How To Calculate the Area of a Rectangle in Java?
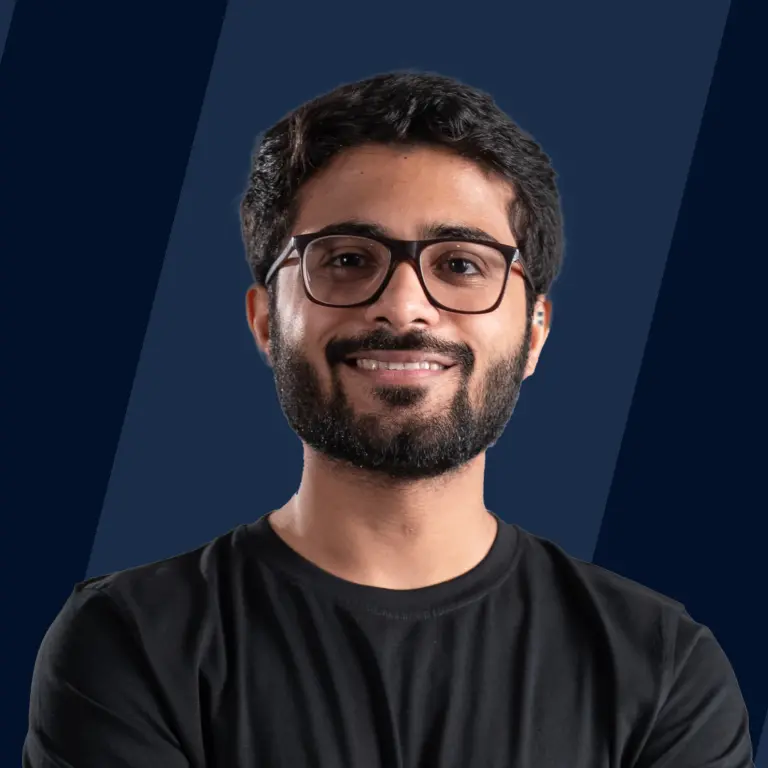
Area refers to the surface area covered by the shape. The rectangle is a geometric shape having four sides such that opposite sides of the rectangle are equal in length.
We have all learned that the area of the rectangle in Java is the product of the length and breadth of the rectangle. This area is represented in square units.
Area(R) = Length * Breadth
OR
Area(R) = Length * Width
The same can be done with a Java program. In this article, the focus is not only on calculating the area of a rectangle in Java but on creating a class, getting user input, validating the input, calculating the output, and printing it to the user.
Java Program to Calculate the Area of a Rectangle
Step 1:
Java is an Object Oriented Programming (OOP) and thus a class acts as a blueprint or template for creating objects. We will be creating a Rectangle class whose object will be a Rectangle having two variables length and width.
A public class in Java is created as follows:
Inside this class, we will have two private variables length and width to calculate the area of rectangle in Java. We will also need a constructor method to initialize these variables.
Constructor is a special method mainly used to validate and initialize class variables for the object. It has no return type as it returns nothing.
It takes values for variables as parameters. As in our case it will take length and width. We will initialize the object variables inside the constructor.
The private keyword makes the variables private to the class and guarantees proper authorized control. These can be accessed through public getters and setters methods.
Step 2:
Now, let us also create getters and setters. Getters and setters facilitate accessing object variables and managing them.
Getters and Setters ensures controlled access to data and prevents external data modification.
Step 3:
Next, we will create a method to calculate the area of rectangle in Java. This method will take two integer values length and breadth and will return the product of these two.
This method is public thus, we can access it outside the class. To ensure reusability, some classes are declared public.
This method is also declared static. To get access to static methods, objects of the class are not required. It is generally used for utility or calculation purposes.
Step 3:
Next, we will define the main method which is also known as the driver method. It is called the driver method because it drives the code i.e. execute the code in Java.
The format of the main method is as follows:
Inside the main method, we will take the user input for the length and width. We will then compute the area of Rectangle in Java and finally, we will print the area in the output.
Step 4:
We will use the Scanner class to get the user input. The scanner class is part of the java.util.* package.
The Scanner class's object is used to represent the input stream. It provides methods such as nextInt(), next(), nextLine(), nextFloat(), etc. to read data of various types.
In our code, we will read two integer values with the help of the Scanner class.
Initially, we are creating an object of the Scanner class. This object is then used to call the nextInt() method to get the input from the user.
Step 5:
Let us first create an object of the user-defined Rectangle class using the new keyword and passing the values for length and width.
Finally, we will call the method getArea() and pass user values to calculate the area of the rectangle in Java. Print this output to the user using System.out.println().
Complete code can be represented as follows:
Output:
Similarly, A parent class Shape can be created which will be a general class, and all the shapes such as Rectangle, Triangles, Circles, etc. can be derived as parent class. This way we can understand the relationship between the real world and programming. Understanding concepts concerning the real world gives us better clarity and builds logic.
The extend keyword lets our class inherit from a parent class.
Time and Space Complexity
Time complexity refers to the amount of time the program consumes to complete its execution. Similarly, space complexity is the amount of space required by the program.
As we are not using any loops and user input, the area of the rectangle in Java can be calculated including mathematical computation, and printing output are all done in constant time which is O(1).
To do these calculations we are not using any extra space in the form of an array or system stack and the space occupied is also constant.
Time Complexity:
Space Complexity:
Conclusion
- Java classes provide a blueprint for the objects. After defining the user class we can create its objects using the new keyword.
- The class must contain a constructor for initialization and getters and setters for access and modification of object variables.
- Utility methods can be declared static so that they can be called without the class object.
- The Scanner class of the java.util package is used to interact with the user and get custom input. We get the length and breadth from the user to calculate the area of the rectangle in Java.