Calculate the Area of a Circle in Python
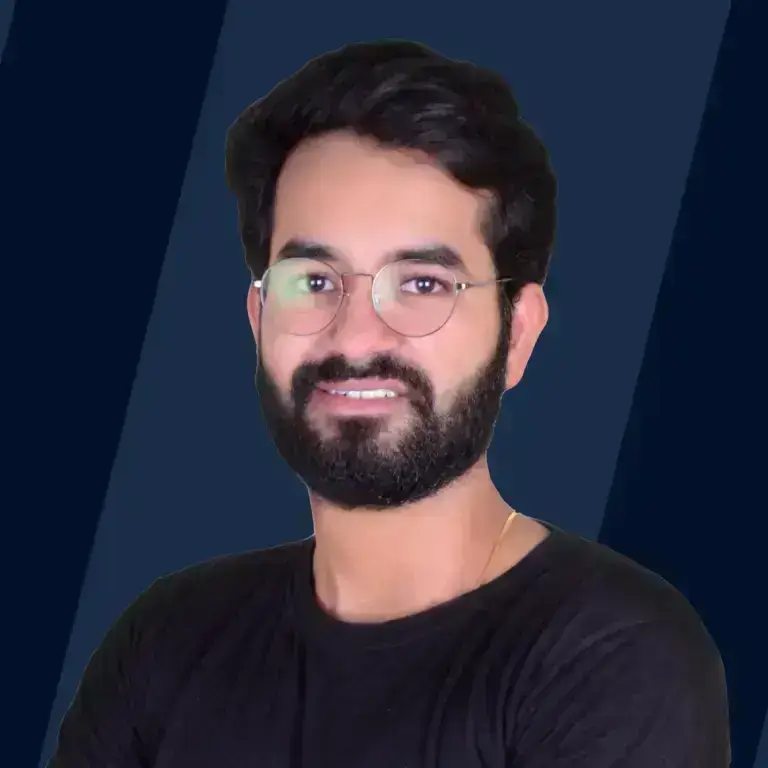
Overview
The calculation of the area of a circle in Python is quite simple and easy. In Python, we can use the power of the math library to simply square the radius and multiply it by the mathematical constant i.e. π (pi) to get the area of a circle. The concise formula is: area = π * radius^2. For example, if the radius is 5, we just need to write a single line of code i.e. area = 3.14159 * 5**2. Python easily computes the circle's area. We can use the math module, or we can even write our function for this easy calculation.
Algorithm for Calculating the Area
A simple code for calculating the area of the circle would be:
Let us now take a journey to calculate the area of a circle in Python and before moving into the above code's explanation, let's see what algorithm we need to follow to get to such code. The algorithm will be a combination of mathematical precision with the elegance and easiness of Python programming logic. In this exploration, we will look at the various algorithmic steps that lead to a clear code of the simple area calculation.
Let us look at the rough algorithm of the approach:
1. Define the Problem
The first and foremost thing in any problem is to clearly articulate the issue or the problem that we have to solve. Whether it is the area of a geometric shape or a region within a dataset, the clarity of the problem and its definition sets the stage.
2. Identify the Formula
After going through the problem statement, the next thing is to identify the appropriate formula for the area calculation. Different geometrical shapes require distinct formulas, be it the straightforward area of a rectangle or the calculation of the area of a circle.
3. Gather Inputs
User inputs play an important role and we need to take care of the input and its type so that no error occurs during our calculation. We should prompt the user for the necessary dimensions, radii, or any other parameters relevant to the formula that we have used. Ensure that the input values are accurate and appropriate for the selected geometric entity. To avoid any further error, we can re-prompt the user if the input is not a number.
4. Validation Checks
The next step in the process is to perform some robust validation checks on the input values. We need to ensure the input falls within the permissible ranges of values and is of the correct data type. This step helps us to guard our code and calculation against erroneous calculations. It also enhances the algorithm's reliability.
5. Execute the Formula
After getting the correct input, we need to invoke the identified formula using the validated inputs. This step transforms our input raw data into a meaningful result.
