What Are the Types of Attributes in Python?
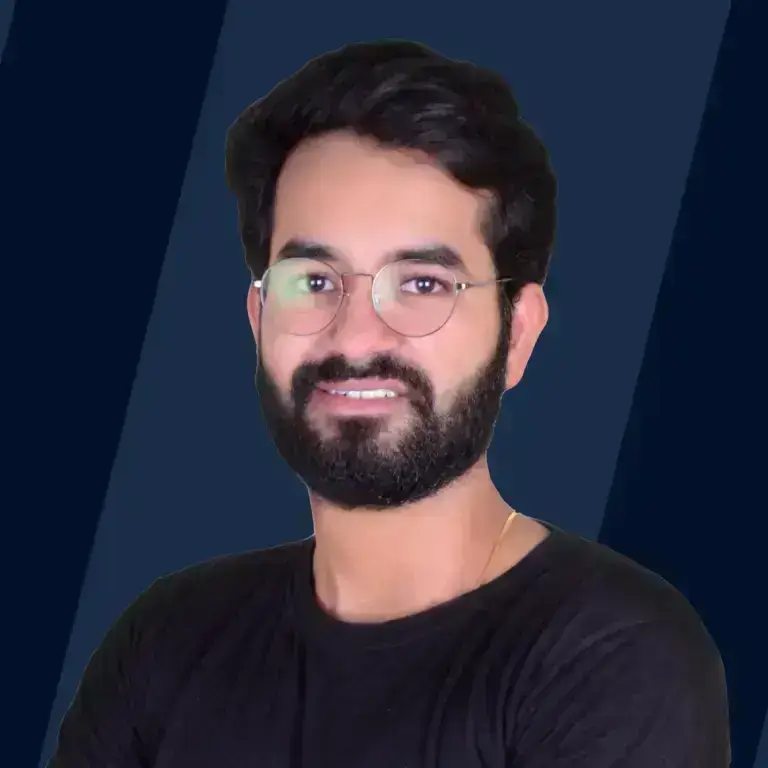
Before learning about attributes in Python, we must be familiar with the concepts of Classes and Objects in Python, let us first briefly discuss the concepts of classes and objects for a better understanding of attributes in Python.
A class is a user-defined data type or blueprint or prototype using which objects are created. Classes provide a method through which we can bind the data and its associated functionalities together. Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state.
Example:
In the above example, we have created a class Student and it has some properties (properties of the class) namely - name and roll.
An Object is an instance of a class. In simpler terms, we can say that an object is a variable of class type. A class is like a blueprint while an instance is a copy of the class with actual values. Python is an object-oriented programming language that stresses objects i.e. it mainly emphasizes functions and methods. Objects are an encapsulation of data variables and methods acting on that data into a single entity.
Example:
Output:
In the above example, we are printing the property of the class using the . (dot) operator as the value of the name property was stored in the class.
Now in terms of classes and objects, these properties are known as class attributes. So, when we create objects in Python, we create attributes that can be shared between various objects of objects. We can also create attributes that cannot be shared and will be unique for each object of the class.
In simpler terms, we can say that the properties and behavior of a class are called attributes in Python.
Note: We must know that all the attributes of the Python class can be invoked using the dir() function as well as the vars() function (It is discussed in detail in later sections).
We have two types of attributes in Python namely- Class attribute and Instance attribute. Let us now discuss them in detail using the example(s) wherever necessary.
1. Class Attribute
An attribute that belongs to a class in Python is called as a class attribute, sounds circular? Let us discuss more. An attribute that is declared and initialized outside any method of the class is known as a Python class attribute. A class attribute can be accessed by a class as well as any instance of the class (object of the class).
In simpler terms, we can say that the class attributes are variables of the class and they can be inherited by every object of that class. Since the class attributes can be accessed by every object of that class, the value of the class attribute remains the same for all the instances of the class (objects of the class).
