Array Size C++
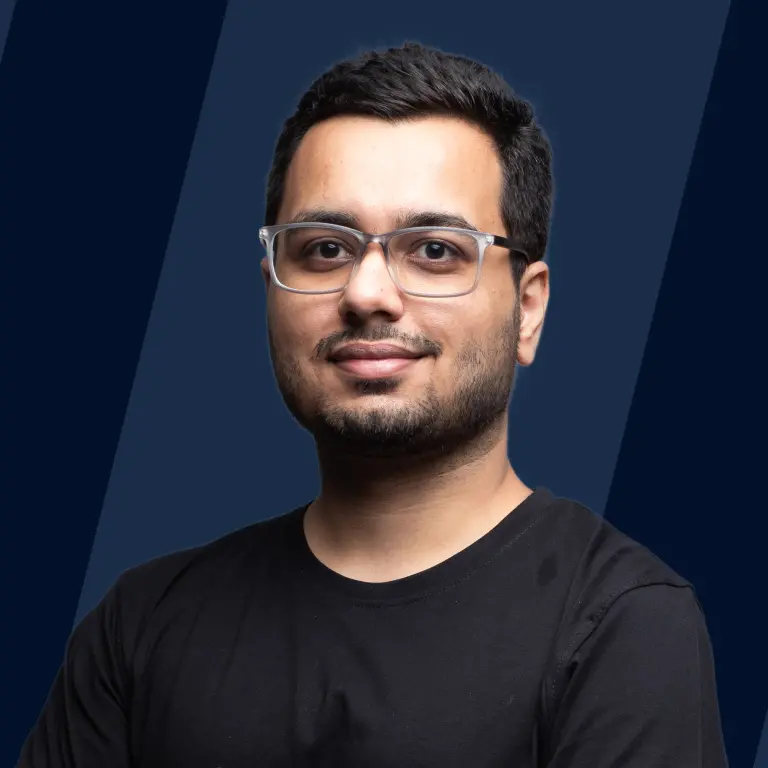
Overview
The size() function of the array class in C++ determines the list container's size or the number of elements the container/array can contain. Compared to C-style arrays, C++ array classes are typically more effective and lightweight. An improved replacement for C-style arrays has been made available by introducing the array class in C++11.
Syntax of Array Size C++ Function
Syntax for using the array class size() function:
Parameters of Array Size C++ Function
Array size C++ function does not have any parameters and arguments.
Return Value of Array size C++ function
array.size() returns the number of elements/items an array container can store. The return value is of type unsigned long.
Exceptions of Array Size C++ Function
- It guarantees that no exceptions will be made.
- size() function expects arguments, so if an argument is passed in the size() function, it will throw an error.
How does the Array Size C++ Function Work?
Array size C++ function is defined in the array class in the C++ libraries. It is an improved replacement for C-style arrays made available by introducing the array class in C++11.
Array size() C++ function works only with the arrays defined using the syntax of std::array declaration (Standard Template Library). It can be used in the C++ program by including the line #include<array>.
Syntax to declare an STL array:
The size() method is defined in the std::array class along with many other methods like sort(), swap(), empty(), etc. size() function takes no arguments and returns the size of the array, i.e., how many elements the array can store.
Example of Array Size C++ Function
Let's see how we declare, initialize, and print the size of the array in the output using the below C++ program:
Example C++ Program:
Output:
Explanation:
In the above C++ program, we have declared four different arrays using the array STL class. The first array is empty, and the rest are of size 6 with a different number of elements. We have printed the size of the arrays using the Array size C++ function in the output. Size represents the elements an array can store.
Alternatives to Array Size C++ Function
There are many alternative methods to calculate the size of the array. These alternative methods are listed below:
- Using sizeof() function,
- Using begin() and end() method, and
- Counting array elements using a range-based for loop.
Let's see all the alternative methods of implementation using C++ programs below:
1. Using sizeof() function:
Output:
Explanation:
sizeof(<argument>) function returns the amount of memory the <argument> object is taking. sizeof(arr) returns the total memory arr is taking, i.e., 6 (array size) * 4 (int) = 24 bytes and sizeof(arr[0]), i.e., one element of the array is 4 bytes. sizeof(arr)/sizeof(arr[0]) = (24 ÷ 4) = 6 returns the size of the array.
2. Using begin() and end() method:
Output:
Explanation:
begin() and end() methods of the standard template library are used to get iterators pointing to the first and last element of the array, respectively. end(arr) - begin(arr) will give us the length of the array.
3. Counting array elements using range-based for loop:
Output:
Explanation:
We can use a range-based for loop and iterate over every element with an increment in the count variable. At the end of the range-based for loop count variable will contain the size of the array.
Conclusion
- size() function of array class in C++ is used to determine the list/container size.
- Array size() C++ function works only with the arrays defined using the std::array declaration syntax.
- Array size C++ function does not take any arguments.
- array.size() returns the number of elements an array can store.
- Many other methods like sizeof(), begin(), and end(), and range-based for loop can be used as an alternative for finding the size of the array.