ASCII in Java
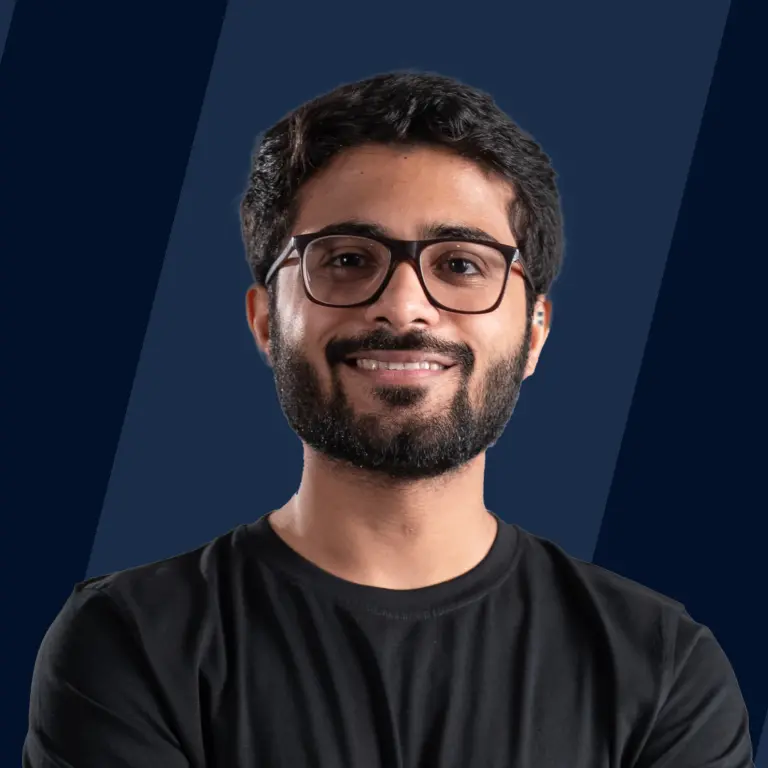
Overview
ASCII (American Standard Code for Information Interchange) is a character encoding system used to represent text in computers and other devices. It assigns a unique numerical value to each character, including letters, numbers, punctuation marks, and other symbols. In the ASCII system, each character is represented by a 7-bit binary code.
It can only represent a maximum of 128 characters because it is a seven-bit code**. There are 95 printable characters now defined by it, including 26 upper cases (A to Z), 26 lower cases, 10 numbers (0 to 9), and 33 special characters such as mathematical symbols, punctuation marks, and space characters.
In Java, ASCII is used as the default character encoding for text data.
How to Print ASCII in Java?
ASCII (American Standard Code for Information Interchange) is a system that assigns unique numerical values to characters so that computers can store and manipulate them. The assigned numerical values are represented in binary form for use by electrical equipment, since it is not possible to use or store the original character form.
There are four methods available in Java to print the ASCII value. Each method is briefly explained below, along with an example of how to implement it:
- Brute force technique
- Type-casting method
- Format specifier method
- Byte class method [Most Optimal]
Method 1 - Using Brute Force Method
Simply assign the character to a new integer-type variable to determine the character's ASCII value. Java automatically inserts the character's ASCII value into the new int variable.
We will determine the ASCII value of the character entered by the user in this example.
Code:
Output
Code Explanation
- We have created an object of the Scanner class to get input from the user.
- We then stored the character entered by the user in a char type variable and assigned the char value to an int type variable.
- Upon assigning the ascii value of the character gets stored in the int variable.
Method 2 - Using the Type-casting Method
In Java, type-casting is a technique for changing a variable's datatype. To use type casting to get the ASCII value of a character in Java, you can follow these steps:
- Declare a character variable and assign a value to it.
- Type cast the character variable to an integer using (int).
- Print the ASCII value using System.out.println().
Code:
Output
Method 3 - Using the format() Method
The format() method in Java is used to format a string using a specified format string and arguments.
The sprintf() function in the c programming language and the format() method in python achieve similar functionality.
In this method, a format specifier is used to produce the ASCII value of the given character. By designating the character to be an int, we were able to save the value of the supplied character inside a format specifier. As a result, the format specifier contains the character's ASCII value.
In this example, we are going to learn how to get the ASCII value of a character using a format specifier.
Output
Code Explanation
- In the above code, we have used a format specifier to get the ASCII value of a character.
- First, we have created an object of Formatter and converted the character to an integer using the %d format specifier.
Method 4 - Using getBytes() Method
This is the most optimal way to get the ASCII value of a character
- Initialize the character as a string.
- Create an array of type bytes using getBytes() method.
- Print the element at the 0th index of the byte array.
This technique is typically used to translate an entire string to its ASCII values. The try-catch is provided for characters that violate the encoding exception.
In this example, we are going to get the ASCII value of a character using the Byte class method which happens to be the most optimal technique.
