Python Program to Add Two Numbers
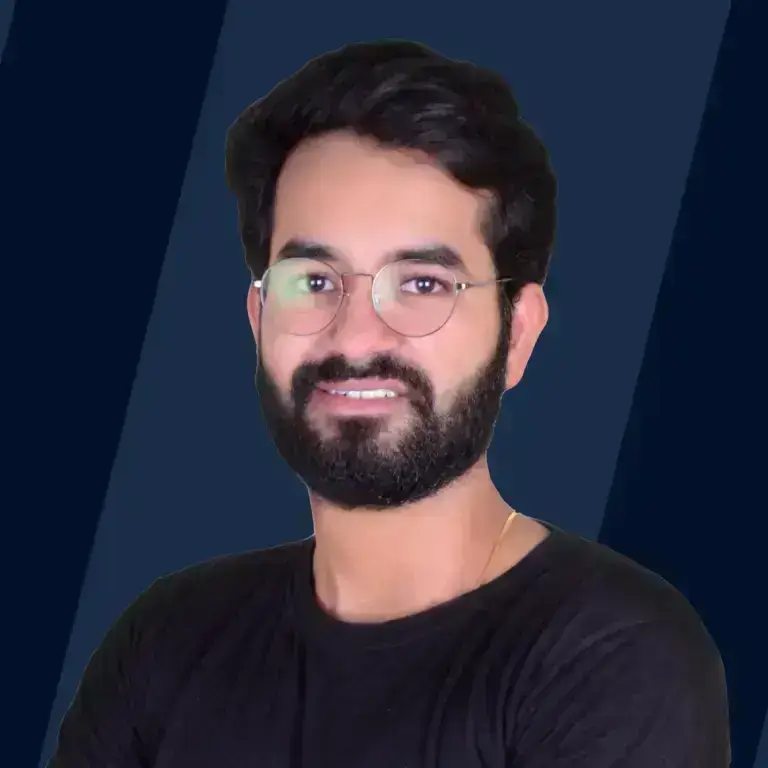
In Python, performing addition involves calculating the sum of two numbers, a process that is foundational to programming arithmetic.
This operation can be applied to various types of numbers, including integers, floating-point numbers, and complex numbers, showcasing Python's versatility in handling different numerical data types.
For example, to add two integers in Python, you can simply use the + operator as follows:
This will output:
Similarly, you can add floating-point numbers or complex numbers using the same + operator, demonstrating Python's straightforward approach to arithmetic operations across different data types.
Add Two Numbers in Python Using “+” Operator
The following syntax must be followed each time to add two numbers in Python.
Explanation:
In the syntax, the ‘+’ operator is responsible for computing the addition and returning the result. Post the calculation, the result needs to be stored in a variable so that we can print it. For this, we have used the variable called ‘sum’.
Example:
Instead of directly adding, we can store the value of our addition in a variable just like “sum” for later use.
Code:
Output:
If you run these codes, you will get the outputs 126 and 100, respectively, as shown.
Here, we will be adding two numbers that are of integer data type, that is, int + int. When we add two integers, we get the output as an integer.
Code:
Output:
Explanation:
- In the first two lines of code, we have taken two variables, a and b, and predefined their values as 1 and 6, respectively.
- Secondly, we have printed the datatypes of a and b using the type() function. In this case, both are integers.
- Next, we are adding the values stored in variables a and b using the ‘+’ operator and storing the result of the addition in a new variable called ‘sum’.
- Finally, we print our result, the ‘sum’ variable and its data type. The variables a and b are both of the same data type, int. Therefore, the sum's result also belongs to the int data type.
Adding Two Numbers With User Input in Python
Instead of hardcoding the input values every single time, we can even ask the user to enter the input values.
Code:
Output:
Explanation:
- In the program shown above, we asked the user to enter num 1 and num 2. To take the input, we used the built-in function: input().
- Since, input() function returns a string, we used the float() function to convert the string to a number.
- The numbers are then added together and stored in variable ‘sum’ which is printed to get the resultant addition of two numbers.
Defining add function and returning the result
We can create a function add which will take two arguments and return the resultant sum as return value from the function. the below program demonstrate the working of addition of two numbers in python using function.
Output
Addition of Two number in Python operatior.add() Function
The Operator library provides different methods to perform mathematical computations. The operator.add() method in Python is used for addition. Here's an example of how you can use it to add two numbers:
Code:
Output
Adding two numbers using the lambda function
Lambda functions are in-hand shortcut methods to implement the statements. You can use a lambda function to add two numbers in Python as well. Here's an example:
Code:
Output
Sddition of two numbers in Python with a Recursive function
We can use a recursive approach to add two numbers in Python. The basic idea of implementation is to recursively increment one operand and decrement another one. Here's an example:
Code:
Output
Conclusion
- The addition of two numbers in python is one of those programs that cross the path of every beginner.
- Python numeric data types, combinations among them for addition, storing the summation in a variable, and printing the result, get clarified in the process.
- In this article, we have learned the syntax that needs to be followed for adding two numbers in Python.
- We have walked past the addition of int and float using implicit and explicit type conversion.