What is the Use of Arrow Operator -> in C++?
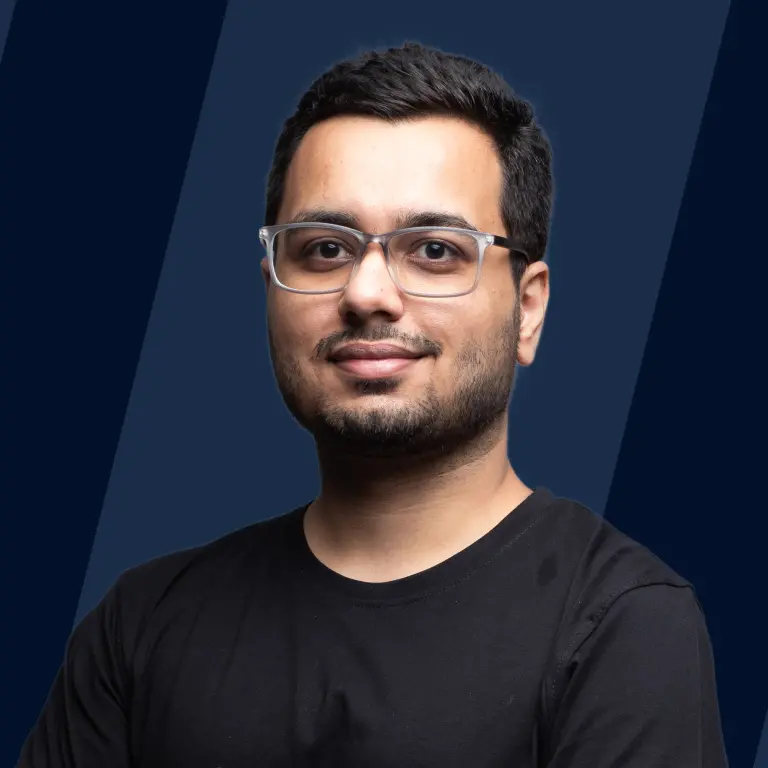
Before learning about the Arrow Operator -> in C++, let's learn about the basics of Operators in C++.
An operator is a symbol that is used to give instructions to the compiler to perform a particular operation on given operands. Each operator has its pre-defined operation. Operators are the basic building blocks of any programming language. There are different kinds of operators in C++, such as Assignment Operators, Arithmetic Operators, Relational Operators, Logical Operators, Bitwise Operators, and many others operators.
Now, let's understand what is -> in C++ (Arrow Operator).
Arrow operator (->) in C++ also known as Class Member Access Operator is a combination of two different operators that is Minus operator (-) and greater than operator (>). It is used to access the public members of a class, structure, or members of union with the help of a pointer variable.
There is a .(dot) operator in C++ that is also used to access the members of a class. But .(dot operator) accesses the member or variable directly means without using the pointers whereas instead of accessing members directly the arrow operator(->) in C++ uses a pointer to access them. So the advantage of the -> operator is that it can deal with both pointer and non-pointer access but the dot(.) operator can only be used to access the members of a class.
Arrow (->) operator in C++ represents the same meaning which is done by the (*) asterisk operator and dot(.) operator. Arrow operator can be written in a different way which is using the combination of two operators *.. For example, (*s).element is the same as s->element. The brackets (parenthesis) used while using the combination of (*.) operators show that the precedence of the dot(.) operator is more than *.
Let's understand the use of the Arrow operator -> in C++ using some practical programming examples.
Syntax
Let's first take a look at the syntax for using the arrow operator in C++ for accessing the members of a class, structure, etc.
Here, we have used the arrow operator to access the class member variable represented by class_member_name through the object pointer which is represented by pointer.
Explanation
Let's implement a simple program to explain and demonstrate the use of the -> operator in C++. In the below example, we have declared a structure Person which contains two member variables. The first one is the name variable which is a character array that stores the name of the Person and the other one is the age variable which is of integer type.
Code
Output
Explanation
In the above example, after creating classes and objects we have called the main() method. In the main() method, we have created an object info of structure type that is Person type. Then we used the arrow operator (->) to assign the 17 value to the member variable age. After assigning the value to the member, we then used the arrow operator (->) to access the member and print its value as we can see in the output.
Examples of Arrow Operators -> in C++
We will implement several examples of the usage of the -> operator in C++ to understand about the arrow operator in more depth.
Example 1
In this example, we will demonstrate the usage of the arrow operator -> in C++ with classes. Here, member functions of the class are accessed by the pointer object using the -> operator.