6. Output Display
After taking input and calculating the areas, we need to provide or output the calculated area to the user in a comprehensible format. A well-structured output always enhances the user experience and facilitates a seamless understanding of the algorithm's outcome.
7. Error Handling
We should anticipate potential errors or exceptions that may occur during the code execution. A good developer always handles the errors and exceptions that may occur in the code so that the code does not stop working in case of an exception. So, we need to implement error-handling mechanisms (using try-catch and finally blocks) to gracefully handle any unexpected scenarios. It ensures that the algorithm remains robust and user-friendly.
8. Testing and Refinement
Although this is a very simple problem, we should test the algorithm with various inputs that may be provided by the user. This iterative process helps us to check any bugs or logical issues.
9. Documentation
A developer should always document their algorithm and highlight its purpose, inputs, processes, and expected outputs. A well-documented algorithm not only adds future reference but also encourages collaboration among the developers.
Python Program to Find the Area of a Circle
Python is quite simple and versatile so it is a go-to language for various applications, including simple and complex mathematical computations. In this section, we will look into the calculation of the area of a circle using Python. We can calculate the area of a circle in Python in three various ways:
- using the math module
- using the π value
- creating a function for efficient and reusable code
Using the math Module
Python has a math module which is a powerhouse for various mathematical operations. To find the area of a circle, we can use the math module, we can use the pi constant value present in the math module and then put in the formula A = πr², where A is the area and r is the radius.
Let us now look at the code.
In the above code, we first ask the user for the radius, calculate the area using the formula, and finally display the result. The math.pi is a constant that provides a precise value for π. It also ensures accuracy in our calculations.
Using π Value
Another way to calculate the area of a circle is to directly use the π value in our calculation without importing the entire math module. This simple method simplifies the code and is suitable for scenarios where only basic mathematical operations are needed. This code becomes faster as we do not need to import any module.
Let us look at the code for the same:
In the above code, we first ask the user to input the radius and then we calculate the area using the formula and display the result. It is important to note here that using the π value directly provides a good approximation for most applications and makes our code faster.
Using a Function
To enhance code readability and reusability, we can go with the third approach i.e. encapsulating the area calculation in a function.
So, let us create a function named calculate_circle_area that takes the radius (provided by the user) as an argument and returns the area of the circle which we can display further.
So, by defining a function, we promote modular coding practices. This function can be easily integrated into large programs or Python scripts and helps to promote maintainability and reduce redundancy.
Comparing Methods
Calculating the area of a circle is a fundamental operation in mathematics, and Python provides various methods to perform this simple calculation. In this section, let us compare three popular approaches i.e. utilizing the math module, using the π value, and creating a dedicated function.
Using the Math Module:
The math module in Python is a powerful toolset for mathematical operations. To find the area of a circle using the math module, we use the predefined constant π (pi) present in the module and the formula A = π * r^2, where r is the radius of the circle. This method simplifies the code and makes it concise and readable.
Using π Value:
For a more simple approach, we can directly use the π value in the formula. This eliminates the need to import the math module and hence it reduces the code's complexity.
Using a Function:
To enhance code reusability and maintainability, we can encapsulate the calculation of the circle's area within a function. This approach enables us to call the function with different radius values while keeping the code modular.
Choosing the right method depends on the specific needs. If simplicity is the prime importance then we can use the π value directly. For more extensive mathematical operations, the math module provides additional functionality. Lastly, by encapsulating the calculation in a function, we promote code organization and reusability. Understanding these methods allows us to tailor our approach based on the requirements of our Python project.
Conclusion
- To enhance code reusability and maintainability, encapsulating the area calculation within a function is a wise choice. This approach enables us to call the function with different radius values while keeping the code modular.
- For a more simpler approach, we can directly use the π value in the formula. This approach eliminates the need to import the math module and hence reduces the code's complexity.
- Python's math module is a powerful toolset for mathematical operations. So, to find the area of a circle, we can use the math module. We can directly use the predefined constant π (pi) and the formula A = π * r^2, where 'r' is the radius. This method simplifies the code, making it concise and readable.
- For visualizing the data and the areas of the circle, we can use various libraries of Python such as MatplotLib.