The class attributes are defined outside the __init__() functions in Python. Now, what is this __init__() function, and how it is related to classes?
Well, __init__() is a method in Python, it can be seen as an equivalent constructor method of the C++ constructor or Java constructor (in an object-oriented programming approach). The __init__() is used to initialize the object, hence it is called every time an object is created using a class. The __init__() method lets the class initialize the object’s attributes and serves no other purpose. The method is only used within classes. It is a class variable that helps us to define various values for each object of a class. We declare the instance attribute (the second type of attribute) in the __init__() method. We will discuss instance attributes in the next sub-section
Let us take an example for more clarity about the class attributes in Python.
Output:
Let us learn about the above example. In the above example, we have created a class Student having one class attribute name. We have also defined some properties under the __init__() function that we will discuss in the next section. After that, we created an object or an instance, or a variable of the class Student.
Since the name variable is a class attribute, it can be accessed by both the class as well as any object of the class. So, we have printed the value of the attribute using an object as well as the class.
2. Instance Attribute
An attribute that belongs to an instance of the object is called as an instance attribute. Let us discuss more. An attribute that is declared and initialized inside the __init__() method of any class is known as a Python instance attribute. An instance attribute can be accessed by an object of the class because the __init__() method is called at the time of object initialization. Hence, instance attributes cannot be called directly via class name.
In simpler terms, we can say that the instance attributes are variables of the object and they can be different from every object of that class as the attribute values are initialized using objects only.
Let us take an example for more clarity about the instance attribute in Python.
Output:
In the above example, we have created a class Student having the __init() function that will initialize the instance attributes of the class. After that, we created an object or an instance or a variable of the class Student and invoked the instance attributes of the class using object names.
What is the use of self here? Now, the self is used to represent the instance of the class. By using the self we can access the attributes and methods of the class in Python. We can correlate self with the this operator of C++ which has the same usage. It binds the attributes with the given arguments. We use self in Python classes because Python does not use the @ syntax to refer to instance attributes. Python decided to do methods in a way that makes the instance to which the method belongs be passed automatically, but not received automatically: the first parameter of methods is the instance the method is called on. self always points to the current object.
Now, if we try to print the last commented line of the above program, an error will be generated i.e. AttributeError. It will look something like this.
Since the __init__() function will be invoked at the time of the creation of an object, we cannot call the instance attributes using class names.
How Attributes Works?
Whenever we try to access any attribute using any object of the class, the Python interpreter searches the invoked attribute in the list of object attributes first. In the invoked attribute is found in the instance attributes list then the Python interpreter uses that otherwise the Python interpreter extends its searching in the class attributes list. Python returns the value of the invoked attribute as long as it finds the attribute in the instance attribute list or class attribute list.
However, if we try to access a class attribute, the Python interpreter directly searches for the attribute in the class attribute list.
The static methods and class methods are associated with the class whereas the instance method is associated with an instance of the class.
What is Namespace in Python and How it is Related to Attributes in Python?
The namespace is nothing but a dictionary that contains the keys as objects of the class and values as the attributes of the class. A namespace is divided into two categories i.e. class namespace or object namespace. So whenever we try to access any attribute, it is first searched in the object namespace, and then it is searched in the class namespace.
Now, the accessing time of the object attribute is lower than the time of accessing the class attribute since first the object namespace is searched. Now, when the Python interpreter cannot find the attribute in the class namespace, it throws an error.
Different Methods to Access Attributes in Python
As we have discussed above, we have two types of attributes in Python namely class attribute and instance attribute. We can invoke or access these attributes using the class name and the object name. But is there any other method to access a class attribute? The answer to the question is Yes! We can access the class attributes and instance attributes using some built-in Python methods and functions. Let us learn about them.
- getattr() – As the name suggests, the getattr() function is used to access the attributes of an object. This function takes two arguments i.e. object name and the name of the attribute that we want to get.
