BigDecimal Java
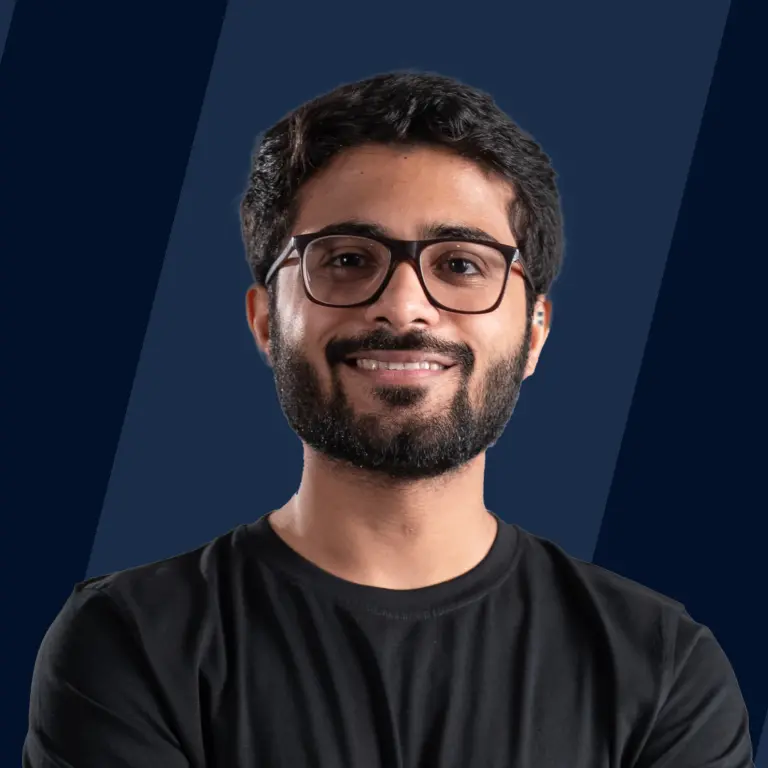
Overview
The class BigDecimal is a part of **`java.math package. For decimal numbers, we use the float and double primitive data types, however, there is a drawback to using these primitive types. Never use a float or double type for an exact value, such a cash number.
Introduction to BigDecimal Java
The operations for arithmetic, scale manipulation, rounding, comparison, hashing, and format conversion are provided by the BigDecimal class. Additionally, the class offers total control over rounding behavior to the user.
The BigDecimal class in Java consists of a 32-bit integer scale and a random precision integer scale. If the scale is positive or zero, the number of digits to the right of the decimal point is used. If the number's unscaled value is multiplied by ten to the power of the scale's negation, it will be less than zero.
Features of BigDecimal are
- Scaling and Rounding Modes
- No Overloaded Operators are allowed
- use CompareTo() to compare BigDecimals not equals()
- BigDecimals are immutable.
Java BigDecimal Declaration
The declaration of BigDecimal Class is shown below
In this example, we are going to see how to initialize BigDecimal Class.
Output
Code Explanation In the above code, we have created an Object of BigDecimal Class and initialized that object with some value.
Need of BigDecimal
- The two primitive types in Java, double and float, are binary representations of a fraction and an exponent that are used to store floating point numbers.
- Aside from booleans, fixed-point integers are other primitive types. In contrast to fixed point numbers, floating point numbers typically return a result with a little error (about 10-19). This is the reason why, in the example above, the outcome of 0.04-0.03 is 0.009999999999999998. In this example, we are going to check why there is a need for BigDecimal in java
Output
Code Explanation In the above example, we have shown subtraction with both double and BigDecimal. By using BigDecimal more precise answers can be achieved.
