How to Make an API Call in JavaScript?
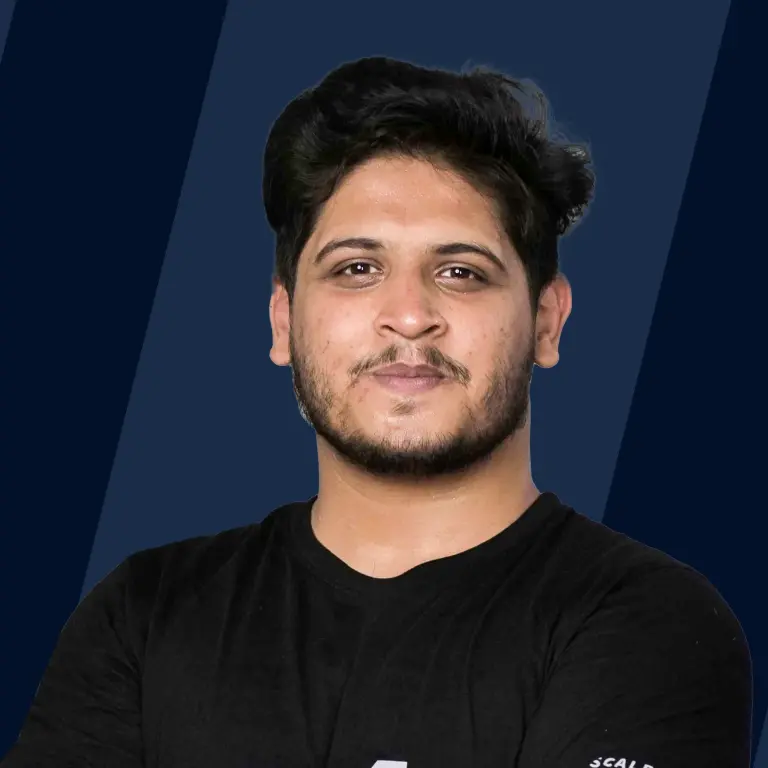
API is short for Application Programming Interface and is a software mediator that allows two or more applications to talk to each other. Every time you send a message to your friend over the internet or check the weather on your phone, you use API without knowing it.
The front end needs to create API requests to servers in the back end to show dynamic real-time content on the user's browsers. This creates a need to know how to make API calls in JavaScript and retrieve dynamic data from the servers. Let's see different ways we can use to create API calls in JavaScript discussed in this article.
4 Ways to Make an API Call in JavaScript
JavaScript provides a few built-in methods and external open-source libraries to create and interact with the API.
There are 4 possible methods to make an API call in JavaScript:
- Using XMLHttpRequest
- Using fetch() method
- Using Axios
- Using jQuery AJAX
API Call in JavaScript Using XMLHttpRequest
XMLHttpRequest was the only way to create HTTP requests in JavaScript before ES6 came out. It is a built-in browser object to request data from the servers and works in old as well as new browsers. Let's look at the code snippet mentioned below to see how to create requests using XMLHttpRequest.
XMLHttpRequest by default, receives responses in string format that needs to be parsed in JSON format (JSON.parse(XMLRequest.response)). This way of creating HTTP requests is deprecated with the introduction of fetch in ES 6. Still, it is used when we work with older browsers.
API Call in JavaScript Using fetch() Method
Fetch provides an interface to fetch resources asynchronously. This method returns a Promise and is an object that either contains a Response or an Error, and .then() tells the program what is to be done once Promise completes.
There are two ways we can create API calls using fetch.
Method 1
Method 2
The other way is to use async and await with fetch, as shown in the code snippet below.
The fetch API is a powerful way to create HTTP calls. The second method has a slight advantage because of improved readability in code. One disadvantage of fetch API is that we need to do error handling.
API Call in JavaScript Using Axios
Another possible method to create API calls in JavaScript is using an open-source library for making HTTP requests with several great features. Axios works in both Node.js and browsers and is a promise-based (asynchronous) HTTP client, which even is used in frameworks like Angular and React.
How to Install Axios?
1. Using Package Manager
If you are using package managers like npm or yarn, then we can install Axios as shown below.
and include it in the HTML file like
2. Using CDN
Another way to include Axios in HTML is by using its external CDN, as shown.
Now that we have successfully included Axios in our HTML file. Let's start creating HTTP requests with it.
Axios has the following advantages:
- Better and easier error handling.
- Axios has a wide range of browser support.
- Axios automatically performs JSON parsing and returns data in JSON format.
Because of the above-mentioned advantages, Axios is preferred over other methods to create asynchronous API calls.
API Call in JavaScript Using jQuery AJAX
jQuery provides several methods to handle asynchronous HTTP requests. Like Axios, in order to use jQuery in our HTML file, we need to include the source file of jQuery, which can be done as shown in the code snippet below.
jQuery uses $.ajax() method to make requests as shown below.
As shown in the example above, the $.ajax() method takes several parameters, some optional and some required. This method contains two callback functions: success and error to handle the received response easily.
Conclusion
- API is a software mediator that allows two or more applications to talk to each other.
- JavaScript provides a few built-in methods and external open-source libraries to create and interact with the API.
- The three commonly used methods to create API calls are:
- XML HTTP Request: It is a built-in browser object to request data from the servers and works in old as well as new browsers.
- Fetch: Returns a Promise and is an object that either contains a Response or an Error, and .then() tells the program what is to be done once the Promise completes.
- Axios: Axios is an open-source library that works in both Node.js and browsers and is a promise-based (asynchronous) HTTP client.
- Most modern applications use Axios to make HTTP requests because it is easy and is an open-source library to handle HTTP requests.