Array Contains in JavaScript
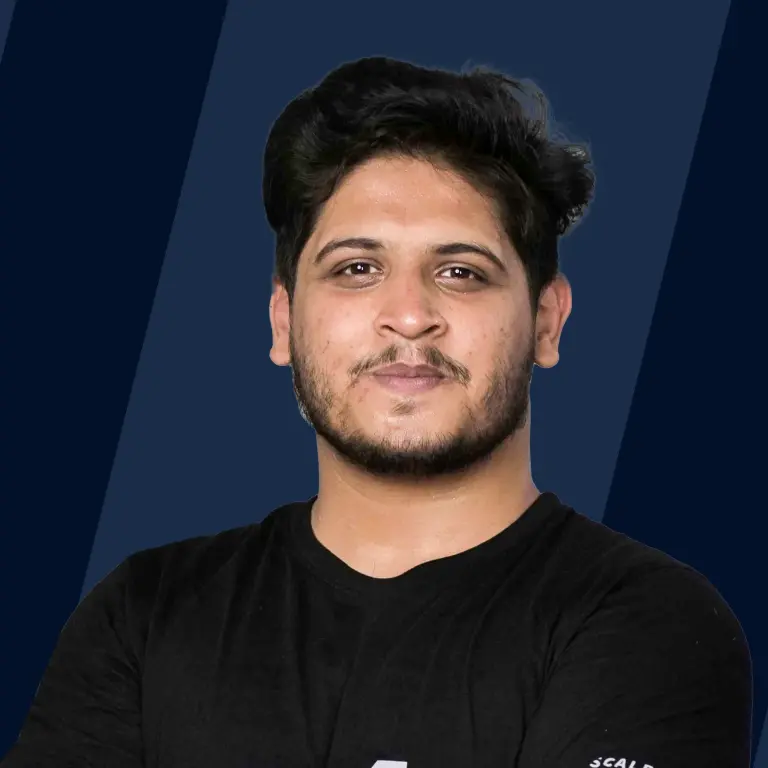
The includes() method in JavaScript is a built-in function for Arrays, determining whether a specified value exists within an array and returning a boolean. Analogous to checking the refrigerator for food when hungry, includes() searches for elements in an array, returning true if found, allowing subsequent actions based on specific requirements.
Syntax
The syntax of includes() method in JavaScript is:
Parameters
There can be a maximum of two parameters of the includes() method:
- value (must): It is the value or the element to look for.
- indexToSearchFrom (optional): It is the index value in the array from which the search for the element should begin.
Return Value
Return Type: Boolean
The includes() method returns a boolean value, either true or false, depending upon the value passed in the method.
If the passed value is present in the specified array, true is returned, else false is returned.
Example of Array Contains in JavaScript
Let us see some examples for a better understanding of the includes() method in JavaScript.
Code:
Output:
Explanation:
In the above-given example, we have an array called subjects which has three items Physics, Chemistry and Maths in it.
- In the first console.log statement, we used the Array.includes() method to see if the item Chemistry is present in the array or not, as it was there in the array we got true in the console.
- In the second console.log statement, we did the same step to check if "Biology" is there in the array, as it was not there we got false in the console.
Array Contains a Primitive Value
There are seven primitive values in JavaScript: string, number, boolean, undefined, null, bigint, and symbol. We can use the includes() method to check if the array contains JavaScript a primitive value or not.
Example:
Code:
Output:
Explanation:
In the above-given example, we have an array called randomArray in which we stored some primitive values in a random order of occurrence. We used the includes() method to check if the provided primitive values are present in the array.
- In the 1st console.log statement, we checked if the string value "orange" is present in the array, as it was there in the array we got true in the console.
- In the 2nd console.log statement, we checked if the string value "range" is present in the array, as it was not there in the array we got false in the console.
- In the 3rd console.log statement, we checked if the undefined value undefined is present in the array, as it was there in the array we got true in the console.
- In the 4th console.log statement, we checked if the number value 2 is present in the array, as it was there in the array we got true in the console.
- In the 5th console.log statement, we checked if the number value 6 is present in the array, as it was not there in the array we got false in the console.
- In the 6th console.log statement, we checked if the boolean value false is present in the array, as it was there in the array we got true in the console.
- In the 7th console.log statement, we checked if the null value null is present in the array, as it was there in the array we got true in the console.
Searching From an Index
We have seen in the Parameters section of this article that we can search for the array element from any applicable desired index value in the array. Let us see some examples of the same.
Code:
Output:
Explanation:
In the above-given example, we have an array called numsArray which contains numbers from 0 to 6. We used the includes() method with the optional argument which is the index from which it starts searching the element till the end of the array.
- In the 1st console.log statement, we checked the numsArr from index position 2 if it contains the value 1, as it's not present in the given range of array, we got false in the console.