Code
Output
Explanation
In the above example, we have first created a Student class that contains some private variables and public functions. The first function which is percentage calculates the total percentage by using the marks stored in the total_marks variable. Now, as we can see the total_marks variable is private so we can use the this keyword to refer to the private variable. Now for referring to the current object's private variable, the this keyword uses the arrow operator.
Example 2
In this example, we will demonstrate the usage of the arrow operator -> in C++ with unions. Here, member variables of the union are accessed by the pointer object using the -> operator.
Code
Output
Explanation
In this example, we created a union called Studentwith some variables. In the main function, the object of the union student is created and initialized with a NULL value. Here, we have dynamically allocated memory to the created union object. Since the object contains two variables we can refer to those variables using the arrow operator. So we have used the object name along with the arrow operator to refer to the desired union variable.
Example 3
In this example, we will demonstrate the usage of the arrow operator -> in C++ with struct. Here, member variables of the structure are accessed by the pointer object using the -> operator.
Code
Output
Explanation
In this example, we have created a structure called Employee having a variable name. In the main function, we have the object of the student structure and initialized them with the NULL value. Now, we have dynamically allocated the memory to the union object. Since the object contains one variable we can refer to that variable using the arrow operator. So we have used the object name along with the arrow operator to refer to the desired struct variable.
Asterisk and Dot Operator Instead of Arrow Operator
Let's see an example to understand the usage of the arrow operator and combination of (*) and (.) operators which is a kind of similar way to access the members of a class.
Example
Code
Output
Explanation
In this example, we have created a class called Combihas a variable and member functions. In the main function, we have created the object of the Combi class. Now, we have dynamically allocated the memory to the object s using the new keyword. Since we have used the new keyword the object is created and a reference to the object(pointer) is returned. We can either use the arrow operator or the Asterisk(*) and dot(.) operator to refer to the data members and member functions. Here, first the *s is resolved and then the dot operator will help us to locate the actual data member and member functions.
Now, we will use the arrow operator to implement the same program. This will also give the same output.
Code
Output
Explanation
In this example, we have created a class called Combi that has a variable and member functions. In the main function, we have created the object of the Combi class. Now, we have dynamically allocated the memory to the object s using the new keyword. Since we have used the new keyword the object is created and a reference to the object(pointer) is returned. Here, we have used the arrow operator to refer to the desired class variable or member function.
Different Operators in C++
Operators are the basic building block of any programming language. An operator is a symbol that will be operated on a variable or a constant value. They are used to give instructions to the compiler to perform a particular operation. Each operator has its pre-defined operation.
There exists a different kinds of operators in C++ which are listed below:
- C++ Arithmetic Operators
- Arithmetic operators are used to perform arithmetic operations on the operands. These operators include + (addition), - (Subtraction), * (Multiplication), % (Modulo), and / (Division).
- C++ Relational Operators
- Relational Operators are used to compare the values of two operands. These operators include == (Is Equal To), != (Not Equal To), > (Greater Than), < (Less Than), >= (Greater Than or Equal To), <= (Less Than or Equal To).
- C++ Logical Operators
- Logical Operators are used to find whether an expression is true or not. These operators include && (Logical AND), || (Logical OR), and ! (Logical NOT).
- C++ Bitwise Operators
- Bitwise operators are used to performing operations on integers at the bit level. These operators include & (Bitwise AND), | (Bitwise OR), ^ (Bitwise XOR), ~ (Bitwise NOT), << (Bitwise Shift Left), >> (Bitwise Shift Right).
- C++ Assignment Operators
- Assignment operators are used for assigning values to a variable. These operators include =, +=, -=, *=, /=, %=.
- and other operators.
To learn more about Operators in C++, refer to the article Operators in C++
Conclusion
- An operator is a symbol that is used to give instructions to the compiler to perform a particular operation on operands.
- Arrow operator (->) in C++ also known as Class Member Access Operator is a combination of two different operators that is Minus operator (-) and greater than operator (>).
- It is used to access the members of a class, structure, or members of union with the help of a pointer variable.
- There is a .(dot) operator in C++ that is also used to access the members of a class.
- . (dot operator) accesses the member or variable directly Whereas instead of accessing the members directly the arrow operator(->) in C++ uses a pointer to access them.
- Arrow (->) operator in C++ represents the same meaning which is done by the (*) asterisk operator and dot(.) operator.
- The combination of (*) and the (.) operator can also be used to access the members of a class similarly.