Arrays aslist Java
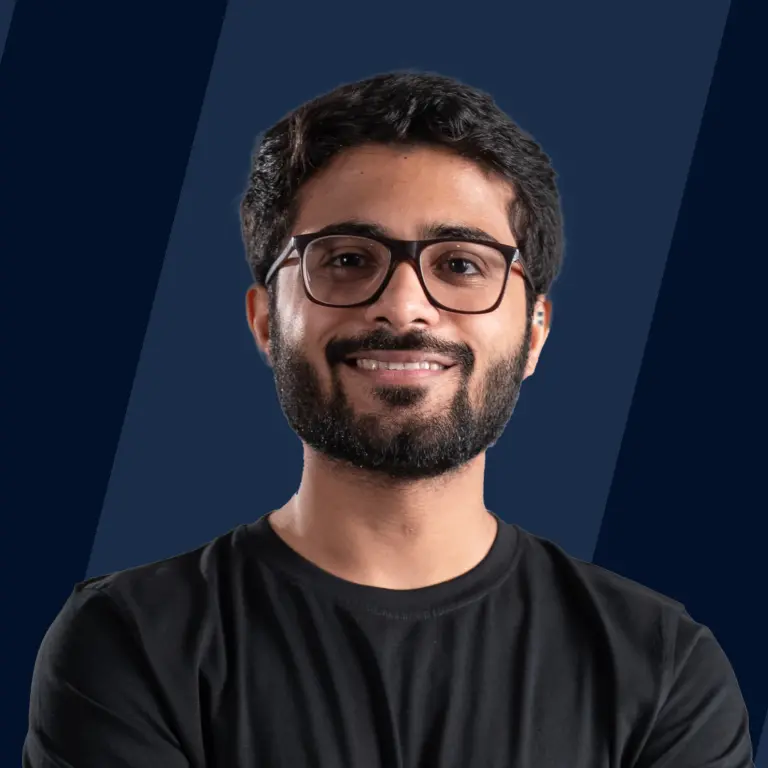
Overview
Arrays.asList() in Java, is used to turn an Array into a List provided by java.util.Arrays as a wrapper. This method takes an array as a parameter and returns a list.
This function serves as a bridge between Array-based APIs and Collections. Since the Java platform API was developed before the collection framework came into play, we might need a translation between the modern collections and traditional arrays. The asList() method allows us to do that.
Syntax of Arrays asList() in Java
where,
- public : access mode
- List : return object type
- asList : function name
- a : array type, passed as a paramter here, should be a Wrapper class
Parameters of Arrays asList() in Java
1. Array as Argument
The string array is converted to a list using the asList() method
2. Elements as Arguments
We can pass multiple elements, separated by a comma, to the asList() method as arguments.
Output :
Arrays.asList() method is called and elements as arguments as passed, which then returns a List of type depending upon the type of elements that we passed to it
3. With Class Objects
Elements of a user-defined class type can also be passed as arguments to the asList() method.
Output :
Objects as arguments are passed in the Arrays.asList() method and a List of input types of arguments are returned.
Return Value of Arrays asList() in Java
Arrays.asList() returns a fixed-size list that is backed by a specified array.
The hidden implementation using java.util.Arrays.asList() gives us a structurally immutable list object, implying that all the methods we have for changing a list structure are disabled by throwing exceptions at the call. In other words, it's not possible to add or remove elements from the list and, the size of the array may not change except for set() to change elements within that list.
The purpose of this method is to allow us to quickly create a fixed "list" of objects.
We can say that the Arrays.asList method "wraps" an array and lets it "look like" a List rather than creating a new array.
asList() as a Bridge between Arrays and Collections
The Arrays.asList() method helps us to "bridge" between the Arrays and Collections, since an array is not a collection, this method allows us to pass data to a method that is expecting a collection.
We can create other collections from an array. If we want to create a set containing the elements from the array, we can do :
Using the Arrays.asList() method, however, this can be done more conveniently :
Output:
After an array is created, it's converted into a list and passed into a HashSet constructor which accepts other collections. Elements of the set are printed in sorted order.
Similarly, if we have an array and want to create an ArrayList containing the elements from the array, we could do that.
A new ArrayList is created whose wrapper, despite being created using Arrays.asList(), is used only during the construction of the new ArrayList and is garbage-collected afterward. It provides us with an independent copy of the original Array. (We'll read more about this further in this article, so keep reading :))
Internal Working of Arrays.asList() Method in Java
Let's understand the internal working of the Arrays.asList() method in Java using the following example :
Output :
Here asList() method takes “teamMembers” as an array and after creating a wrapper it’s implemented using List<String> which gets our list from the original array teamMembers
A single wrapper object is created. When we implement any operation like changing a list wrapper or overwriting an element, changes are also reflected upon the original array. List operations like add() or remove() are not allowed in this immutable list, however methods like get() and set() can be used.