- In the 2nd console.log statement, we checked the numsArr from index position 1 if it contains the value 1, as it is present in the given range of array, we got true in the console.
- In the 3rd console.log statement, we checked the numsArr from index position 1 if it contains the value "1", as it is a numeric string that is not present in the given range of array, we got false in the console.
- In the 4th console.log statement, we checked the numsArr from index position 1 if it contains the value 7, as it is out of the given range of array, we got false in the console.
Passing No Arguments
If we don't pass any arguments in the includes() method, we get false in the output. Let us see an example of the same.
Code:
Output:
Explanation:
In the above-given example, we have numsArray that we created in the previous example. We used the includes() method without passing any argument hence got false in the console.
Array Contains an Object
There can be situations when we want to check if an array contains JavaScript an object having a property equal to the passed value. The question arises, Can we use the includes() method for the same? The answer is "No". Let us understand this with the help of an example:
Code:
Output:
Explanation:
In the above-given example, we used the includes() method to check if the array contains JavaScript the object and an object having the passed property's value. Even though it contains the object and the object with the brand value 'HP', it returned false in both the cases. Hence we can not check this using the includes() method. Let us see how to solve this problem using a simple logical program.
Solution to this problem:
Code:
Output:
Explanation:
In the above-given example, we created a function to find the passed value in the object. We just used a for loop and an if condition to achieve the correct results. The for loop iterates over the whole object sequentially while the if the condition checks for the passed value in each object's property called brand. This is how we can check if an array is an object with the passed value.
Check If the Array Contains Objects Using the Higher-Order Functions
The “Higher-Order Functions” are the functions that accepts another functions as arguments and(/or) returns a function using that argument function. We can also check if an array contains JavaScript an object using the Higher-Order Functions like filter() and some(). Let us discuss each of these in detail with the help of examples.
Check If Array Contains Objects Using filter() and Arrow Function
Here we will use the filter() method with an arrow function to check if the array contains JavaScript the object. Before proceeding ahead, let us quickly revise the definition of the filter() method in JavaScript.
Definition:
The filter() method accepts a callback function that has a certain condition or test. It checks that condition for all the elements of the array and creates another array that consists of only those elements which passed the provided condition or test. Hence we can use the filter() method to check if a specific element is present in the array.
Code:
Output:
Explanation:
In the above-given example, we used the filter() method with an arrow function to check if the array contains JavaScript the object. There's a callback function in the filter() method to check if the element is present in the array or not. We know that the filter() method creates a new array containing the elements that satisfy the given condition, hence if there's an element(or object) that satisfies the given condition, it gets added to the new array in such cases the array length must be greater than zero.
Hence we are using the length property of the array to validate if the array length is positive(>0) or not, if its positive we update the value of isPresent from false to true. This is how we can use the filter() method to check if the provided object is present in the array or not.
Check If Array Contains Objects Using some() and Arrow Function
We can also use the some() method with an arrow function to check if the array contains the object with the provided value. Before proceeding ahead, let us quickly revise the definition of the some() method in JavaScript.
Using some() method:
Definition:
The some() method accepts a callback function that has a certain condition or test. It checks if any element of the array passes the test given by the provided function. If any element passes the test, it returns true else if no element in the array pass the test, it returns false. Hence we can use the some() method to check if a specific element is present in the array.
Code:
Output:
Explanation:
In the above-given example, we used the some() method with an arrow function to check if the array contains the object. There's a callback function in the some() method to check if the element is present in the array or not. We know that the some() method do not create a new array hence the array length condition is not required here. If it finds an element that matches the condition, it simply returns true or else it returns false. If the element is found, in such case we update the value of isPresent from false to true. This is how we can use the some() method to check if the provided object is present in the array or not.
The main difference between some() and filter() method is that some() returns a boolean value true if it finds atleast one element in the array that passed its test while filter() creates a new array containing only those filtered elements which passed its test.
Array Contains Another Array Element in JavaScript
We can use some() method in combination with the includes() method to check if the array contains in JavaScript atleast an element same as in another array. Code:
Output:
Explanation:
In the above-given example, we have 3 arrays namely arr1, arr2, and arr3. We used the some() method to check if the array contains another array having at least one element same in both the arrays. As the arr1 and arr2 have one element in common, isPresent1 returned true. In contrast to this, as arr1 and arr3 have nothing in common, isPresent2 returned false.
Javascript Array Contains vs. Includes
There's no built-in method called Array.contains() in JavaScript to check for the element in an array. Instead of this, we have the Array.includes() method to check if an element is present in the array. We have discussed this in detail in the above sections of this article.
Conclusion
- The Array includes() in JavaScript is one of the built-in methods of Arrays used to check if an Array contains the specified value or not.
- The includes() method returns a boolean value, either true or false, depending upon the value passed in the method.
- If we don't pass any arguments in the includes() method, we get false in the output.
- Higher-Order Functions like filter() and some() can be used to check if an array contains an object.