Top Advance Python Concepts for Python Developer
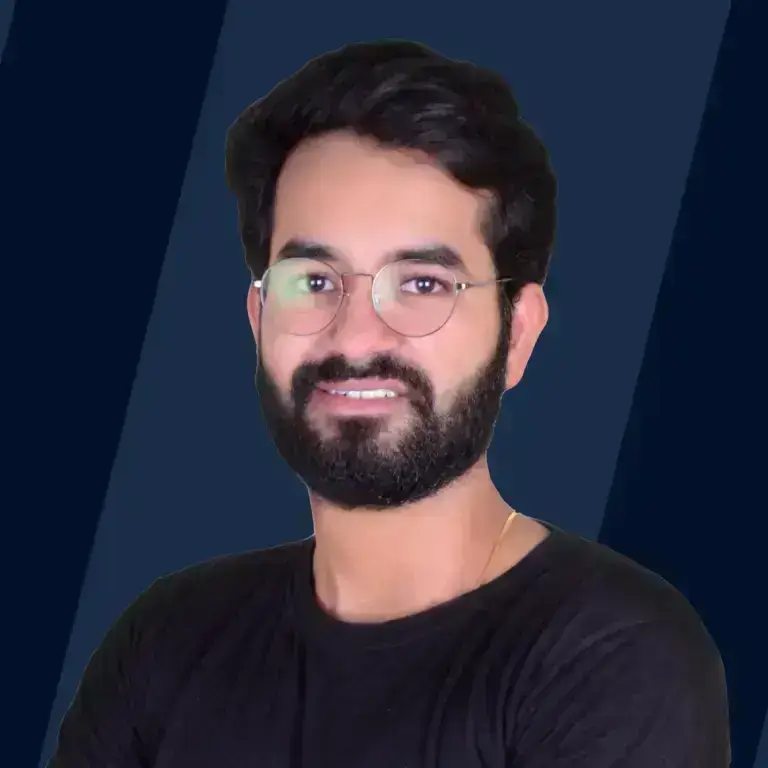
Overview
Python is a powerful and object-oriented programming language that is famous for its clarity and simplicity features. Multiple advanced concepts are supported by Python. A wide range of opportunities are provided by Python for both freshers and experienced programmers. In this article, we are going to study the advanced Python concepts, which would be very helpful in becoming a Python expert.
Top Advanced Python Concepts That You Must Know
Python is an object-oriented and high-level programming language that is nowadays becoming the best programming language for a lot of professionals and students because of its easy-to-learn, dynamic, and versatility features. Python can be used in multiple domains like artificial intelligence, testing, automation, data analytics, web development, data science, machine learning, technical fields, etc.
Map Function
map() function in python is an inbuilt function of Python, by which we can perform operations or process the data of the iterable without using the loop features.
The map object is returned by the map() function which also works as a iterator. A map object returned by the map() function contains all the elements of the iterable after applying the operation specified in the function.
Syntax:
The map() function has the following arguments:
The first argument specifies the function name which tells the operation to be applied to all the elements of the iterable. The second argument specifies the iterable on which the specified function is mapped.
In a real-world scenario, the map function in Python is commonly used to apply a specific operation or transformation to each element in an iterable (such as a list) and generate a new iterable with the results. This can be particularly useful for simplifying code and improving readability.
Example:
Output:
Itertools and Advanced Data Structures (Including Collections, Namedtuples, and Dataclasses)
Itertools is a standard library provided by Python that involves various functions that support us in making code fast, clean, and memory-efficient because of lazy evaluation. Multiple building blocks of the iterator are implemented by the itertools module and they all combine to make the iterator algebra which helps us in efficient tool building. The functions provided by the itertools module operate in the iterators and return more complex iterators. Itertools provide multiple functions such as combinations(, permutations(), product(), accumulate(), repeat(), cycle(), count(), etc. Every function has its arguments and performs operations on them. Functions provided by itertools generate results very fast in comparison to generating results from the conventional code.
Data structures help us in organizing and maintaining the data efficiently. Python provides in-built data structures such as Collections, NamedTuples, Dataclasses, etc.
- Multiple containers are provided by the python collection Module. The container allows us to store a variety of objects and we can access all those objects by iterating over them. Some examples of built-in containers are dictionaries, lists, tuples, etc.
- Collections modules provide NamedTuple is present.
- DataClasses is a class that mainly consists of the data, but there are not any restrictions.
Lambda Functions
A lambda function is also an advanced Python concept, it is a small anonymous function that does not have any name and is written within one line. In Python, functions are defined using the def, but for defining lambda functions we use the lambda keyword. The lambda function can have n number of arguments but it has only one expression. It helps us make code concise and increase the code readability and is considered as the best option when we have to use the function only once.
Syntax:
Lambda functions are concise and serve well in situations where a short, one-time-use function is needed, such as in this sorting operation.
Example:
Output:
Exception Handling
Exceptions are the errors that occur during program execution and disturb the normal program flow. Some of the examples of exceptions are trying to divide any number by zero, or trying to access the element from an index of iterable which is greater than the size of an iterable. Python provides try, except, and finally keywords for exception handling. The Try block is used for writing the block of code in which there is a chance of errors, the except block is used to write the code that will be executed when the exception occurs in the try block code, and finally block always executes in both cases when an exception is raised or when an exception is not raised.
