemo() we are creating another frame with the title "WindowAdapter Demo", and here also we are adding the WindowListener to the frame and overriding the windowClosing() method.
- Finally, we are labeling the window by setting its alignment, and size.
Key Adapter Class in Java
Key Adapter is an adapter class in Java. One of the major functions of the Key Adapter is to receive keyboard events. It consists of all empty methods. It is a utility class for building listener objects. In the following example, we are implementing the Key Adapter class and its methods.
Code:
Output:
Explanation: Let us break down the above code and understand it in detail. In the above code,
- Firstly, we are importing all the necessary libraries.
- After that, we are creating objects of frame, label, and panel.
- Inside the KeyAdapterDemo class we have created its constructor, which is called the prepareGUI() method.
- Inside the main method we are creating the KeyAdapterDemo class object instance, and we are calling it the showKeyAdapterDemo() method.
- Inside the prepareGUI() method we are creating a frame with the title "Java AWT Examples" and then we are adding the WindowListener to the frame
- Since, we are extending the WindowAdapter class from the java.awt.event library, we are not required to override all the methods inside the WindowListener interface, and we are just overriding the windowClosing() method.
- After that, we are labeling the window by setting its alignment, and size.
- Now, inside the showKeyAdapterDemo() we are adding a text field, and here we are adding the KeyListener to the frame and overriding the keyPressed() method.
- We are also adding a button,
- Once we type the text, in the text section, click the button, the keypress() method is triggered, and our entered text is displayed below.
Mouse Adapter Class in Java
Mouse Adapter is an adapter class in Java. One of the major functions of the Mouse Adapter is to receive mouse events. It consists of all empty methods. It is a utility class for building listener objects. In the following example, we are implementing the Mouse Adapter class and its methods.
Code:
Output:
Explanation:
Let us break down the above code and understand it in detail. In the above code,
- Firstly, we are importing all the necessary libraries.
- After that, we are creating objects of frame, label, and panel.
- Inside the MouseAdapterDemo class we have created its constructor, which is called the prepareGUI() method.
- Inside the main method we are creating the MouseAdapterDemo class object instance, and we are calling it's showMouseAdapterDemo() method.
- Inside the prepareGUI() method we are creating a frame with the title "Java AWT Examples" and then we are adding the WindowListener to the frame
- Since, we are extending the WindowAdapter class from the java.awt.event library, we are not required to override all the methods inside the WindowListener interface, and we are just overriding the windowClosing() method.
- After that, we are labeling the window by setting its alignment, and size.
- Now, inside the showMouseAdapterDemo we are adding the mouseListener to the frame and overriding the mouseClicked() method.
- Once the mouse is clicked, the mouseClicked() method is invoked, and the number of times the left and the right side of the mouse are clicked is displayed below.
Difference Between Adapter Class in Java And Object Adapter
Class Adapter
- The class adapters use multiple inheritances to carry out their aims.
- Just like the object adapter, the class adapter also inherits the client's target interface.
- However, it inherits the adapter's interface as well.
- Due to the fact that Java does not allow real multiple inheritances, the java interface class must acquire a few of the interfaces.
- It is an important point to note that the Java Interface might be the adapter interfaces or the target.
- The target request is simply redirected to the particular request inherited from the adapter interface.
Object Adapter
- The Object adapters usually convert one of the interfaces to another with the help of the compositional method.
- The adapter object gets the target interface, which the user is expecting to see while holding the instance of the adapter.
- When the client performs the request() function on one of its particular objects (the adapter), the request is converted into the matching individual demand of the adoptee.
- The user and the adapter are totally disconnected by the object Adapter anyhow. However, the adapter only knows about their presence.
Advantages of Adapter Class in Java
The first and foremost benefit of the adapter class in Java is that it allows us the different unrelated classes to function (or work) together and hence allows the usage of the classes in various ways. Also, the Adapter classes help to combine the related patterns, improving class transparency.
Apart from that, the users also get the option to use a pluggable kit for developing the apps. All these make the Adapter classes become extremely reusable and important.
Let us jolt down into point some of the major benefits that the Adapter class in Java provides --
- Even the distinct or unrelated classes can work (function) together using the Adapter class.
- Similar classes can be utilized in multiple ways with the help of the Adapter class in Java
- It helps in improving the visibility of the classes.
- It makes it simpler to work with disconnected classes.
- It enhances the reusability of the classes in multi-folds.
- Also, the users get an option to use the pluggable kit to create the apps, hence becoming very useful.
- Adapter class also helps in grouping the patterns together in a class.
