Assertions in Java
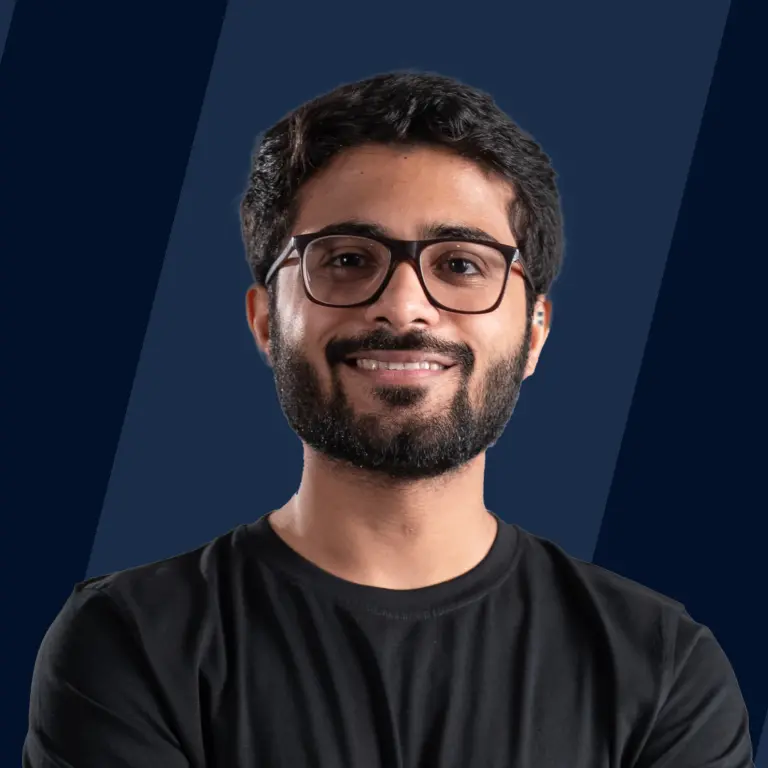
The assert in Java is a development feature that validates specific conditions, enhancing code reliability by detecting bugs early. Developers can perform runtime checks by embedding an assert statement, ensuring that key assumptions hold true. When an assertion in Java fails, it throws an AssertionError, highlighting discrepancies between expected and actual program behavior. This tool is pivotal for affirming program correctness and is primarily utilized during the development phase for internal testing and validation purposes.
Syntax
In Java, the syntax for using the assert statement comes in two forms:
- Simple Assertion:
- Syntax:
- Example:
This form evaluates the boolean expression and throws an AssertionError if the expression evaluates to false, indicating the assumption was not met.
- Assertion with a Message:
- Syntax:
- Example:
In this form, if expression1 evaluates to false, an AssertionError is thrown with expression2 providing additional context. Expression2 is converted to a string and included in the error message, aiding in debugging by offering more detailed information about the assertion failure.
Examples
To illustrate the use of assertions in Java, let's consider a simple example that checks the validity of a method's parameter.
A Simple Code Example of Assertion in Java
In this example, the assertion checks if the variable number is greater than 0. If number is not positive, an AssertionError with the message "Number is not positive" is thrown.
Enabling Assertions
-
In the Command Line: To enable assertions when running your program, use the -ea option (or -enableassertions) with the java command.
This command enables assertions for all classes except system classes.
-
For Specific Classes or Packages: You can enable assertions for specific classes or packages by specifying their names after the -ea option.
Disabling Assertions
-
In the Command Line: Although assertions are disabled by default, if you've enabled them globally, you can disable them for specific classes or packages using the -da option (or -disableassertions).
This command enables assertions globally but disables them for classes in com.example.myPackage.
Remember, assertions are primarily intended for development and testing purposes and should not be used for runtime error handling in production code.
Advantages of Assertion
- Helps in detecting errors in programming, testing, and debugging.
- Helps to remove the boilerplate code and makes code more readable.
- Done during the development and testing phase and are removed when deployed to production.
- Optimizes the code by refactoring it.
Assertion vs Normal Exception Handling
Assertions:
- Assertions are primarily used for situations that are logically impossible or should never occur.
- They are helpful for detecting programming errors and logical inconsistencies during development and testing.
Normal Exception Handling:
- Normal exception or error handling is used to prevent and handle runtime errors or exceptional conditions in the code.
- It focuses on handling expected errors or exceptional situations that can occur during program execution.
When to Use Assertions?
- You’re never supposed to handle an assertion failure. That means you should not catch it with a catch clause and attempt to recover.
- Should be used for conditional cases. The assertions should be used to validate arguments to a private method.
When Not to Use Assertions?
Not use assertion:
- Don't use assertions in order to validate arguments to a public method.
- Don’t use assertions to validate command-line arguments.
- Don’t use assert expressions that can cause side effects in the code.
Conclusion
- Java's assert statement is pivotal for verifying program correctness, primarily used to check boolean expressions during development.
- It offers two syntax forms: a simple assertion (assert expression;) and an assertion with a message (assert expression1 : expression2;), enhancing error diagnostics.
- Enabled via -ea and disabled with -da, assertions are versatile for internal testing, not meant for handling runtime errors in production.
- Their usage underscores programming logic and assumptions, with AssertionError thrown upon failure, signaling a deviation from expected behavior.