Output
Code Explanation
- In the above code, we have created a byte array from a single character string using the ASCII encoding and prints the ASCII value of that character.
- In the above Java code, the getBytes() method is used to encode a string into a sequence of bytes using a specific character set.
- In this case, the getBytes() method takes the "US-ASCII" character set as an argument, which means that the string will be encoded using the US-ASCII encoding standard.
- If an exception occurs due to unsupported encoding, a message is printed to indicate the issue.
ASCII Table
In total, there are 256 ASCII characters, and can be broadly divided into three categories:
- ASCII control characters (0-31 and 127)
- ASCII printable characters (32-126) (most commonly referred)
- Extended ASCII characters (128-255)
Character | Character Name | ASCII code | Character | Character Name | ASCII code | Character | Character Name | ASCII code | ||
---|---|---|---|---|---|---|---|---|---|---|
! | Exclamation point | 33 | A | Uppercase a | 65 | a | Lowercase a | 97 | ||
“ | Double quotation | 34 | B | Uppercase b | 66 | b | Lowercase b | 98 | ||
# | Number sign | 35 | C | Uppercase c | 67 | c | Lowercase c | 99 | ||
$ | Dollar sign | 36 | D | Uppercase d | 68 | d | Lowercase d | 100 | ||
% | Percent sign | 37 | E | Uppercase e | 69 | e | Lowercase e | 101 | ||
& | ampersand | 38 | F | Uppercase f | 70 | f | Lowercase f | 102 | ||
‘ | apostrophe | 39 | G | Uppercase g | 71 | g | Lowercase g | 103 | ||
( | Left parenthesis | 40 | H | Uppercase h | 72 | h | Lowercase h | 104 | ||
) | Right parenthesis | 41 | I | Uppercase i | 73 | i | Lowercase i | 105 | ||
* | asterisk | 42 | J | Uppercase j | 74 | j | Lowercase j | 106 | ||
+ | Plus sign | 43 | K | Uppercase k | 75 | k | Lowercase k | 107 | ||
, | comma | 44 | L | Uppercase l | 76 | l | Lowercase l | 108 | ||
– | hyphen | 45 | M | Uppercase m | 77 | m | Lowercase m | 109 | ||
. | period | 46 | N | Uppercase n | 78 | n | Lowercase n | 110 | ||
/ | slash | 47 | O | Uppercase o | 79 | o | Lowercase o | 111 | ||
0 | zero | 48 | P | Uppercase p | 80 | p | Lowercase p | 112 | ||
1 | one | 49 | Q | Uppercase q | 81 | q | Lowercase q | 113 | ||
2 | two | 50 | R | Uppercase r | 82 | r | Lowercase r | 114 | ||
3 | three | 51 | S | uppercases | 83 | s | Lowercase s | 115 | ||
4 | four | 52 | T | Uppercase t | 84 | t | Lowercase t | 116 | ||
5 | five | 53 | U | Uppercase u | 85 | u | Lowercase u | 117 | ||
6 | six | 54 | V | Uppercase v | 86 | v | Lowercase v | 118 | ||
7 | seven | 55 | W | Uppercase w | 87 | w | Lowercase w | 119 | ||
8 | eight | 56 | X | Uppercase x | 88 | x | Lowercase x | 120 | ||
9 | nine | 57 | Y | Uppercase y | 89 | y | Lowercase y | 121 | ||
: | colon | 58 | Z | Uppercase z | 90 | z | Lowercase z | 122 | ||
; | semi-colon | 59 | [ | Left square bracket | 91 | { | Left curly brace | 123 | ||
< | Less-than sign | 60 | \ | backslash | 92 | | | Vertical bar | 124 | ||
= | Equals sign | 61 | ] | Right square bracket | 93 | } | Right curly brace | 125 | ||
> | Greater-than sign | 62 | ^ | caret | 94 | ~ | tilde | 126 | ||
? | Question mark | 63 | _ | underscore | 95 | |||||
@ | At sign | 64 | ` | Grave accent | 96 |
Conclusion
- ASCII stands for American Standard Code for Information Interchange and it's a character encoding system which assigns a unique numerical value to each character, including letters, numbers, punctuation marks, and other symbols.
- Different letters and symbols are assigned a distinct numerical value in ASCII so that computers can store and manipulate them. The hardware always operates with the binary value of the assigned ASCII number. Since doing so in the original form is impossible.
- There are four different ways to output an ASCII value in java for a particular character.
- Using the brute force Method
- Using the type-casting Method
- Using the format specifier Method
- Using Byte class Method
- We can use the format() method in Java to format a string using a specified format string and arguments. The sprintf() function in the c programming language and the format() method in Python achieve similar functionality
- The Byte class method is the most optimal method. We use the Byte class's static method to get the ASCII value of a character. This method is more efficient than the other three methods.