atoi() Function in C
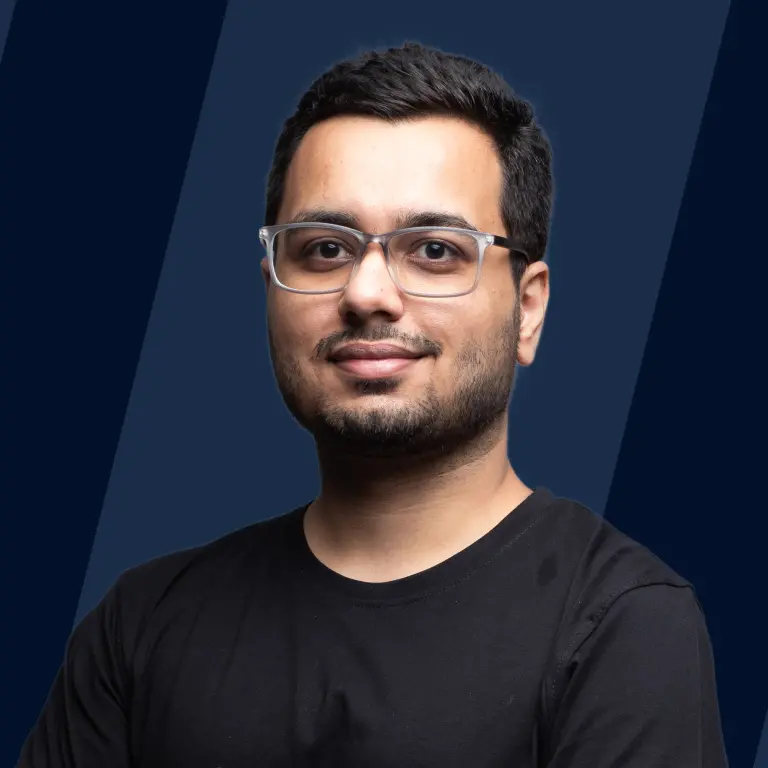
Overview
The atoi function in C converts a string of characters to an integer value. The input is a character string and the function stops reading the input when it first encounters a character that is not a number. The atoi function in C can not convert string in decimal and exponent notation. The function skips all the white spaces encountered at the start of the string. The function is specified in stdlib.h header file.
Syntax of Atoi() Function in C
The syntax of the atoi() function is:
Parameters of Atoi() Function in C
The atoi function in C accepts only one parameter as an argument which is a pointer to a string. The string is passed as const, which ensures the function doesn't change the value of the string and only returns the integer value from the string.
Return Value of Atoi() Function in C
For valid inputs, the atoi function in C returns an integer value equal to the passed string. If the passed string has non-valid input, the function returns 0. Because the atoi() function works incrementally (converts string to number by reading characters one by one), the function breaks if it encounters a non-ASCII value and skips all whitespace at the starting of the string. atoi() function can convert a string to a valid integer if the string contains the following parameter:
- Strings made of ASCII digits i.e. '0', '1', '2', '3', '4', '5', '6', '7', '8' and '9'.
- Strings beginning with characters '+' or '-' and made entirely of ASCII digits.
If the function call breaks in between, it returns either of two possible values.
- If the function is called at the start and no valid input is encountered so far, the function returns the value 0.
- Otherwise, the atoi() function returns the number encountered before the first non-digit character.
Required Header
C language requires the following header file for atoi function:
Example
Let's see an example of converting a number in a string to an integer value using the atoi() function in C.
Output
Here, we use the atoi function in C to convert the string value stored in the character array str to an integer. The atoi function returns an integer value that is assigned to the variable value.
More Examples
Now that we have seen how the atoi function works in C. Let us see different cases when an invalid or partially valid string is passed as an argument to the function.
Output
In the above example, we can make the following observations from the output of the program:
- If an invalid string is passed to the atoi function, it returns the integer value 0.
- If the atoi function in C encounters a non-digit character, it returns an integer value it can transform before the first non-digit character.
- If the string starts with the character '+' or '-' followed by ASCII digits, it is a valid input.
Conclusion
- The atoi function in C converts a string of characters to an integer value.
- To use atoi function in C we have to include stdlib.h header file in our program.
- The function reads the input string character by character and breaks when a non-digit ASCII character is encountered. It also skips the all whitespaces present at the start of the string
- The atoi() function accepts only one string parameter as input and returns its converted integer value. The input is of constant value that ensures the function does not change the value of the input string.
- If an invalid string is passed as an input, the function returns 0.