C++ Program to Print ASCII Value of 1 to 9
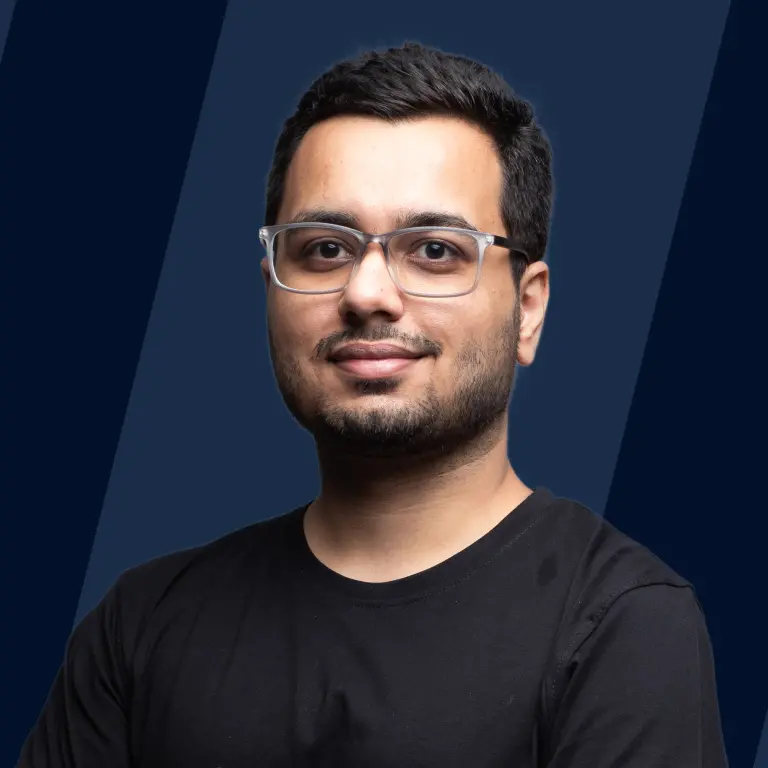
For integer N, display ASCII values of its digits. Reference the table for ASCII, decimal, hexadecimal, octal, and binary conversions.
Input/Output
Let's take an example to understand to print the ASCII value of 7.
Input:- No need to input anything. Output:- ASCII value of 7 is 55. Explanation:- The ASCII value of 7 is 55.
Program to Print ASCII Value of 1 to 9 Using the ASCII Table
Let's look at the ASCII table for the numbers from 1 to 9.
Number | ASCII value |
---|---|
1 | 49 |
2 | 50 |
3 | 51 |
4 | 52 |
5 | 53 |
6 | 54 |
7 | 55 |
8 | 56 |
9 | 57 |
It is clear from the above table that we need to add 48 to the number to get its ASCII value.
Program
Output
Explanation
In this program, we add 48 to the number to get the ASCII value of the number. We run a loop nine times, and we print the number and its ASCII value each time.
Time Complexity:- O(1), as we are running a loop for a fixed number of times(i.e., 9 times in this case). Space Complexity:- O(1), as we are not using any secondary data structure.
Program to Print ASCII Value of 1 to 9 Using Type Conversion
Program
Output
Explanation
In this program, we create a string that includes all the numbers from 1 to 9. We run a loop to iterate this string and print the number along with its ASCII value. The s[i] will give us the number, and its integer value gives us the ASCII value of the number.
Time Complexity:- O(n), where n is the length of the string. Space Complexity:- O(n), where n is the length of the string.
Program to Print ASCII Value of 1 to 9 Using While Loop
Program
Output
Explanation
In this program, we take a variable x which stores the ASCII value of 1. We run a while loop until 57(ASCII value of 9) and print the character value of x, which gives the number, and the value of x is equivalent to the ASCII value of that number.
Time Complexity:- O(1), as we are running a loop for a fixed number of times(i.e., 9 times in this case).
Space Complexity:- O(1), as we are not using any secondary data structure.
Conclusion
- The ASCII code or American Standard Code for Information Interchange is a collection of 255 symbols in the character set.
- The regular ASCII code is 7 bits long and ranges from 0 to 127, while the extended ASCII code is 8 bits long and ranges from 128 to 255.
- The ASCII codes are unique for each character.
- Printing the ASCII values through type conversion is the best way of doing it because we don't need any prior knowledge about the ASCII values of the character.