How to Create a 1D and 2D Array of pointers C++?
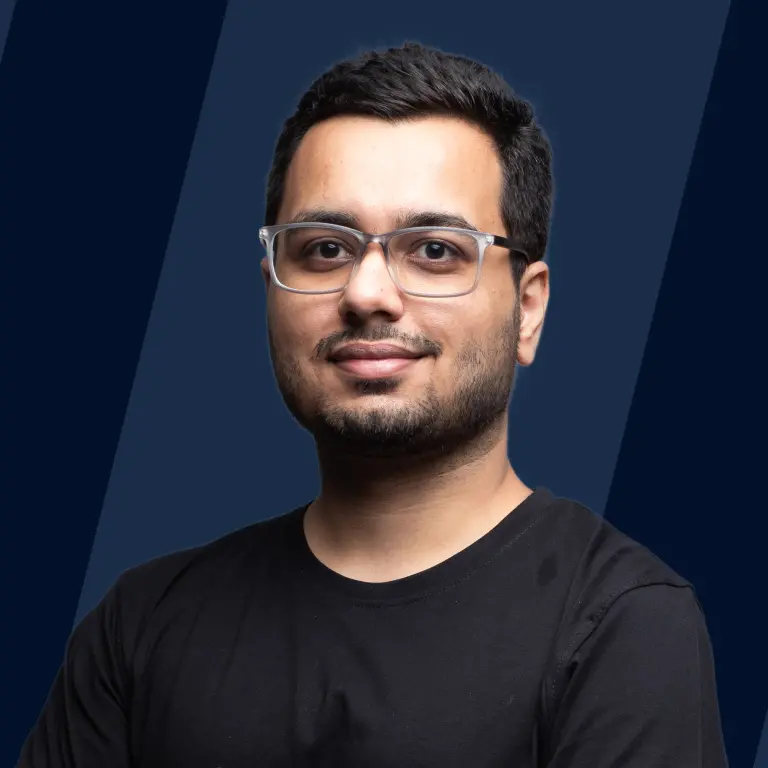
How to Create a 1D and 2D Array of Pointers in C++
In this article, we will discuss how we can create a one-dimensional as well as a two-dimensional array of pointers in C++. First, we will discuss the array of pointers, how they are declared and defined in C++, and their syntax. Next, we will see different ways of creating them i.e. through static allocation and dynamic allocation. Further we talk about how to access an array of pointers in C++ and how they are used while dynamically creating a two-dimensional array.
What is an Array of pointers in C++?
An array is a collection of elements having similar data types stored at contiguous memory locations. These data types can be integers, characters, etc. Similarly, if this data type is a pointer, we can also have an array of pointers. Pointers store addresses (memory locations) of variables. Hence, they are said to point to those variables whose addresses they are storing. They are distinguished from normal variables (normal variable here refers to variables that store values and not addresses) by having an asterisk (‘*’) along with the data type they store the address of.
For example:
Here the variable ‘ptr’, a pointer to an integer, is assigned the address of integer variable a. The ampersand operator (‘&’) refers to the address of the variable it is operated upon. We can say pointer ptr points to integer variable a. So, an array of pointers in C++ is simply an array containing pointers to other objects. It is also important to distinguish between a pointer to an array and ‘an array of pointers’. A pointer can point to any object, which can also be an array. This pointer will be called a pointer to an array. To assign the address of the array to the pointer, we simply use the ampersand operator (&) on the name of the array. This is because the array's name contains the array's base address (the address of the first element).
For example:
Here the ptr still contains type int because it contains the address of the first element of the array (the base address) which is an integer.
Creating an array of pointers in C++:
An array in C++ is represented as follows:
<data_type>
For example:
Similarly, an array of pointers in C++ can be created as:
Here, ‘d’ is a pointer to integer variable ‘c’ and is stored in the array of pointers. Moreover, the addresses of integer variables can also be directly stored in the array as shown in the case of variables ‘a’ and ‘b’. The elements of the array can be accessed just like any other array using a ‘[]’ operator. This way, an array can be created and initialized at the compile time. The array will be located in the stack. However, Arrays of Pointers play an important role in dynamic allocation. A dynamic 2-dimensional array of any data type is eventually represented as an array of pointers. To understand this, let’s see how an array is created dynamically.
Creating an Array Dynamically
First, let’s understand why we would need to create an array dynamically. This is because, in many programs or software, we may involve variables that have a limited lifecycle and are created, accessed, and deleted within the runtime of the program. To store such variables which are so dynamic throughout the program execution, a dynamic array would also be needed. The size of such an array can also be determined at the runtime, as per the user’s data, and need not be fixed beforehand.
How to create a 1D Array Dynamically?
For dynamic creation, C++ offers a keyword ‘new’ which allocates the memory of the requested data type in the heap section of the memory. While the stack is used for storing the local and static variables whose memory to be occupied is known before program execution, the variables that are created at runtime and whose size isn’t predetermined, are allocated memory in the heap. The new keyword creates a block of memory according to the requested data type and size, and it returns the address of the first memory block. Every other memory element can be accessed using this returned address. Hence, all data that is present in the heap section of memory is accessed through pointers. Hence, you can see the relevance of pointers in dynamic creation, which will be intensified in the case of 2-dimensional arrays.
Syntax:
<datatype>* <pointerName> = new <dataType>[<size>]
The
Example:
Accessing Array Elements:
As said, the new keyword returns the base address of the created block, hence for the above example variable ‘ptr’ contains the base address of the allocated memory. To access any other memory location, we simply add its index (relative to the first element). For example, we dynamically create an integer array where the base address is returned in variable ‘ptr’ and is 2000, now the second element of the array can be accessed as (ptr+2) whose address will be 2000 + 2*4 = 2008. (because an integer takes 4 bytes in C++) The data contained in the variable pointed by a pointer can be accessed by using the ‘ * ’ operator. This is called the dereference operator. Simple array access using the [] operator is also possible.
For example,
Hence, *(p+2) will be the value present at the second index of the array, while *( p )+2 will be the sum of the values present at the zeroth index of the array and 2.
Code:
Output:
(b) Creating a 2-dimensional array dynamically A dynamic 2D array can be represented as an ‘array of pointers’ where every index of this array points to an array of memory blocks. Such a representation gives a 2-dimensional view of the array. The following diagram describes the below idea.
Syntax:
Accessing Array Elements:
Accessing array elements follows the same rules as in the one-dimensional case. The difference appears in the fact that first, we need to apply a dereference operator on the array of pointers at the required index. It will return us the address of the array to which it points to. Then we will add an index accordingly to reach our data and dereference again.
For example
In a 2D array of size 5*6, we create an array of pointers of length 5, and each index points to an array of size 6. Consider the base address of the array of pointers to be ‘ptr’. To access the data present at index (4, 2), first we do *(ptr+4), this will return us the value at the 4th index of the array of pointers. This value is in turn the address of an array. Then to this address, we add 2 to reach the 2nd column and dereference again. Hence, * ( * (ptr+4)+2) gives us the required answer. Again, [] operators can do the same tasks for us, but to understand how things are working, the pointer way is necessary to understand.
Code:
Output:
Conclusion:
In this article, we discussed:
- What an array of pointers is in C++
- Difference between an array of pointers and a pointer to an array
- How to create an array of pointers in C++ at compile time
- How to create an array of any data type dynamically
- Relevance of pointers in dynamic memory allocation
- Accessing elements of an array using pointer arithmetic
- Importance of array of pointers in 2 dimensional dynamic array
- Accessing elements of a 2-dimensional array using pointers
- Gone through examples to understand the working of an array of pointers in C++