Frequently Asked Questions of Adapter Class in Java
1. What Is an Adapter Class in Java?
Answer: The adapter pattern which is used to define a class is called an adapter class. Without changing an existing class, an adapter can be used to extend the functionality of that class. The adapter pattern is used to expand the functionality of an existing class without having to change its code.
2. What Are Adapter Classes Used For?
Answer: The adapter class is used for providing the basic implementation of all methods in an event listener interface. When you want to define very few methods of event handler interface, using an adapter class you don't have to override all the methods of the interface, and in this case adapter class comes in handy.
3. What Are the Advantages of Using the Adapter Class in Java?
Answer: The main advantage of using the adapter class is that you do not have to override all the methods of any interface, which is not required in your code for event handling. As the adapter class defines all the methods of the interface (keeping them blank). Which will reduce the length of the code, and also it is an ideal practice.
4. How Many Adapter Class Are Used in Java?
Answer : The Java adapter classes can be found in three different packages. The three packages are- java.awt.event, java.awt.dnd, and javax.swing.event.
5. What is an AWT in Java
Answer : Java AWT (Abstract Window Toolkit) is a Java API, which is used for creating a graphical user interface (GUI) or any window-based applications. In accordance with the operating system's perspective, platform-dependent Java AWT elements are shown.
6. What Is an Adapter Design Pattern in Java?
Answer : In software design, a design pattern is a common, useful solution to a frequent problem in a specific environment. It is not the ultimate layout that can be turned into code. It is kind of a description or template for solving an issue that may be applied to a variety of scenarios. There are various design patterns in Java to choose from. There is no need to derive a design pattern from a different language.
7. What Is the Difference Between the Adapter Class and The Listener Interface?
Answer : For a given interface, all the methods do not need to be implemented in an adapter class. An adapter class is required when only a few of the methods of an interface needs to be changed. Without the adapter class, we have to override all of the methods defined in a listener’s interface.
8. What Are Adapter and Wrapper Classes in Java?
Answer : A wrapper class is the one, which is used in the Facade pattern, which is designed to make the in traction with external libraries easier. The main purpose of an adapter is to fill the gap between two interfaces. You may look at a new library you want to utilize and develop a wrapper to make it easier to use.
9. What Is an Object Adapter?
Answer : An object's principal is the primary means for an object to access ORB services like object reference generation through an object adapter. A portable object adapter exports standard interfaces to the object. An object adapter’s major tasks are to generate and understand object references.
10. What Is the Difference Between a Class Adapter and An Object Adapter?
Answer : Class Adapter uses inheritance and can only wrap a class. It cannot wrap an interface since by definition it must derive from some base class. Object Adapter uses composition and can wrap classes or interfaces, or both. It can do this since it contains, as a private, encapsulated member, the class or interface object instance it wraps.
Conclusion
In this article, we learned about the adapter class in java. Let us recap the points we discussed throughout the article:
- an adapter class is a class in java, which is used to simplify the process of event handling.
- Without the adapter class, if we are implementing any event listener interface in our code for event handling, we need to define all the methods inside the interface manually, but with the help of the adapter class we do not need to implement manually, it will be automatically Handel by the adapter class.
- You just have to define a new class to act as an event listener by extending one of the adapter classes and implement only those methods which you want to use in your code.
- there are three types of adapter class in Java, they are - java.awt.event, java.awt.dnd, javax.swing.event.
- All the classes that are needed for event handling are included in this java.awt.event package.
- The java.awt.event package includes a number of event classes and Listener interfaces for event handling. They are - WindowAdapter - WindowListener, KeyAdapter - KeyListener, MouseAdapter - MouseListener, MouseMotionAdapter - MouseMotionAdapter, etc.
- In Java 1.2, there is a package called as java.awt.dnd package. The java.awt.dnd package majorly consists of various interfaces and classes, which enables the usage of the drag and drop feature, hence making data transfer possible.
- The java.awt.dnd package also provides multiple event classes which are responsible for handling all the communications occurring within inter-objects, throughout the drag operation. Some of its adapter classes and listener interface are DragSourceAdapter - DragSourceListener, DragTargetAdapter - DragTargetListener.
- The javax.swing.event package is an extension of the java.awt.event package. The javax.swing.event package provides us the different Swing-specific event objects, listeners, and adapters.
- Some of the adapter class and listner interface of javax.swing.event package are - MouseInputAdapter - MouseInputListener, InternalFrameAdapter - InternalFrameListener.
- We have seen some examples of window adapters, key adapters, and mouse adapter class in Java.
- We have seen the difference between the adapter class and object adapter in Java.
- The benefit of the adapter class in Java is that it allows us the different unrelated classes to function (or work) together and hence allows the usage of the classes in various ways. Also, the Adapter classes help to combine the related patterns, improving class transparency.