Autoboxing and Unboxing in Java
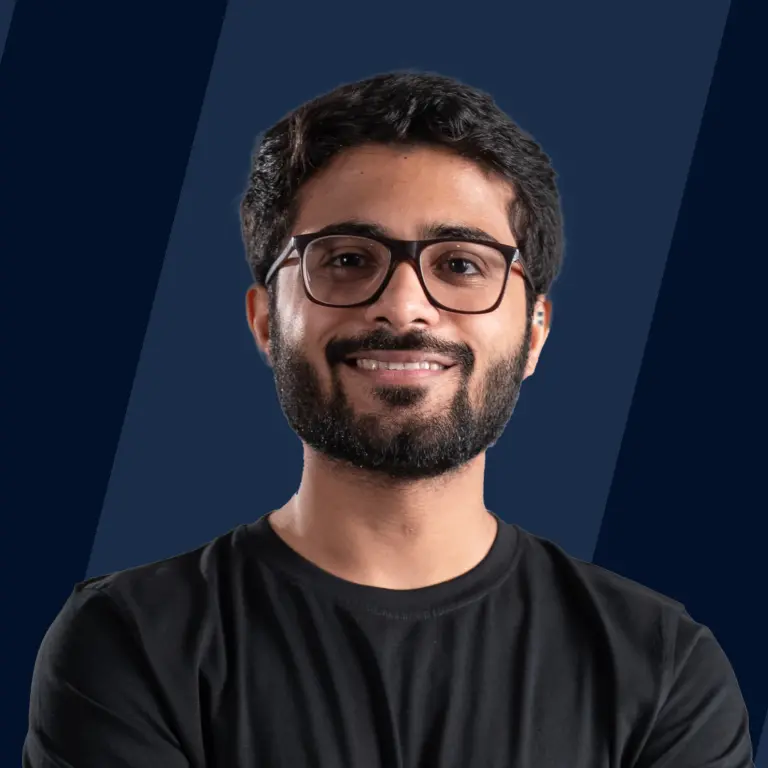
The automatic transformation of a primitive type variable into its matching wrapper class object is known as autoboxing. When a primitive value is passed as a parameter to a function that expects a wrapper class object, the compiler automatically handles the conversion. It's assigned to a wrapper class type variable. The online Java compiler automatically converts between primitive types and the object wrapper classes that correspond to them. For example, it automatically converts from int to int, double to double, etc.
Unboxing is the process of automatically converting objects of wrapper classes to their corresponding primitives, for as Integer to int.
The automatic processes of autoboxing and unpacking, which were added to Java in version 1.5, are handled by JRE
==JRE(Java Runtime Environment) is an installation package that gives your computer a running environment for Java programs (or applications), not a development environment. JRE is solely utilized by end users of your system who want to run Java apps..==
The most crucial thing in this situation is to create decent code that doesn't introduce too many extraneous objects into the scene.
Let's now talk about how Autoboxing and Unboxing work inside Java
Internally, the compiler employs the valueOf() function to automatically box primitives into appropriate wrapper objects, while for unpacking, it uses paradigms akin to intValue(), doubleValue(), etc.
The wrapper and primitives mapping in Java is described below for reference:
Primitive type | Wrapper class |
---|---|
boolean | Boolean |
byte | Byte |
char | Character |
float | Float |
int | Integer |
long | Long |
short | Short |
double | Double |
Let's see an example of implementing Autoboxing in Java
Output
Explanation
In the example above, we made an array list of the type Integer. As a result, the array list can only include objects of the type Integer.
Observe the line.
list.add(1); We are passing a primitive type value in this case. The primitive value, however, is automatically transformed into an Integer object and saved in the array list as a result of autoboxing.
Learn more about wrapper classes here
Example of Autoboxing and Unboxing in Java
Let’s take an ArrayList of Integers now and then make see how unboxing under the hood can convert Integer to int
Output
Hence, in the above example, while adding value to x, we see that x appears to be primitive, so unboxing happens here automatically while this assignment is done
Output
If you are familiar with how Java creates objects, you will notice that Integer sum = 0 defines an object and that when the calculation is performed inside the for loop, the primitive value I is added to the wrapper sum.
Since the + operator only operates on primitives, it follows that object unboxing will take place first. The computation will then happen, followed by object autoboxing once more, and the value will then be assigned to the variable sum
As several intermediary Integer objects will be produced and destroyed (to be later trash collected), this places needless burden on the JVM and may cause a slowdown. As a result, such logic should be handled carefully.
==JVM (Java Virtual Machine) is a very important part of both JDK and JRE because it is contained or inbuilt in both. Whatever Java program you run using JRE or JDK goes into JVM and JVM is responsible for executing the Java program line by line, hence it is also known as an interpreter==
Let's look at some scenarios that defy conventional wisdom and use some code where autoboxing and unpacking become crucial concepts to comprehend.
What do you think will happen when you run the snippet that is below?
