ArrayList Vs Vector in Java
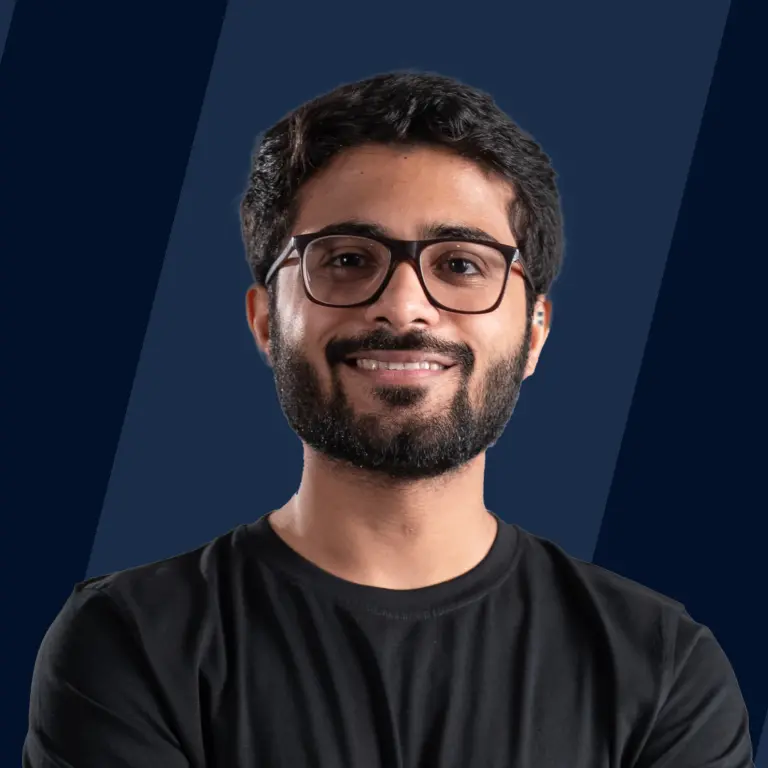
Overview
This tutorial will focus on the differences between the ArrayList and Vector classes. Both of them implement the java.util.List interface and are a part of the Java Collections Framework.
The implementations of these classes, however, varied greatly from one another. The dynamic arrays (resizable arrays), ArrayList, and Vectors implement the list interface. Let's talk about some of the key distinctions between ArrayList and Vectors.
ArrayList
The ArrayList class is part of the Java.util package and is an important part of the collection system. Because ArrayList can alter its size, it is often called a dynamic array. An advantage of memory management is adding and removing elements from an ArrayList, depending on the need.
Vector
The vector in Java is comparable to a dynamic array that can change in size. The list interface is implemented using it. One of a vector's greatest advantages is that there is no size restriction, allowing us to store unlimited elements. The ArrayList and Vectors are quite comparable. However, the Vectors contain many old methods that are not included in the collection framework.
ArrayList Vs Vector
Feature | ArrayList | Vector |
---|---|---|
1. Synchronization | ArrayList is not synchronized. | Vector is synchronized, making it thread-safe. |
2. Capacity Increment | ArrayList increases capacity by 50% | Vector doubles its array size when exceeding capacity. |
3. Legacy Status | ArrayList is not a legacy class. | Vector is considered a legacy class, introduced earlier in Java's history. |
4. Thread Safety | ArrayList is fast as it's non-synchronized, suitable for single-threaded environments. | Vector is slower due to synchronization, making it safer for multithreaded environments but potentially slower for single-threaded operations. |
5. Element Traversal | ArrayList uses the Iterator interface for element traversal, providing flexibility and compatibility. | Vector can use either the Iterator interface or the older Enumeration interface for element traversal. |
6. Performance | ArrayList generally offers higher performance in single-threaded scenarios. | Vector may exhibit lower performance due to synchronization overhead in multithreaded scenarios. |
7. Thread Support | ArrayList allows multiple threads but requires external synchronization for thread safety. | Vector inherently supports thread safety for operations in a multithreaded environment. |
Comparative Analysis between ArrayList and Vector in Java
Synchronization
Unlike ArrayList, which is not synchronized and allows several threads to work on it concurrently, Vector is synchronized, meaning only one thread can access the code at a time. In a multithreading system, for instance, if one thread executes an add operation, another thread may perform a removal action. When many threads access an array list simultaneously, we must synchronize the code block that updates the list's structure or permits simple element changes. The term "structural modification" refers to adding or removing one or more list elements. An existing element's value being changed is not a structural adjustment.
Performance
ArrayList is faster. In contrast to vector operations, which execute more slowly because they are synchronized (thread-safe). If one thread works on a vector, it has obtained a lock, preventing any other thread from accessing it until it is released.
Data Growth
ArrayList and Vector dynamically expand and contract to ensure optimal storage utilization, although they do it in distinct ways. If there are more elements than the array list can hold, the array size is increased by ArrayList by 50% and Vector by 100%, thus doubling the array size.
Traversal
While ArrayList can only use an Iterator, Vector can use Enumeration and Iterator to traverse its elements.
Applications
Programmers typically like ArrayList over Vector because ArrayList may be explicitly synchronized using Collections.synchronizedList.
How to Get a Synchronized Version of ArrayList Object?
By default, an ArrayList object is non-synchronized, but we may get a synchronized version by utilizing the Synchronised List() function of the collection class.
Note: ArrayList is preferable when there is no specific requirement to use vector.
Java Program to illustrate the Use of ArrayList and Vector in Java
Output:
Which One to Choose?
In contrast to Vectors, ArrayList is not thread-safe and is not synchronized. A Vector can only have one thread use its methods, which is slightly inefficient but useful when safety is an issue. As a result, ArrayList is the obvious choice in single-threaded situations, whereas vectors are frequently better in multi-threaded situations. Vector has an advantage because we can specify the increment amount in vectors if we don't know how much data we will have but know the rate at which it grows. Faster and newer is ArrayList. If neither of them is explicitly required, we prefer using ArrayList over vector.
FAQs
Q. When should I use ArrayList over Vector?
A. Use ArrayList when you don't require thread safety or are working in a single-threaded environment. It's generally more efficient due to its lack of synchronization overhead.
Q. When should I use Vector over ArrayList?
A. Use Vector when you need thread safety, especially in multi-threaded applications. Vector ensures that multiple threads can safely manipulate the list simultaneously without causing data corruption.
Q. What is the performance impact of synchronization in Vector?
A. Synchronization in Vector can lead to reduced performance in comparison to ArrayList in single-threaded scenarios. In multi-threaded scenarios where synchronization is necessary, Vector is a better choice.
Q. Can I make an ArrayList thread-safe like Vector?
A. Yes, you can make an ArrayList thread-safe by using synchronized blocks or by wrapping it with the Collections.synchronizedList() method. However, this might impact performance similarly to a Vector.
Conclusion
In conclusion, when choosing between ArrayList and Vector in Java, consider the following:
- ArrayList is not synchronized, making it more efficient for single-threaded applications, while Vector is synchronized, ensuring thread safety in multi-threaded environments.
- ArrayList should be preferred for better performance in most cases due to its non-synchronized nature, while Vector can introduce synchronization overhead.
- Vector is legacy and less commonly used in modern Java development, while ArrayList is a more popular choice for dynamic arrays.
- Use ArrayList when thread safety is not a primary concern, and Vector only when synchronized access is required.
- In contemporary Java programming, ArrayList is typically the preferred choice for its flexibility and performance advantages.