Arrays.fill() in Java with Examples
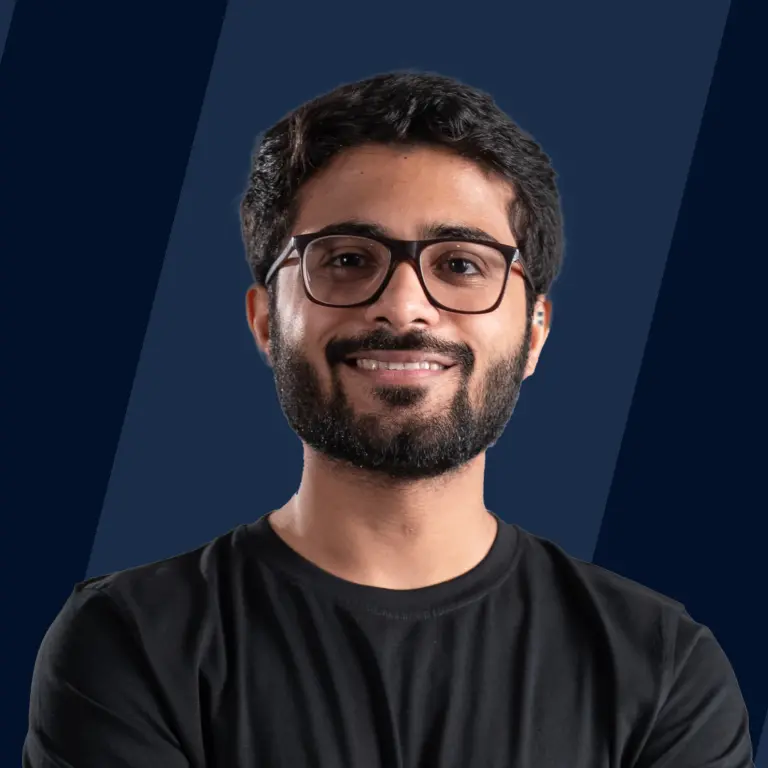
Overview
Array is a widely used data structure and Java provides many useful methods to facilitate operations on arrays. The java.util contains many such methods and one of them is Arrays.fill() method in Java which is used to fill an array with a specified value. It also provides the facility to fill an array with a default value partially. It is useful to avoid null and garbage values.
What is Arrays.fill() in Java?
It is a method present in the Arrays class of java.util package of Java. The java.util package is a built-in package that provides a variety of utility classes and interfaces for common tasks, such as data structures, collections, internationalization, and I/O.
Arrays are a fixed-size data structure that stores values of the same data type. Sometimes to avoid null errors, garbage values, or incomplete arrays we use the Arrays.fill in Java to fill the array with some constant or default value.
We can either fill the entire array or some parts of the array. This method is capable of not only filling 2D arrays but we can also fill 3D arrays.
Syntax
The method syntax is as follows:
The Object can be of any type. We can use int arrays, boolean arrays, string arrays, etc. The value to be filled must match the type of the array.
Parameters
It takes four parameters out of which two are mandatory and two are optional.
Required Parameters:
- Array: The array into which we want to fill values is to be passed.
- Value: The default value to be filled inside the array.
Optional Parameter:
- fromIndex: the starting index from which it will start filling the given value.
- toIndex: It defines the last index to be filled with the given values.
Return Values
The role of the method Arrays.fill in Java is to fill the specified value at the required positions. It doesn't return any value when called.
Exceptions
Arrays.fill in Java throws two exceptions as follows:
- IllegalArgumentException - This is thrown when user enters fromIndex greater than the toIndex.
- ArrayIndexOutOfBoundsException - If any of the indices is greater than the length of the array or less than 0, this exception is thrown.
We can handle these exceptions with the help of try-catch block. It is mainly useful when we are taking input from the user and user may enter some invalid values. An example of handling exceptions is discussed in coming sections.
Examples
Let us look at examples of the Arrays.fill in Java.
