argparse in Python
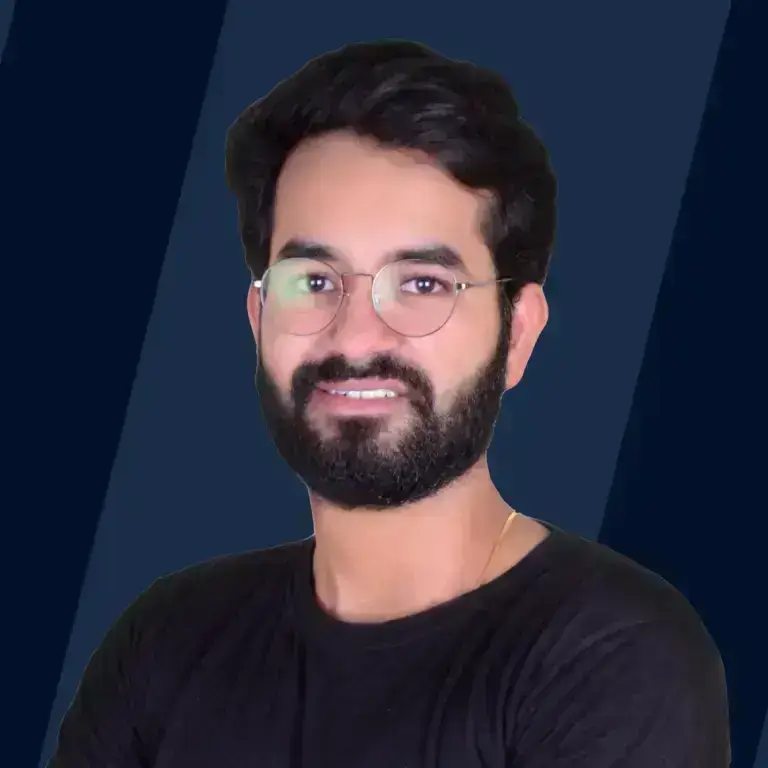
Overview
Python argparse is a command line parsing module to get command line arguments (the input parameters passed to your scripts during program execution) into your program. Python provides various ways to deal with these arguments. Some of the most common methods are: using sys.argv, using the getopt module, using the argparse module, and so on. In this article, we will see how the Python argparse module is effective and user-friendly.
What is argparse Module in Python?
Python argparse is used to accept arguments from the command line. As we have already seen, there are multiple ways to accept command line arguments, but using the argparse module is the most powerful way with minimal additional code required. Or, instead of having to manually set variables inside the code, the argparse module could be used to add reusability and flexibility to your code.
Steps to Use argparse Python Module
Now, let's look into the steps to use the argparse Python module.
Creating a Parser
Description:
To create a parser, first, we have to import the argparse module. After we have imported the argparse module, we now have to create a parser that will store all the necessary information that has to be passed from the command line.
Syntax:
Parameters:
- prog: The name of the program (default: os.path.basename(sys.argv[0])).
- usage: The string describing the program usage (default: generated from arguments added to parser).
- description: Text to display before the argument help (default: none).
- epilog: Text to display after the argument help (default: none).
- parents: A list of ArgumentParser objects whose arguments should also be included.
- formatter_class: A class for customizing the help output.
- prefix_chars: The set of characters that prefix optional arguments (default: ‘-‘).
- fromfile_prefix_chars: The set of characters that prefix files from which additional arguments should be read (default: None).
- argument_default: The global default value for arguments (default: None).
- conflict_handler: The strategy for resolving conflicting optionals (usually unnecessary).
- add_help: Add a -h/--help option to the parser (default: True).
- allow_abbrev: Allows long options to be abbreviated if the abbreviation is unambiguous. (default: True).
- exit_on_error: Determines whether or not ArgumentParser exits with error info when an error occurs. (default: True).
