Array to Arraylist Java
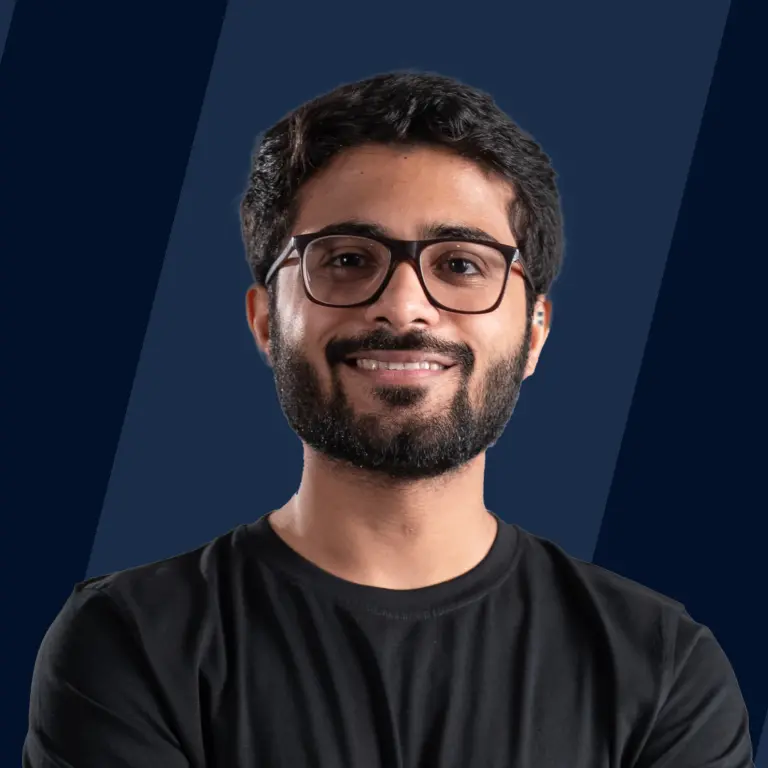
Overview
Arrays are a collection of similar types of data stored in a contiguous location. They are very simple and provide us with basic functionalities. However, their size is fixed and this adds to a major disadvantage that the arrays have. This leads to difficulty in providing the very basic operations such as adding an element in the beginning if we are out of size.
However, the Collections framework in Java has introduced us to many data structures such as ArrayList, HashSet, and HashMap which overcome the disadvantage of arrays. They have a dynamic size that can grow and shrink as per the demand of the user.
We will be talking about the conversion of Arrays to ArrayLists in this article. Since the ArrayList's constructor can accept any Collection, therefore, we have been creative enough to pass different Collections into it and fulfill our purpose to convert an array into an Arraylist.
Note :
A Collection in Java is any group of individual objects that can be grouped in a single unit. Java provides the Collection Framework that provides a set of classes and interfaces to implement common data structures.
Conversion of Array to ArrayList in Java
We convert an array to an ArrayList because of the major disadvantage of the array, which is, its fixed size. We cannot insert elements in arrays during runtime because of their fixed and predefined size.
The ArrayList, on the other hand, provides a dynamic size and can add elements during runtime also. Therefore, we convert the arrays into an ArrayList to provide more flexibility to our data structures.
There are various methods of converting an array into an arrayList in Java such as Arrays.asList() method, Collections.addAll() method, Lists.newArrayLists() method, add() method in ArrayList and many more.
All these methods are discussed in detail in the subsequent subtopics.
Using Arrays.asList() Method
The Arrays class has a lot of different methods which are used to serve different purposes. One such method is asList() which is used to convert an array to an ArrayList.
This method takes an array as an argument and returns a fixed-size list that is serializable and implements Random Access. Since the list is of fixed size, therefore, we cannot add more elements to it but we can replace the elements in the list using the method set(index, newElement).
Let us see how will be using this asList() method of the Arrays class to convert an array to an ArrayList.
Output :
What if we add more elements to the converted list ?