Filling Entire Array
Let us look at an example where we will be filling the entire array with some fixed value.
Output:
Explanation: Here, we are initializing an array of size 10 and integer data type. Initially, integer arrays have the default value 0. Next, we fill the array with the value -1. We can perform operations on this array. We are traversing the array and inserting 3 wherever the index is divisible by 3.
The Arrays.toString() method is used to convert an array to a string which makes array visualization easier. It is also present in the java.util package.
Filling Part of an Array
Let us now explore how we can partially fill the default value inside an array with the help of fromIndex and toIndex.
We will implement a stack with the help of an array. Inside the stack we will be able to push elements, pop elements, see the element at the top, check the size of the stack, check if the stack is empty, and clear the stack. The clear method will make use of Arrays.fill() to clear the stack and insert zeros inside the stack from the 0^th^ index to the top index.
Output:
Explanation: In the above example, we are implementing a stack with the help of an array. The stack has three properties top, size, and array to store data. It has various methods,
- pop - remove an element from the stack
- push - add an element to the stack
- peep - see the element at the top
- size - number of elements in the stack
- isEmpty - checks if the stack is empty i.e. has no data
- clear - clears the stack, removes all the elements
The clear method in the above example is used to demonstrate the real-life use case of the Arrays.fill in Java. We are selectively clearing the array with clean and concise code.
Exception Handling: Providing invalid values while partially filling arrays may result in exceptions at runtime and thus exception handling is important. Let us see how we can handle these exceptions:
Output:
Explanation: The above code first initializes array with some specific value. It then tries to fill particular indices. We are writing the code in try-catch block. The catch block takes IllegalArgumentException, IndexOutOfBoundsException and the third Exception for any other exception thrown in case such as NullPointerException.
Filling Multidimensional Array
So far we have only used this method on the 1D array, let us now see how we can fill multidimensional arrays with the Arrays.fill() method.
2D Array We can use a for loop to fill each row of the 2D array. A 2D array can be thought of as an array of arrays. So when we traverse through the array, each element in the 2D array is also an array of 1 Dimension. We can use Arrays.fill() method to fill each of these arrays.
Output:
Explanation: We are using a for each loop to select each row of the array. Each row represents a 1D array. We use the method Arrays.fill() method to fill each of these rows. Here, we are using a boolean array.
3D Array Similarly, we can add one more for each loop and fill each 2D array as shown in the above example.
Output:
Explanation: The above example represents a 3D array of strings. We know that initially, each string inside the array has a null value. We use two for each loop to get each row and fill it with the desired string value.
FAQs
Q. How do I use the Arrays.fill() in Java?
A. To use the Arrays.fill() method, you simply pass the array you want to fill and the value you want to fill it with to the method. For example, the following code fills an array of integers with the value 10:
Q. What are the benefits of using the Arrays.fill() method?
A. There are several benefits to using the Arrays.fill() method:
- It is very efficient, especially for large arrays.
- It can be used to initialize arrays to avoid null errors, garbage values, or incomplete arrays.
- It can be used to clear arrays.
Q. What are some limitations of the Arrays.fill() in Java method?
A. The Arrays.fill() method cannot be used to fill arrays with multiple values. To do this, you can use a for loop or the Stream API.
Conclusion
- Arrays are important data structures for every programming language. The java.util package provides a method Arrays.fill in java to fill the array with some default or constant value.
- It takes four parameters, the array, the value, the starting index, and the ending index. The array and value must be passed to this method.
- The indices are optional, they are used to fill values between particular ranges in the array.
- Arrays.fill in Java works with all the data types such as boolean, string, double, character, etc. The type of the array and the data type of the value must be same.
- It throws two exceptions, IllrgalArgumentException when the starting index > ending index and ArrayIndexOutOfBoundsException when either of the indices is invalid.
- We can use an additional for each loop to fill the 2D arrays.
- Similarly, we can add more nested for loops to fill the more dimensional arrays.