Output
We can only compare the integer range from -128 to 127 in this way, therefore if you answer "yes," you are "false" because the result is likewise "false." Values outside of this range must be unboxed.
As a result, we must compare the intValue() of the aforementioned integers; for the time being, the compiler handles this using valueOf() property.
The above code, as written, will likely return true if this is within the range mentioned above because it first makes use of the integer literal pool for comparison.
Output
This program evaluates to true value, as 100 is present in the literal pool.
Advantages of Autoboxing and Unboxing in Java
Now that we're clear on the basics of what Autoboxing in Java is, you must be wondering why do we need Autoboxing in the first place?
Following are the advantages of Autoboxing in Java
- No need for conversion between primitives and Wrappers manually so less coding is required
- Autoboxing and unboxing lets developers write cleaner code, making it easier to read.
- The technique lets us use primitive types and Wrapper class objects interchangeably and we do not need to perform any typecasting explicitly
- The best strategy for transformation is consequently picked, e.g. Integer.valueOf(int) is utilized rather than new Integer(int)
Autoboxing and Unboxing with Comparison Operators
Autoboxing can be performed with comparison operators.
Now what are these comparison operators? Let's take a look
Comparison operators can evaluate and compare strings or numbers. In contrast to arithmetic expressions, comparison operator expressions do not return a numerical value. Expressions used for comparison yield either 1, which represents the truth, or 0, which represents the opposite
Let's take a look at some of the comparison operators and their meanings
Operator | Meaning |
---|---|
= | Equal |
== | Strictly Equal |
\ = | Not equal |
\ == | Not strictly equal |
> | Greater than |
< | Less than |
> < | Greater than or less than (same as not equal) |
> = | Greater than or equal to |
\ < | Not less than |
< = | Less than or equal to |
\ > | Not greater than |
Let's see the example of boxing with comparison operator in Java:
Output
Here, our program performs unboxing internally in order to make the comparison with 100 and obtain a result for the conditional statement
Autoboxing and Unboxing with Method Overloading
It is assumed that the reader is familiar with the notion of method overloading before we tag autoboxing and unpacking with it. Let's study a little bit more about method overloading first.
Method overloading is the technique of presenting several variations of any calculation method often by using the same method name with a varied amount of input parameters, different data kinds of variables with the same names, etc.
If you write the method as a(int,int) for two parameters and b(int,int,int) for three parameters then it may be difficult for you as well as other programmers to understand the behavior of the method because its name differs. Suppose you have to perform addition of the given numbers but there can be any number of arguments.
So, in order to understand the program, we use method overloading.
In Java, there are two ways to overload a method.
- By changing number of arguments
- By changing the data type
==Java does not allow method overloading by merely modifying the method's return type.==
1. Method Overloading by Changing the Number of Arguments
In this example, we have built two methods; the first, add(), adds two numbers, and the second, adds three numbers.
To avoid having to build an instance before using a method, we are creating static methods in this example.
Output:
2. Method Overloading by Changing the Data Type of Arguments
In this instance, we have developed two procedures with various data types. Two integer arguments are passed to the first add method, and two double arguments are passed to the second add method.
Output:
Learn more about method overloading here
Continuing with our topic,
You might encounter a situation in method overloading when a signature accepts a reference type or a primitive type as a formal parameter.
The compiler first looks for a method that has one or more parameters with the same data type (s). When you use the wrapper class Object as an actual argument and the compiler is unable to locate a method with reference type (a class or interface type) matching parameter(s), it begins looking for a method with primitive data type matching parameter(s).
To better comprehend it, let's look at an example, and this time we'll also show the result that is shown in the image.
Output
The aforementioned behavior thus makes it very evident that the autoboxing technique is heavily used in overloading concepts and should be used with caution while developing.
Boxing and unpacking are operations that can be done in method overloading. The following guidelines apply to method overloading in boxing:
- Widening beats boxing
- Widening beats varargs
- Boxing beats varargs
1. Example of Autoboxing Where Widening Beats Boxing
If there is the possibility of widening and boxing, widening beats boxing.
Output
2. Example of Autoboxing Where Widening Beats Varargs
If there is the possibility of widening and varargs, widening beats var-args.
Output
3. Example of Autoboxing Where Boxing Beats Varargs
Let's see the program where boxing beats variable argument:
Output
Conclusion
- The automatic transformation of a primitive type variable into its matching wrapper class object is known as autoboxing
- Unboxing is the process of automatically converting objects of wrapper classes to their corresponding primitives, for as Integer to int
- Internally, the compiler employs the valueOf() function to automatically box primitives into appropriate wrapper objects, while for unpacking, it uses paradigms akin to intValue(), doubleValue(), etc.
- Method overloading is the technique of presenting several variations of any calculation method often by using the same method name with a varied amount of input parameters, different data kinds of variables with the same names, etc.
- Java does not allow method overloading by merely modifying the method's return type