Argument Addition
Description: Now we will provide information about the arguments to the ArgumentParser. For this, we'll use the add_argument() method. This information tells ArgumentParser how to take arguments from the command line and turn them into objects.
Syntax:
Parameters:
- name or flags: Either a name or a list of option strings, e.g. foo or -f,--foo.
- action: The basic type of action to be taken when this argument is encountered at the command line.
- nargs: The number of command-line arguments that should be consumed.
- const: A constant value required by some action and nargs selections.
- default: The value produced if the argument is absent from the command line and if it is absent from the namespace object.
- type: The type to which the command-line argument should be converted.
- choices: A container of the allowable values for the argument.
- required: Whether or not the command-line option may be omitted (optionals only).
- help: A brief description of what the argument does.
- metavar: A name for the argument in usage messages.
- dest: The name of the attribute to be added to the object returned by parse_args().
Parsing Argument
Description: In the add_argument() method, we have provided the information about the arguments to the ArgumentParser. Initially, the data is stored in sys.argv array in a string format. parse_args() converts argument strings to objects and assigns them as attributes of the namespace.
Previous calls to add_argument() determine exactly what objects are created and how they are assigned.
Syntax:
Parameters:
- args: List of strings to parse. The default is taken from sys.argv.
- namespace: An object to take the attributes. The default is a new empty Namespace object.
Command Line Interface
Description
The command line interface is also known as the CLI. A command-line interface interacts with a command-line script. Python has many libraries that allow us to interact with the command-line interface, but Argparse is the most suitable for interacting with a command-line script.
Types of Arguments in CLI
Two arguments that include in the command line interface are:
- Positional Argument
- Optional Argument
Let's try to understand both arguments:
1. Positional Argument:
Sometimes we don't need to use the flag's name in the argument because we can use the positional argument to eliminate the need to use the flag name before inputting the actual value. Although positional arguments make the command line cleaner, sometimes it's become difficult to tell what the actual field is that we are passing because no name is associated with it. To overcome this, we can use the help parameter in the add_argument() function to specify more details about the argument.
2. Optional Argument:
Optional arguments are not mandatory because they are used to give the user a choice to enable certain features or not. Let's say in input we pass the name and age of a person. Then we will make our program something that if we don't passage, then it will still print some output. To add an optional argument, we have to use the required parameter in the add_argument() method. If we set the required to true, then we have to pass that parameter, else it is optional.
Examples
Let's have a look at the examples of positional and optional arguments.
Example 1: Multiply two numbers without positional arguments
Code:
Output:
In the above output, you can see that we are passing arguments ( and ). There is no need to pass these arguments; we can also perform operations without passing them.
Example 2: Multiply two numbers with positional arguments
Code:
Output:
By default, the positional arguments are treated as strings, but we can typecast them to other data types, as you can see in the above code. We are passing two numbers of an integer type as positional arguments.
Example 3: Working with strings with and without optional arguments.
Code:
Output:
In the above code, we can see two arguments: the name and the roll number. Here the name is required (if you don't pass, then it will give an error) and age is an optional argument.
Argparse Python Examples
Find the Sum of Command Line Arguments using Argparse Python
Code:
Output:
Arranging Integer Inputs in Ascending Order Using Argparse Python
Code:
Output:
Find the Average of the Entered Command Line Numerical Arguments
Code:
Output:
Conclusion
Let's conclude our topic Argparse Python by mentioning some of the important points:
- Python argparse is a command line parsing module to get command line arguments (the input parameters passed to your scripts during program execution) into your program.
- Instead of having to manually set variables inside the code, the python argparse module could be used to add reusability and flexibility to your code.
- To create a parser, first, we have to import the argparse module. Now, create a parser that will store all the necessary information that has to be passed from the command line.
- Filling an ArgumentParser with information about program arguments is done by making calls to the add_argument() method.
- parse_args() convert argument strings to objects and assign them as attributes of the namespace.
- Two arguments that includes in command line interface are: Positional Argument and Optional Argument.