What are Argument Objects in JavaScript?
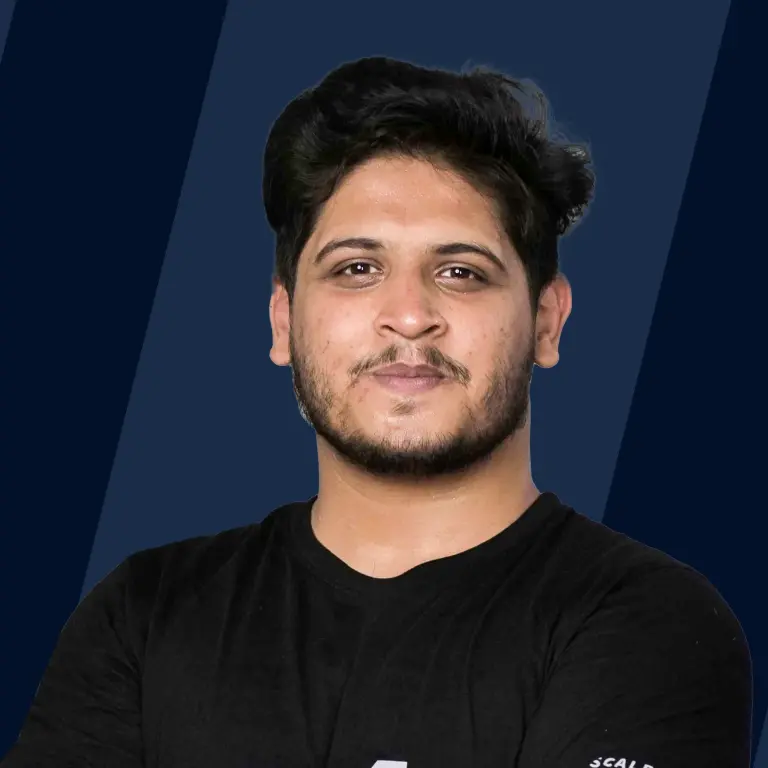
What are argument Objects in JavaScript?
The arguments in JavaScript is an object that is available to use only inside the function. It is like a local variable that is available to all with all functions except the arrow function. This argument variable helps us to access the args that are passed to the function. These arguments can be accessed by using the index.
All the arguments that are passed to the function are stored in the arguments object. This args javascript object can be used with functions that can use more arguments than they were formally defined to accept.
Syntax
This is the syntax you have to follow to use arguments in JavaScript.
Output
Using typeof with Arguments
You must be wondering what is the type of data that is stored in the arguments object. The args JavaScript object is quite flexible in this case. It automatically infers the type from the data that is passed to it.
Let's understand this with the help of a simple function.
This is a simple function that will print the type of argument it will receive. Let's test this function for different inputs.
Output
Type of argument is: string
Type of argument is: object
With these examples, it is clear that the arguments object can automatically infer the type from the data it gets.
Properties of args JavaScript
length
length is a property of args JavaScript that returns the number of arguments that are passed to the function.
Output
callee
callee is property of args that contains the reference of the currently executing function.
Output
arguments[@@iterator]
This property of arguments returns an Array iterator object that contains values for each of the indexes in arguments.
Output
Examples
Example 1: Defining a Function that Concatenates Several Strings
Output
The above code uses an arguments object to access the strings that are passed as arguments to concatString function. Then we can add all the argument strings to a single string using a for in loop.
Defining a Function that creates HTML Lists
Output
This code looks complex, but it's very simple. For creating HTML lists, we have to decide whether we want an ordered List ol or an unordered list ul. The list items are defined by the li tag.
So, we take the type as an argument first and create a tag. Then we use splice to get all the arguments other than type.
If we call args separately,
Output
How we can access the Arguments of a Function with the object Arguments
As the arguments object is available to all the functions in JavaScript except arrow functions, we can easily access the arguments passed to the function with the help of this args javascript.
Output
Convert the Arguments Object into an Array
There are many ways in which the object can be converted into an array.
Using array.from()
Output:
Using Array.prototype.slice()
Output:
Using Object.values() method
Output :
Using spread Operator
Output:
In this example we have used the Javascript spread syntax to convert the object into array. The work of spread operator is to enumerate the properties of an object and add the key-value pairs to the object being created.
The Rest Parameter
Simply put, the rest parameter acts as a collector by gathering the other parameters.
The rest parameter allows the function to accept an indefinite number of arguments as a array.
Output:
Conclusion
After reading this article you have learnt that
- arguments is an object in JavaScript that stores all the arguments passed to the function.
- arguments object is available only within the function.
- This args javascript is not available for arrow functions.
- args javascript can automatically infer the type of arguments.
- There are three properties of arguments object
- length
- callee
- arguments[@@iterator]
- The rest parameter collects the arguments.
- Rest parameter allows the function to accept an indefinite number of arguments.