Advanced Concepts in JavaScript You Need to Know
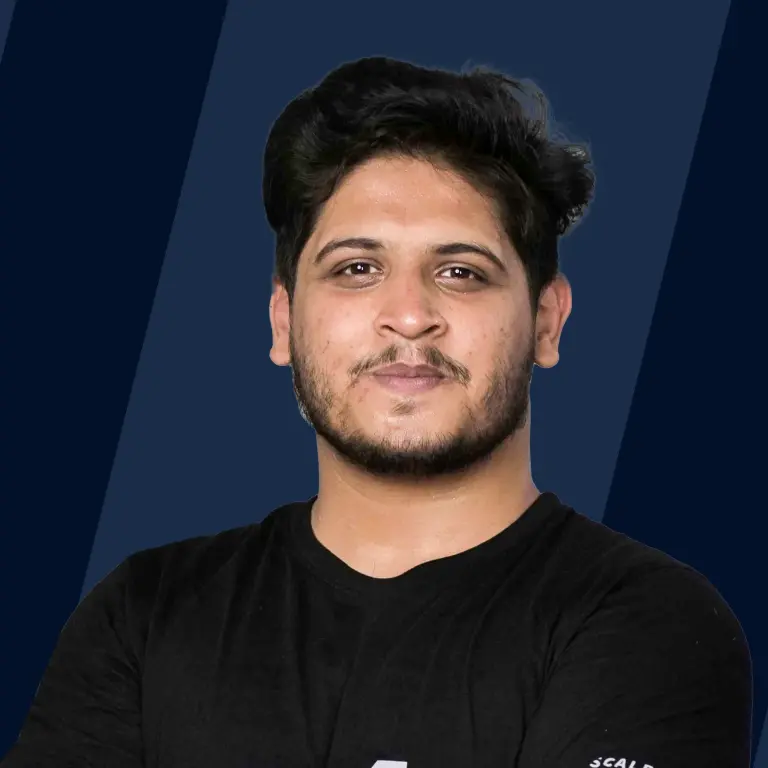
JavaScript is a programming language that is highly used in modern web development for making interactive web pages and also has multiple applications including server-side development with Node.js, mobile app development with React Native, and cross-platform desktop applications with Electron. Let us explore some of the most important and powerful concepts that can elevate your JavaScript skills.
Truth and False Values in JavaScript
In JavaScript, every value is either true or false when evaluated in a boolean context. JavaScript considers certain values as truthy, meaning they are treated as true, while others are falsy, treated as false.
Truthy Values:
Truthy values are those that are evaluated as true in a boolean context.
Output:
In this example, the variable value holds a non-empty string. The if statement evaluates the non-empty string in value as true.
Falsy Values:
False values are those that are evaluated as false in a boolean context.
learn more about various falsy values in javascript and their behaviors.
Output:
In this example, the variable value is an empty string. The if statement considers the empty string in value as false.
new Function
The new Function constructor in advance javascript provides a dynamic way to create functions during runtime, where the function's logic is determined dynamically.
Example
In this example, we utilize the new Function constructor to create a function that takes two parameters and returns their sum. You can also explore a new way to perform the same action in the article on javascript constructors.
Arrow Functions
Arrow functions are part of advanced javascript and offer a more expressive syntax for writing functions. They provide a shorthand way to define anonymous functions and often reduce the need for explicit binding of the this keyword.
Example
The arrow function ArrowFunction has a more concise syntax. The function can be split into:
- The parameters a and b
- The =>, if followed by {} should have a return statement, and single expressions are directly returned.
- The expression.
Explore more on arrow functions
Rest Parameters & Spread Operator
Rest Parameters
Rest parameters in advance javascript allows a function to accept an arbitrary number of arguments as an array. This is denoted by the use of three dots (...) followed by the parameter name.
Example:
In this example, the calculateSum function uses the rest parameter, ...numbers to accept any number of arguments passed to it. The rest parameter gathers these arguments into an array called numbers. The reduce method is then used to sum up the values in the array. If you are confused about the reduce method, you can read about it in reduce in JavaScript.
Spread Operator:
The spread operator is also a part of advanced javascript, also represented by three dots (...), and performs the opposite action. It allows an array or iterable to be spread into individual elements.
Example:
Here, the spread operator is used to concatenate two arrays which will create a new array with all elements.
Async/Await vs Generators
Both Async/Await and Generators are mechanisms introduced to handle asynchronous operations in advance javascript.
Async/Await
Async/Await is used for dealing with asynchronous code. It allows developers to write asynchronously with better maintainability. Learn more about async/await in javascript.