If we try to add more elements to the converted list, we will get an
UnsupportedOperationException error, since the list is of fixed size and it cannot be extended.
Let us see the same with the help of a program to convert an array to arraylist in Java.
Output :
Using new ArrayList<>(Arrays.asList())
Since the previous approach does not let us add more elements to the converted list, therefore, in such cases we create a new ArrayList and pass the converted list to the constructor of the new ArrayList using new ArrayList<>(Arrays.asList()).
Let us see this with the help of a program to convert an array to arraylist.
Output :
This method is considered the most popular and used approach to convert an array into an ArrayList.
Using new ArrayList<>(List.of())
The List.of() method in Java is used to create immutable lists. However, this method also provides you the functionality of skipping the initialization of an array itself rather it passes the array elements directly to the ArrayList constructor inside the List.of() method.
Let us see the use of the List.of() method to convert an array to an ArrayList.
Output :
Note :
Mutable Lists are those whose value can be changed after initialization. However, the List.of() method creates an immutable list.
Using Collections.addAll() Method
The Collections Framework in Java provides us with many useful methods to achieve our desired purpose. One such method in Collections Framework is addAll() which will solve our purpose to convert an array to an ArrayList.
This method is used to append the elements at the end of the collection. It takes two parameters, a collection c into which the elements are inserted and an array arr that contains the elements to be inserted into the collection c.
Let us see their usage with the help of a program :
Output :
However, we can also add more elements to the converted list, if a collection does not support the add operation then it returns an error stating UnsupportedOperationException.
Also, if the array contains any such element that cannot be added to the collection c then it returns the IllegalArgumentException error.
Adding Null to the List
If the collection c in which the elements of the array need to be stored is null then it will throw the NullpointerException error.
Let us see it with the help of a program to covert an array to an arraylist :
Collectors.toList()
This toList() method of the Collectors class is a static method and returns a Collector Interface that collects the input array onto a new list.
If you are working with Streams rather than Collections, then the toList() method can be used to collect these streams and pack them into a list.
Let us see how can we perform the same with the help of a program to convert an array to an arraylist.
Output :
Collectors.toCollection()
The toCollection() method is used to create a Collection using the Collector Interface. This method returns a Collector that gathers the input elements into a collection in the same order in which they were passed.
It takes a parameter, Collection c, in which the input elements are gathered. Similar to the toList() method, the toCollection() method of the Collectors Interface can also be used to collect the streams from different collections and pack them into a list.
Let us see how to use the toCollection() method to convert an array to an arrayList.
Output :
Lists.newArrayList()
Google’s guava project introduced the Lists class which provides many useful methods. One such method is newArrayList() which takes an array as a parameter and returns an ArrayList. However, it returns a mutable ArrayList that contains elements from an array or an iterator.
However, in case a mutable arrayList is not required, then we can use the ImmutableList.copyOf() method instead.
Let us see its usage with the help of a program to convert an array to an arraylist.
Output :
However, in this approach, you can also skip the type of the ArrayList while initializing it.
Using Manual Method to Convert Array Using add() Method
This is a manual method of converting an array to an ArrayList using the add() method in Java. This method is used when you do not want to use the in-built methods in Java.
In this method, first, the array and the ArrayList are declared and initialized. After which, each array element is added to an ArrayList using a loop.
Let us see the implementation of this method :
Output :
Conclusion
- The new ArrayList<>(Arrays.asList()) method is the most popular and used approach to convert an array into an ArrayList in java.
- The Arrays.asList() method converts an array into an ArrayList but returns a fixed-size list in which more elements cannot be added. Therefore, this advantage of the asList() method is overcome by creating a new ArrayList and passing the converted list as an argument to it.
- The Collection.addAll() method is also used for the same conversion. However, it takes the collection as well as the input array as the parameters.
- The guava project of google also provides a direct method Lists.newArrayList() that converts the array into an ArrayList by passing the array as a parameter to the function.
- If you do not want to use the in-built methods of Java, you can also use the manual method using the add() method to perform the conversion.