Assertionerror Python
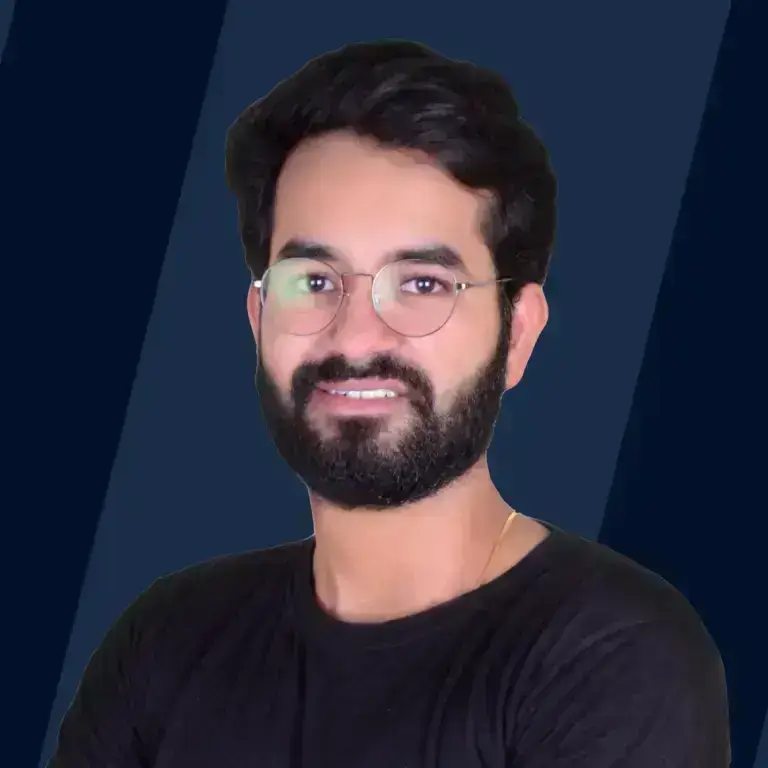
Overview
An Assertion is a programming concept that helps us to test an expression. If the expression returns true, the control of the program is moved to the next line. But if the expression returns false, an AssertionError is generated by Python. AssertionError Python can be avoided by using the -O flag, using the environment variable to set a flag, or by making sure that the flow of the program doesn't reach the assert statement. AssertionError Python is useful to check the output of a function, to check whether the input is valid or not, or to test a program.
What is Assertionerror in Python?
Before learning about AssertionError Python, let's have a brief of what is an assert statement. The assert statement is used when we want to debug the code. Consider a case where we want the expression 'x' to be always true. But can we check if 'x' is always true or not? Here comes the assert statement. An assert statement returns the AssertionError if the expression is false. Hence it halts the execution of the program.
Explanation
From the above image, we can see that if the assertion condition is false, the program is halted and AssertionError Python is returned.
Syntax
The assert statement in Python also provides us the option to add an error message in case the AssertionError Python is returned. Let's see the syntax for both cases.
Without Error Message
With Error Message
Examples
Let's have an example of the division of two numbers. When we divide a number x by another y, we know that y can not be equal to 0. Hence we have to make sure that y!=0. Here our expression will be y!=0 if it returns true we must stop the execution of the program. Let's use the assert statement to implement the same.
Output:
Now let's set y as 0 and check the program's output.
Output:
See the assert statement returned the AssertionError Python as y was equal to 0.
Now let's add the error-message to the assert statement and then check the program's output.
Output:
Here we can see that the error-message is printed stating that we "Cannot divide by 0".
How does Assertionerror in Python Work?
The Python programming language has an assert statement that allows us to generate basic debug message outputs based on logical assertions. An AssertionError Python is returned when the assertion expression is false. In Python BaseException class is the base of all the exception classes. AssertionError's base class is the Exception class which inherits from the BaseException class. When an assert statement is used in the code, we have to make sure to add the exception handling code as well. If the error-message is provided in the code, the error-message is printed else the error is handled without the error-message.
Steps to Avoid Assertionerror in Python
- The -O flag can be used to disable all assert statements in the program. By disabling the assert statements in the program, we can avoid the AssertionError exception in Python.
Example: If we try to run python -c "assert False" in the terminal, the output states that there's an assertion error as the expression is false. But we can use the -O to disable the assert statement as,The output of the above code in the terminal will be empty. As the assert statement was disabled by the -O flag.
- We can also use the environment variable to set a flag that disables the assert statements. But note, that all the processes that inherit or use this environment will be impacted if this is used.
Example: In the cmd we can use SET PYTHONOPTIMIZE=TRUE to disable the assert statement and this will affect all the processes that use or inherit the environment. Later we can enable asserts using SET PYTHONOPTIMIZE=. - Another way to avoid AssertionError Python is to make sure that the flow of the program doesn't reach the assert statement or after catching the AssertionError Python the normal flow of the program should resume. For example. using try-except block in Python.
Handling Python Assertionerror
Consider the example we used in the beginning of the article where we are dividing two numbers. We will add a try-except block to that code and let's see the output.
Output:
Can you guess why the output is empty?
This is because the except block is printing the error-message but we have not provided any error-message to the assert statement. Let's add the error-message and check the output.
Output:
Practical Applications of Python Assertion Error
- Checking values of parameters
We can check whether the provided parameters are valid or not. Just like the example we saw in the beginning of the article. - Checking valid input/type
We can also check whether the user has passed the correct data type or not. Consider the case where we are dividing two numbers and the user passes string instead of numbers. This can be caught using the assert statement. - We can also detect whether an interface is being misused by another programmer or not.
Assertionerror Python Example
Let's have an example to calculate average marks of a student,
Output:
You can see in the above program even after getting an AssertionError Python on line 10, the normal flow of the program resumes.
Conclusion
- The Python programming language has an assert statement that allows us to generate basic debug message outputs based on logical assertions.
- An AssertionError Python is returned when the assertion expression is false.
- When an assert statement is used in the code, we have to make sure to add the exception handling code as well.
- AssertionError Python can be avoided by using the -O flag, using the environment variable to set a flag, or by making sure that the flow of the program doesn't reach the assert statement.