Exception handling is crucial in scenarios where your code may encounter unexpected issues or errors. A real-world use case for exception handling is in file handling operations.
Decorators
In Python, decorators are a powerful way to modify or extend the behavior of functions or methods without changing their code. They use the "@" symbol and are applied above the function definition.
A real-world use case for decorators in Python is in web frameworks, where decorators are commonly used to modify the behavior of functions that handle HTTP requests.
Generators, Iterators, and Comprehensions
Generators are the special function provided by Python which returns an iterator object instead of returning a single value. The iterator object returned contains a sequence of values. It allows us to create our iterator function. In place of the return statement yield keyword is used in the generator function to pause the function execution. The difference between return and yield is that yield will pause the function execution and return will terminate the function action.
Comprehensions provide us with a shorthand syntax for iterable objects and collections creation.
Magic Methods and Operator Overloading
Magic methods are also called dunder or double underscore methods, magic methods are the special type of methods that are invoked internally. Double underscores are used in the starting and ending of magic methods. __lshift__(), __trunc__(), __str__(), __floor__(), __round__(), __abs__(), __add__(), etc. are some of the examples of magic methods. __add(10)__ is similar to writing an expresion number + 10.
A real-world use case for magic methods can be found in creating custom classes that emulate built-in types or support specific operations.
Operator Overloading:
In Python same operator can be used for multiple functionalities, like the + operator can be used for performing arithmetic addition for getting the sum of two numbers, and can also be used for string concatenation and merging the lists.
A real-world use case for operator overloading can be found in developing a class to represent and perform operations on complex numbers.
Threading and Concurrency
Thread is the smallest unit of the process which is scheduled by an operating system. Thread class is provided by Python which helps in multi-threaded programming. Multithreading makes the computation speed faster as multiple threads are there for task execution. Python threading module(thread module is deprecated now) is used for achieving multithreading in Python.
A real-world use case for threading and concurrency can be found in scenarios where a program needs to perform multiple tasks simultaneously to improve overall performance. One such example is a web server handling multiple client requests concurrently.
Example:
Output:
Regular Expressions
Regular Expressions is also an advanced Python concept that allows us to provide specific characters for pattern matching. It helps us in checking whether the strings contain the specified pattern or not. It is a fast, powerful, elegant, and concise way of pattern matching. We have to import the re module for working with regular expressions it involves multiple functions like split(), search(), findall(), etc.
Regular expression helps us in increasing code readability and makes the code faster.
In real-world applications, regular expressions are widely used for tasks such as form validation, log file parsing, data extraction, and more, where a specific pattern needs to be identified and validated within a larger set of data. A real-world use case for regular expressions (regex) is in data validation and parsing.
Object-Oriented Programming in Python
Object-oriented programming (OOP) in Python includes creating classes which are blueprints of objects and objects (an instance of a class). Classes consist of attributes and methods. Polymorphism, inheritance, and encapsulation are the key concepts of object-oriented programming used for organization and reusability of code.
In a real-world use case, consider developing a system for a university. Each student, professor, and course can be modeled as an object with attributes and behaviors. The Student class may encapsulate student information like name, ID, and courses enrolled. Professors, similarly, may have attributes such as name, ID, and courses taught. Courses can be represented as objects with details like name, schedule, and enrolled students.
Inheritance allows for creating specialized classes based on a general class. For instance, a Staff class can inherit properties from a common Person class, avoiding redundant code. This mirrors real-world relationships, enhancing code readability and minimizing duplication.
Polymorphism enables objects to take different forms based on their context. A method like calculate_grade can be implemented differently for students and professors, adhering to the principle of polymorphism. This flexibility fosters code adaptability and extension.
Additionally, OOP facilitates modular development. Each class serves as a module, and changes in one class do not necessarily impact others, promoting code modularity and ease of maintenance. For example, modifying the Student class doesn't affect the behavior of the Professor class.
Context Managers
Context Managers is also an advanced Python concept that is used for resource management. Resources such as database connections, and file operations are used widely, so we need to perform optimal management as we have minimal availability of the resources. And the main issue is releasing resources after using it. So resource's automatic setup and breakdown would prove very helpful for the users. So in Python, context managers help the users in simplifying the resource management.
Extended Keyword Arguments
Arguments are used for passing the parameters while calling the function. Sometimes we will use positional or keyword arguments. And often keyword arguments are used to make the calling the function more explicit.
- Extended Format Argument:
These are the arguments at the definition side of the function. - Extended Actual Argument:
These are the arguments at the calling side of the function. Argument at the function calls side.
Python Packages and Program Layout
A package in Python is a module that consists of other modules. Packages are mostly implemented in the form of directories which consist of a special file with the name __init__.py. And when the package is imported then this file will be executed.
Sub-packages are also included in the package, and every sub-package implements its __init__.py file.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.
Conclusion
- Python is a powerful and object-oriented programming language that is famous for its clarity and simplicity features.
- Python can be used in multiple domains like artificial intelligence, testing, automation, data analytics, web development, data science, machine learning, technical fields, etc.