How to Add Two Numbers in Java
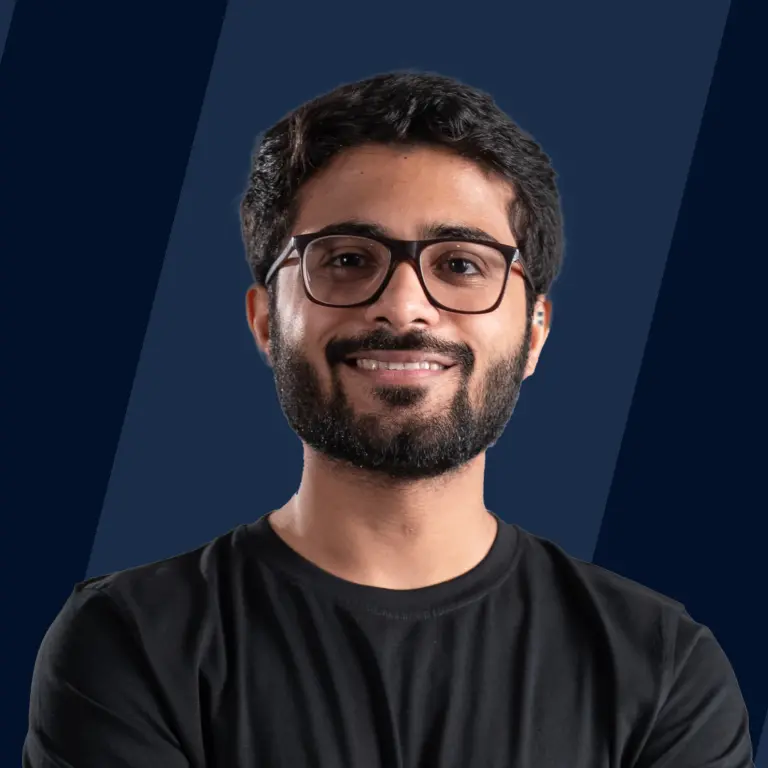
In this section, Java programs for calculating the sum of two numbers, three numbers, and n numbers are demonstrated. The first Java program, a straightforward example, prompts users to input two integers, stores them in variables, and displays their sum. Utilizing a user-defined function, the second program replicates this process for summing two integers. These programs serve as practical illustrations for understanding the basics of Java programming and user input processing.
Method 1: Sum of two numbers
This is the most easiest way to find the sum of two numbers in Java. We will initialise and declare the value in the program itself. Here the input is not taken from the user.
Example
Output
Explanation In the above program we have declared two variables and initialised their values in the program. The sum of the numbers is stored and displayed on the console.
Method 2: Add Two Numbers in Java With User Input
In Java, the Scanner class is one of the classes which fetches the user input.
Example
Output
Explanation We have initialised the scanner class so that we can take in the user's input. The line scn.nextInt() reads the user input in the form of integer and it gets saved in that variable. The sum gets stored and is displayed on the screen.
Method 3: Sum of Two Numbers Using Command Line Arguments in Java
Command line arguments are basically the arguments that are passed on the console during the program execution. It is the information that we pass after typing the name of the program during the execution and it is stored as strings in a string array. It is stored in String[] args. The arguments are in string and then we have to convert it into integer.
Example
Steps to be executed
- While compiling the above program in your IDE, type "javac Main.java" as the class here is Main. To execute the program, type "java Main 99 83". Here 99 and 83 get stored in the String[] args array.
- The first number at 0th index is stored in a variable and the same is done for the second number.
Output
Method 4: Sum of Two Numbers in Java Using Method
a) By using User-defined method
In this method we are creating a function where the addition of two numbers will be calculated and the value will be returned.
Example
Output
Explanation We have created a function sumFunction which is a user-defined function which takes in two numbers as parameters. The sum of these numbers is returned and displayed.
b) By Using the Sum() Method
The sum() method is used to add numbers given in the arguments of the method. The sum() method is in the Integer class of Java which is in the util package.
Syntax
Return type
Example
We will use the Integer.sum() function.
Output
Explanation In the above program we have declared two variables and initialised their values in the program. The sum of the numbers is calculated by the sum() method in Java which takes in numbers as arguments.
Method 5: Sum of 3 Numbers in Java
The sum of 3 numbers is similar to the sum of 2 numbers, the only difference is that here we have got three variables.
Example
Output
Explanation We have calculated the sum of 3 numbers.
Method 6: Sum of N Numbers in Java
This is a more generalised way of calculating the sum of a given numbers. When the number of iterations is unknown, we use loops to perform the operations in our program.
Steps-
- Read or initialize the value of N which is the number of integers to be added.
- The loop will run N times and ask the user to enter the numbers or else it depends on how the loop is initialised.
- Calculate the sum for each input and store it into a variable.
- When all the iterations are done, print the value of sum.
The flowchart below demonstrates the logic for the program of finding the sum of N numbers.
Example
Output
Explanation In the example, we have calculated the sum of N numbers with N taken as an input from the user. The loop runs N times and each time user enters a number to be added. The value of that entered number gets added in the sum variable and after the iterations are completed, it is displayed.
Integer Overflow
If our sum exceeds the maximum value for an integer in Java which is Integer.MAX_VALUE = 2147483647, it will cause integer overflow. If the sum is greater than Integer.MAX_VALUE which is the maximum value an integer can hold, then at the point when it exceeds that value, it gets converted to Integer.MIN_VALUE and the rest of the number is added. It goes to -2147483648 and then continues from there.
To avoid the integer overflow, use data type like long which can hold longer values.
Consider the example where we have stored the value 2147483647 which is the maximum value an integer can hold and then performed addition.
Example
Output
Explanation
- In the first sum, after adding 0 to it the value remains the same(2147483647) and hence it gets printed as it is.
- In the second sum, after adding 1, it exceeds the maximum value of an integer, hence it gets converted to Integer.MIN_VALUE and continues from there.
- In the third sum, after adding 2, it exceeds the maximum value of an integer when 1 gets added, hence it gets converted to Integer.MIN_VALUE and continues from there. The remaining number is then added to the value.
Conclusion
- Sum deals with the addition of given numbers.
- In Java we can find the sum of two numbers by various ways like- user input, command line arguments, user-defined and built-in method and by loops.