- hasattr() – The function hasattr() is used to check if an attribute exists in the class or not. This function also takes two arguments i.e. object name and the name of the attribute that we want to check.
- setattr() – The function setattr() is used to set the value(s) of any attribute.Now if the attribute does not exist, then it will create the attribute for us. This function takes three arguments i.e. object name, attribute name, and the value of the attribute that we want to set.
- delattr() – As the name suggests, the delattr() function is used to delete an attribute. If we try to access the attribute after deleting it, the Python interpreter raises an error called: class has no attribute. This function also takes two arguments i.e. object name and the name of the attribute that we want to delete.
Let us take an example for better understanding.
Output:
In the above function, we have created a class Student and defined two class attributes namely name and roll. After that, we created an object for the Student() class.
Now, we have used the getattr() function to get the name attribute of the class. So, it will return Sushant. After that, we used the function hasattr() to check whether the name attribute is present in the class or not. Since it is present then it will return True.
Now, we have used the setattr() function to set the value of the class attribute college. After that, we deleted the name attribute from the Student class. So, when we tried to access the name attribute, at last, using the getattr() function, it will show an error and the attribute got deleted in the previous line.
Applications of Attributes in Python
Let us now look at some of the applications of attributes in Python.
- Class attributes are used to create constant value variables (the variables that do not change their values after their initialization).
- The class attributes can be used in the other methods of the class as well.
- The attributes generally take lesser memory space and less time to initialize than normal variables.
- The class attribute can also be used to list the objects across all the instances.
- The attributes can be used to provide a default value to the variables. This feature comes in handy when we built a large application using classes and objects and we want certain default values if the value of the variable is not provided. For example, if the user does not provide any name then we can set the default name of the user as Anonymous.
- We can change the class attribute to the instance attribute by just changing the value of the class attribute with an instance of the class.
- Python interpreter treats functions and attributes as the same. Static methods, class methods, and instance methods all belong to the class.
- We can invoke the instance attributes using the class attribute by passing the instances of the object in the method. This feature saves the memory of the Python program.
Examples of Attributes in Python
Let us check an example in which we will try to access the attributes of one class in another class. We can access the attributes of one class into another class by passing the object of one class to another.
Output:
First, we have created a class named A. In the class we have initialized two instance attributes i.e. variable1 and variable2 using the __init__() method (constructor). We have also defined a method i.e. testMethod() that will assign the sum of both these variables into variable1 and then return it.
Again we have defined a class named B which inherits class A. Now, using the __init__() method, we have initialized the instance attributes of the second class (B) as well.
Now, we have created an object of class A and then called its testMethod() and stored its value in the sum variable, and printed it. Similarly, we have created an object of class B and then printed the instance attribute values of class B.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.
Conclusion
- The properties and behavior of a class are called as attributes in Python. When we create objects in Python, we create attributes that can be shared between various objects of objects.
- We have two types of attributes in Python namely- Class attribute and Instance attribute.
- An attribute that is declared and initialized outside any method of the class is known as a Python class attribute. A class attribute can be accessed by a class as well as any instance of the class (object of the class).
- An attribute that is declared and initialized inside the __init__() method of any class is known as a Python instance attribute. An instance attribute can be accessed by an object of the class.
- The instance attributes are variables of the object and they can be different from every object of that class as the values of the attributes are initialized using objects only.
- Whenever we try to access any attribute using any object of the class, the Python interpreter searches the invoked attribute in the list of object attributes first. Then the Python interpreter extends its searching in the class attributes list.
- We can access the class attributes and instance attributes using some built-in Python methods and functions.
- Class attributes are used to create constant value variables. The class attributes can be used in the other methods of the class as well.
- We can change the class attribute to the instance attribute by just changing the value of the class attribute with an instance of the class.
- We can invoke the instance attributes using the class attribute by passing the instances of the object in the method. This feature saves the memory of the Python program.