Generators
Generators are a lower-level approach to handling asynchronous code. They allow functions to be paused and resumed, providing a manual way to control the flow of asynchronous operations. Learn more about Javascript generators
Promises
Promises are used to handle asynchronous operations more effectively in advance javascript. The Promise constructor is used to create a Promise and it takes a callback function with parameters such as reject and resolve. When the operation completes successfully, the resolve function is called with the result. If an error occurs, the reject function is called with an error message.
Example:
In this example, the fetchData function returns a Promise. Inside the callback, an asynchronous operation is simulated using setTimeout. After a delay of 2000 milliseconds, the Promise either resolves with the fetched data or rejects with an error message.
Prototypes in JavaScript
In JavaScript, every object has a prototype, which acts as a template for the object's properties and methods. Prototypes enable the concept of inheritance in JavaScript, allowing objects to share and override properties and methods.
Example
In this example, the animalPrototype object acts as a prototype for other objects to create new objects. These objects inherit the properties and methods from their prototype. Learn more about inheritance in javascript.
Chaining JavaScript Methods
Chaining methods in JavaScript is a technique used for calling multiple methods on an object in a single statement.
Example
In this example, an array of numbers is manipulated using the following methods:
- The filter method filters the numbers greater than 2.
- The map method is then applied to double each remaining number in the filtered array.
- The reduce method calculates the sum of the modified numbers.
In this operation, each method in the chain operates on the result of the previous one. You can also learn more on call method in javascript for chaining.
Global Object in JavaScript
The global object in JavaScript represents the global environment and serves as the container for various global properties and functions available in both browser and Node.js environments. In a web browser, the global object is usually the window object.
Example:
In a browser, the window object is the global object. Properties like window.innerHeight provide information about the browser window, while window.location.href accesses the current URL. Any variables created become a property of the global object. Learn more about global object
Function Binding
Function binding involves explicitly setting the value of this within a function. It allows you to control the context in which a function is executed.
Example
By default, the this within the greet method refers to the user object. However, we use the bind method, thethis is explicitly set to a different context. Now, when we invoke the greetFunction, it prints the message based on the new context.
Closures
Closures refer to the ability of a function to remember and access variables from another scope even after that scope has finished executing.
Example:
In this example, we can access the inner function only through the outer function. When closure1 is created by invoking the outer function, it closes over the value 5 for outerVariable. When we later invoke closure1(3), it prints the sum of the closed-over value (5) and the parameter passed to innerFunction (3). Learn more about closures in javascript
Lexical Environment
A lexical environment in advance javascript is the context in which variables and functions are declared and exist during runtime.
Example
In this example, the createCounter function defines a lexical environment. It has a local variable and an inner function that accesses and modifies the count variable. When counter1() is called, it increments and prints the value of the count variable within its captured lexical environment. Meanwhile, when counter2() is called, it operates independently, maintaining a separate lexical environment and its own count variable. Learn more about scope in Javascript.
Execution Context
The execution context represents the environment in which code is executed. It includes information about the code being executed, variable scope, the value of the this keyword, and other details necessary for the runtime execution of the code. The execution context plays a major role in Hoisting. Learn more about Execution Context in javascript.
FAQs
Q. What is the significance of arrow functions in JavaScript?
A. Arrow functions in JavaScript provide a concise syntax and lexical scoping. They also have implications for the value of 'this' within the function.
Q. How do rest parameters and the spread operator enhance function flexibility?
A. Rest parameters allow functions to accept any number of arguments, while the spread operator expands the arrays or iterable objects into individual elements.
Q. Why is understanding prototypes important in JavaScript?
A. Prototypes help in understanding JavaScript's object-oriented nature and enable efficient code organization, reuse, and inheritance.
Conclusion
- JavaScript with applications in web, mobile, and desktop development encompasses advanced concepts like arrow functions, rest parameters, and async/await, providing developers with powerful tools for efficient and readable code.
- Promises play a crucial role in handling asynchronous operations, offering a clean and structured approach with simplified error handling and chaining capabilities. Generators help in controlling the flow of asynchronous operations and async/await syntax helps to work with asynchronous operations easily.
- Function binding and prototypes contribute to effective code organization and inheritance, providing developers with control over function execution contexts and object behaviors. Prototypes help to understand objects and are used for inheritance.
- Method chaining allows the sequential execution of operations on objects, reducing the need for intermediary variables. Understanding closures and lexical environments enhances the developer's ability to manage variable scope and create modular and encapsulated code structures.