Armstrong Number in JavaScript
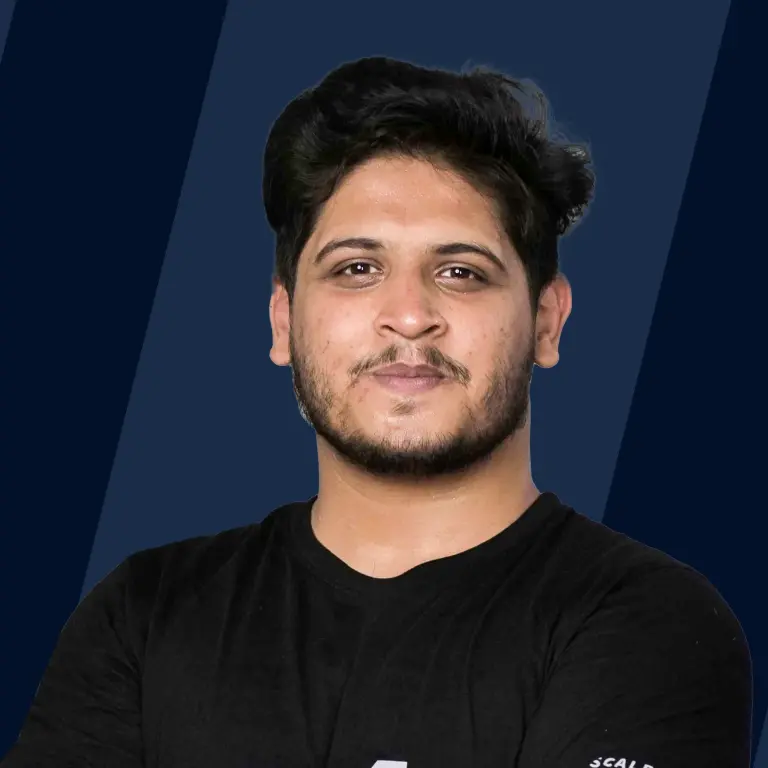
An Armstrong number is a special integer where the sum of its digits, each raised to the power of the number's length, equals the number itself. For instance, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
Armstrong Number in JavaScript
We can detect whether a given number is an Armstrong number or not by using a simple javascript program. The Javascript program will take an integer as an input and will tell whether the input number is an Armstrong number or not. The subsequent sections will discuss how this program will be written in javascript.
The Logic of Armstrong Number in JavaScript
In the javascript program to detect whether a number is Armstrong or not, we will take an integer as an input. By definition of the Armstrong number, which is also discussed above, if the number has n digits in it, it is said to be an Armstrong number if the sum of the nth power of all the individual digits is the same as the input number itself. So, the logic to detect the Armstrong number will be as follows.
- We will extract all the digits of the input integer by using a while or a for loop.
- We will take the nth power of all the extracted digits and find the sum of these nth power of the digits. If the resulting sum is the same as the original input number, then the input number is an Armstrong number. If the resulting sum is not the same as the original number, then it is not an Armstrong number. If we talk about the time complexity, the time complexity to find out whether an integer 'n' is an Armstrong number or not is O(log(n)).
Given below is the flow chart of the javascript program to detect whether a number is an Armstrong number or not.
Examples of Armstrong Number in JavaScript
Using while loop
The code given below detects whether the input number is an Armstrong number or not using a while loop.
Output:
The output for the input number "123" is given below.
The output for the input number "9474" is given below.
Using for a loop
The code given below detects whether the input number is an Armstrong number or not using a for loop.
Output:
The output for the input number "123" is given below.
The output for the input number "9474" is given below.
Check Armstrong Number of n Digits
The code given below detects whether the three digit input number is an Armstrong number or not.
Output:
The output for the input number "12367" is given below.
The output for the input number "54748" is given below.
Conclusion
- An n digit integer is said to be an Armstrong number if the sum of the nth power of all the individual digits is the same as the number itself.
- Any number can be checked whether it is an Armstrong number or not by passing the taken number into a simple javascript program.
- The time complexity to find whether an integer 'n' is an Armstrong number or not is O(log(n)).