Class Attributes in Java
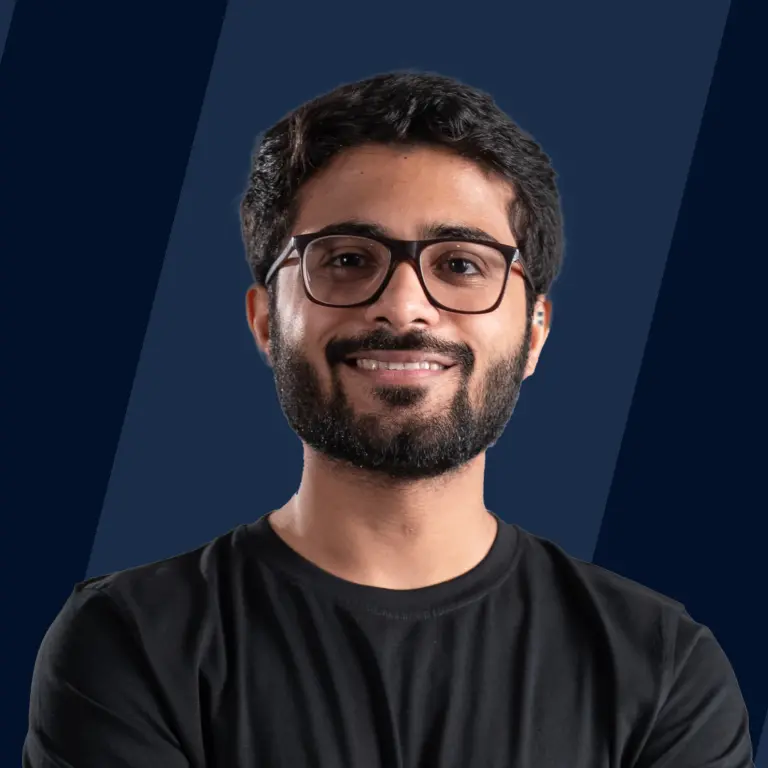
Overview
In object-oriented programming, Java stands as a prime example of a language that thrives on the concept of classes and objects. At the heart of this paradigm lies the ability of Java classes to encapsulate not only methods but also fields. These fields are known as class attributes. These attributes, essentially variables declared within a class represent distinct properties of the class. For instance, think of a class named "Student" – within it, attributes like roll number, name, and section can be defined, embodying the very essence of the Student Class. This article delves into the significance of class attributes in Java.
What is a Class Attribute in Java?
Variables contained within a class are commonly referred to as class attributes in Java. These attributes are alternatively known as fields. To better grasp the essence of class attributes, consider the example of a Student class.
Code:
Explanation:
In the above snippet name, rollNo, fathersName, and age are the attributes of the Student class.
Accessing Attributes in Java
You can access the class attributes by creating an object of the class and using the .(dot) operator. To get a better understanding, consider the following code snippet.
Code:
Output:
Explanation:
In the Student class defined above, we have class attributes as name, rollNo, fathersName, and age. In the main() function, we have created an object student of the Student class. We have then used this student object with the .(dot) operator to access the class attributes as student.name, student.rollNo, student.fathersName, student.age and printed all these attributes using print statements.
How to Modify Attributes in Java?
Code:
Output:
Explanation:
We have reused the Student class that we have used in our previous examples. In the main() function, we have created a student object. We print the previous rollNo of the student object. We then modify the rollNo using student.rollNo = 7; statement. We print the new rollNo to show that the rollNo of the student object has been modified.
The access to the class attributes in Java can be controlled by the appropriate use of access specifiers. We do not keep the class attributes as public if we do not want them to be accessed from anywhere in the program. Consider the following code snippet.
Code:
Output:
Explanation:
The private variable age can be accessed using a dot operator only inside its class i.e. class Person. In the above example, we have tried accessing the private class attribute age in the Main class. Since the private variable age can not be accessed outside the class in which it was declared, we get an error as shown in the output.
Now the question is how do we access the private class attributes of a class outside that class?
While direct access using the .(dot) operator is common for public attributes, it is recommended to use getter and setter methods for more controlled access and to follow encapsulation principles. It is a common coding practice to declare the class attributes as private/protected and expose public getter and setter methods to access these attributes. Consider the following code snippet.
Code:
Output:
Explanation:
In the above code snippet, we can see that:
- The name and age instance variables are marked as private. This means they cannot be directly accessed from outside the Person class, providing a level of data hiding.
- By providing getter methods like getName() and getAge(), we can retrieve the values of name and age outside the Person class without directly accessing the private variables. This allows the class to maintain control over how its properties are accessed.
- Setter methods like setName(String name) and setAge(int age) allow controlled modification of the private variables. They can include validation and business logic checks before allowing changes to the data. For instance, the setAge() method ensures that negative ages are not allowed.
Multiple Objects in Java
In the scenarios where you have to create multiple objects of a single class, you can change the class attribute in one object, without affecting the class attribute values of the other object. Consider the following code snippet.
Code:
Output:
Explanation:
In the main() function of the Student class, we have created two objects viz. student1 and student2. The class attributes of student1 take the default values defined in the Student class. We modify the values of class attributes of student2 using the .(dot) operator. We have then printed the class attributes of both student1 and student2 showing that altering the class attributes of student2 doesn't affect the class attributes of student1.
Multiple Attributes
As it is clear from the examples above, a class can have many class attributes. Consider the following code snippet.
Code:
Output:
Explanation:
In the above code snippet, the Student class has multiple class attributes viz. firstName, lastName, rollNo, fathersName, age, and isClassMonitor. We can add more class attributes as per the requirement of the project.
Using Final Keyword with Java Attributes
If you define a class attribute as final, its value can not be overridden. Consider the following code snippet to understand this better.
Code:
Output:
Explanation:
In the code snippet above, we have a main() function in which we have created a student object. The attributes of the student object have the default value mentioned in the Student class. Now we are modifying the attributes of the student object using the .(dot) operator. When we try modifying the final class attribute name, it gives us an error as shown above. The error clearly states that final class attributes can not be reassigned.
Now let us try running the same code by removing the statement where we are trying to modify the final attribute i.e. student.name = "Chandler";
Code:
Output:
Explanation:
In the above code snippet, we can see that all the class attributes that were not defined as final were modified.
The final keyword is a modifier in Java that is used when you want a certain variable to have a constant value that doesn't change during the program.
Code:
FAQs
Q. What are Attributes in Java?
A. Attributes in Java refer to the characteristics or properties of a class or an object. They are represented by fields or variables defined within a class and encapsulate the data associated with the object.
Q. What are access modifiers that the class attributes in Java can have?
A. The class attributes in Java can have different access modifiers such as private, public, protected, or default. The access modifier determines the visibility of the attribute to other classes.
Q. How are Attributes declared and accessed?
A. Class attributes can be accessed and modified using getter and setter methods. They can be accessed directly using the .(dot) operator if they have public access.
Conclusion
- Variables contained within a class are commonly referred to as class attributes in Java. These attributes are alternatively known as fields.
- We can access the class attributes or even modify them by creating an object of the class and using the .(dot) operator.
- You can create multiple objects from a class and modify the class attributes of one object without affecting the class attributes of the other objects.
- A class can have multiple class attributes.
- If you define a class attribute as final, its value can not be overridden.