We'll learn more about this further in the article :
Note :
that the array type used for asList() method should be a Wrapper class like Integer, Float, String etc and not Primitive data types like int, float, string etc.
In this case, there is no Autoboxing and so when a primitive data type like int a[] is passed the function int a[] is identified as an object and List<int a[]> is returned instead of List<Integer> which gives an error in various collection functions.
To know more about Wrapper class, primitive data types, and Autoboxing refer to Wrapper Classes in Java article.
More Examples
1. Get List from String Array
Let's see how we can convert an Array of Strings to a fixed-size List using the Arrays.asList() method.
An array is first created of type String which is of a wrapper class We then get the list view of that array of String type by passing an array as a parameter to the Arrays.asList() method, the list is then printed.
Ouput :
2. Get List from Integer Array
Let's see how we can convert an Array of Integers to a fixed-size List using the Arrays.asList() method.
An array is first created of type Integer which is of a wrapper class. We then get the list view of that array of Integer types by passing the array as a parameter to the Arrays.asList() method. The list is then printed.
Output:
3. Get a List of Several Types of Arrays
We've talked earlier about how for the asList() method the array type that's supposed to be used should be a Wrapper class and not a primtive data type, just like String and Integer examples, let's see how other arrays types of wrapper class work with our asList() method.
Arrays are created of type Boolean, Character, Byte, Double, Long, Float, Short, which are of a wrapper class, we then get the list view of that array of these types by passing an array as a parameter to Arrays.asList() method which is then printed.
Ouput :
4. asList() Method Which Returns Fixed-size List and Throws UnsupportedOperationException
When the Arrays.asList() method is called on an array, the returned object is not an ArrayList. It is not copied or created; it is just a view of the same array with get() and set() methods to access the array. All the methods like add() or remove() that change the size of an array would throw an UnsupportedOperationException error (we'll learn more about this in the article).
This is why the Java program, despite being compiled successfully, throws a Runtime Exception error because a List is returned as a result of Arrays.asList(), where adding/deletion is permissible, but since the returned data structure is a fixed-sized array, we get a runtime exception.
ArrayList is a resizable array implementation of the List interface.
Let's see how modifications made to the single items of the List will be reflected in our original array.
Now, let's see what happens if we modify the first element of our List :
We see that our original array was modified too. Now our List and Array both contain the same elements in the same order.
Let's now try to insert a new element to our List :
We get the following error :
Thus, adding or removing elements to/from the List will throw java.lang.UnsupportedOperationException.
Why does it happen? Why do we need an immutable list in the first place?
Often it's useful to have a List that we can iterate and read. An immutable list protects us, in the same way as the final keyword stops us from mistakenly modifying a variable.
Creating an Independent List from an Array with Arrays.asList()
The java.util.Arrays is a utility class with a bunch of static methods to operate on a given array. asList() is one such static method that takes an input array and returns an object of java.util.Arrays.ArrayList, which is a static nested class that extends AbstractList<E> and implements the List interface.
A List wrapper returned by the Arrays.asList() method is present in the java.util.Arrays.ArrayList and not java.util.ArrayList, which is why it refers to the original array, thereby any changes made in the List would be reflected upon the original array as well.
How can we solve this issue if we need to create a list from an array with list functionality?
Similar to the Arrays.asList() method, we can use:
when we need to create a List out of an array.
This, unlike the previous example, is an independent copy of the array, meaning that modifying the new list won't affect the original array, and we have all the capabilities of a regular ArrayList, like adding and removing elements:
Now let's modify the first element of stringList:
And now, let's see what happened with our original array:
We see that our original array remains untouched. Let's see why this happens:
In java.util.ArrayList, we are provided with the following overloaded constructors:
A new dynamic array is created when the Arrays.asList() returned object is passed to the public ArrayList(Collection<? extends E> c) constructor. This dynamic array is a result of a shallow copy of the original array.
- A dynamic array would grow automatically whenever, despite having no more space, we insert more elements, causing its capacity to double in size.
- A shallow copy of an object copies the properties that share the same references as that of the source object and does not create a new set of the same objects as in the source object.
Conclusion
- Arrays.asList() method is used to turn an Array into a List provided by java.util.Arrays as a wrapper.
- Arrays.asList() returns a fixed-size list that is backed by a specified array.
- Arrays.asList() method helps us to "bridge" between Arrays and Collections since it allows us to pass data to a method that is expecting a collection.
- Adding or removing elements to/from the List will throw a java.lang.UnsupportedOperationException error.
- The array type used for the asList() method should be a Wrapper class and not a Primitive data type.