What are the Advantages of Function in C?
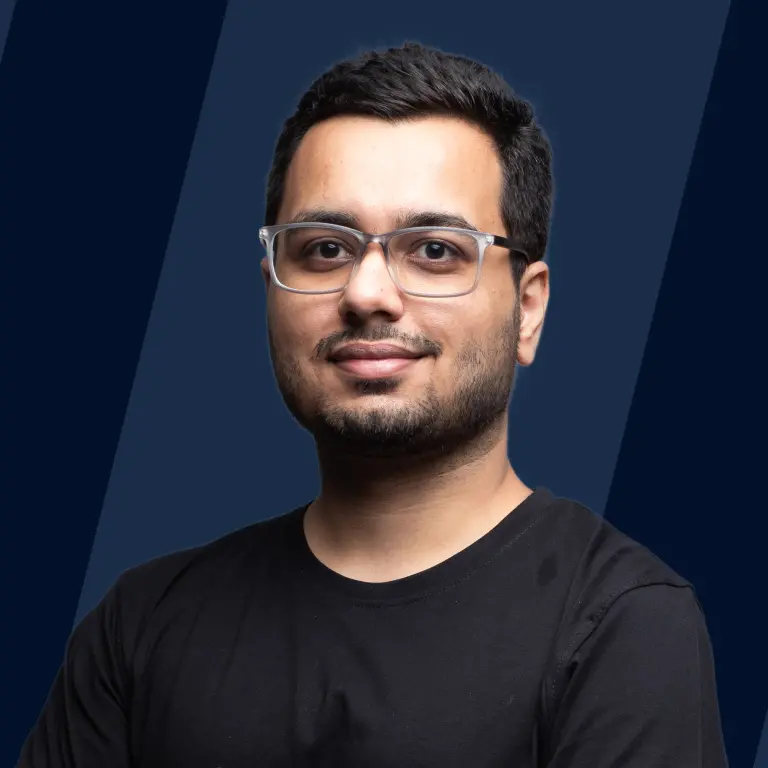
In this article, we will discuss the advantages of function in C. There are many advantages of function in C.
- Functions make a program more readable. Large programs are frequently difficult to read. Breaking the code into smaller functions keeps the program structured, understandable, and reusable.
- The function can be reused countless times after it is defined.
- A function can be used to reduce the size of a program by being called and used at different places in the program.
- Functions help in code modularity, which means that the entire code is divided into separate blocks, each self-contained and performing a different task. This makes each block implementation and debugging much more accessible.
- In top-down structured programming, dividing a program into functions is more efficient and easy to understand.
- If you just use the function in your program, you don’t have to worry about how it works inside.
Example: printf()
Introduction to Functions in C
- A function is a set of statements or a block of code that takes inputs, performs operations and produces the results.
- Before calling a function, it's preferable to declare it first.
- We could call the function multiple times.
Note: Providing inputs to the function is not mandatory. Without input, it can perform some computation, and it can return some output as well.
Syntax
Function aspects | Discription | Syntax |
---|---|---|
Return type | Return type indicates the type of output returned by the function | return_type |
Function name | Function name indicates the name of the function which we use to call the function | function_name |
Set of inputs | Set of inputs indicates inputs provided to the function | set_of_inputs |
Body | Body contain set of instructions function will perform on calling | Body_of_function |
Two Types of Functions
In C programming, functions are divided into two categories: library functions and user-defined functions. The malloc() and calloc() function is an example of a library function, and any function defined by the user is a user-defined function. The primary distinction between these two functions is that library functions do not require programmers to author them. Still, the programmer must develop a user-defined function while creating the program.
Built-in Functions/library Functions:
These are the pre-defined functions. As the name implies, these functions are pre-defined in the standard libraries. The header files can be used to include these libraries, and then we can call these pre-defined functions to do specified tasks.
Built-in Functions/library Functions | Use |
---|---|
strcat() | Appends string2 to string1 and ends the resulting with the null character. |
gets() | It's used to read keyboard string input. |
puts() | It is used to display only the string. |
malloc() | It is used to dynamically allocate a memory block. |
calloc() | It's used to allocate memory blocks of the specified type dynamically. |
pow() | It is used to get the power of a number. |
sqrt() | It's used to calculate a number's square root. |
User-defined Functions:
We'll have to declare and create our functions for case-specific functions that aren't defined in any header files. This can be achieved by using the syntax mentioned in previous sections. User-defined functions can be used to implement program-specific code.
User-defined Function | Use |
---|---|
main() | At runtime, it calls the programming code. |
There are 3 Aspects of Each C Function
Function aspects | Description | Syntax |
---|---|---|
function definition | Function definition consists of a block of code which is capable of performing some specific well-defined task. | return_type function_name(set_of_inputs){function body}; |
function call | To call a function, we always write its arguments in brackets with the name of that function. | function_name(set_of_inputs); |
function declaration | Declaration is used to tell the compiler about the function and its returning type, name, and parameters. | return_type function_name(set_of_inputs); |
Example of a Function
Below is the program for Area of Square:
Output:
In the above code, we defined a function square() to calculate the area of the square, which takes an integer as a parameter and returns an integer value.
Learn More
You may read functions in C resource to learn more about C Functions.
Conclusion
- A function has four parts: a return type, a function name, a set of parameters, and a function body.
- The function does not need inputs; it can still execute calculations and produce results without them.
- A function is an independently coded subprogram that performs a specific task, and it can be called anywhere in the program.
- In C, we looked at two different sorts of functions:
- Built-in Functions/library Functions
- User-Defined Functions
- function prototype always ends with the semicolon (;)
- It is not mandatory to put the name of the parameters in the function prototype.