Fields for BigDecimal Class
Following are Fields of BigDecimal class:
Field | Description |
---|---|
static BigDecimal ONE | The number 1 on a scale of 0; |
static int ROUND_CEILING | Rounding in the direction of positive infinity. |
static int ROUND_DOWN | Rounding in the direction of zero. |
static int ROUND_FLOOR | Rounding in the direction of negative infinity. |
static int ROUND_HALF_DOWN | If both neighbors are equally distant from one another, the rounding manner is to "round down" otherwise, it is to "nearest neighbor" |
static int ROUND_HALF_EVEN | rounding mode to state that rounding is unnecessary because the requested operation has an exact result. An ArithmeticException is raised if this rounding mode is specified on an operation that produces an inexact result. |
static int ROUND_HALF_UP | If both neighbours are equally distant from one another, rounding mode is set to "nearest even neighbour," otherwise, it is set to "nearest neighbor" |
static int ROUND_UNNECESSARY | rounding mode to state that rounding is unnecessary because the requested operation has an exact result. An ArithmeticException is raised if this rounding mode is specified on an operation that produces an inexact result. |
static int ROUND_UP | Rounding in the opposite direction of zero. |
static BigDecimal TEN | 10, on a scale of 0 to 10. |
static BigDecimal ZERO | 0, on a scale of 0 to 10. |
Java BigDecimal Constructors
The Constructors of BigDecimal Class are Shown Below
Constructor | Description |
---|---|
BigDecimal(BigInteger val) | Converts a BigInteger to a BigDecimal. |
BigDecimal(BigInteger unscaledVal, int scale) | A BigInteger unscaled value and an int scale are converted to a BigDecimal. |
BigDecimal(BigInteger unscaledVal, int scale, MathContext mc) | Converts an unscaled BigInteger number and an int scale to a BigDecimal, rounding according to the context settings. |
BigDecimal(BigInteger val, MathContext mc) | The context settings are used to convert a BigInteger to a BigDecimal rounding |
BigDecimal(char[] in) | Accepts the same sequence of characters as the BigDecimal(String) Constructor for converting a character array representation of a BigDecimal to a BigDecimal. |
BigDecimal(char[] in, int offset, int len) | Allows you to convert a character array representation of a BigDecimal into a BigDecimal, taking the same sequence of characters as the BigDecimal(String) Constructor and enabling you to provide a sub-array. |
BigDecimal(char[] in, int offset, int len, MathContext mc) | Allows you to convert a character array representation of a BigDecimal into a BigDecimal, taking the same sequence of characters as the BigDecimal(String) Constructor and rounding according to the context settings. |
BigDecimal(char[] in, MathContext mc) | Accepts the same sequence of characters as the BigDecimal(String) Constructor and rounds according to the context settings to convert a character array representation of a BigDecimal into a BigDecimal. |
BigDecimal(double val) | Converts a double to a BigDecimal, which is the precise decimal representation of the binary floating-point value of the double. |
BigDecimal(double val,MathContext mc) | Converts a double to a BigDecimal, rounding as specified by the context. |
BigDecimal(int val) | Convert int To BigDecimal |
BigDecimal(int val, MathContext mc) | Converts an int to a BigDecimal with rounding based on the context settings. |
BigDecimal(long val) | Convert Long to BigDecimal |
BigDecimal(long val, MathContext mc) | Converts a long to a BigDecimal, rounding according to the context settings. |
BigDecimal(String val) | Converts a BigDecimal's string representation to a BigDecimal. |
BigDecimal(String val, MathContext mc) | Accepts the same strings as the BigDecimal(String) Constructor, with rounding based on the context settings, to convert the string representation of a BigDecimal into a BigDecimal. |
Java BigDecimal Methods
Method | Description |
---|---|
BigDecimal abs() | Returns a BigDecimal with the absolute value of this BigDecimal and the scale this. scale(). |
BigDecimal abs(MathContext mc) | Returns a BigDecimal with the absolute value of this BigDecimal, rounded according to the context settings. |
BigDecimal add(BigDecimal augend) | This function returns a BigDecimal with the value (this + augend) and the scale max(this.scale(), augend.scale()). |
BigDecimal add(BigDecimal augend, MathContext mc) | Returns a BigDecimal containing the value (this + augend), rounded to the context settings. |
byte byteValueExact() | Checks for missing information before converting this BigDecimal to a byte. |
int compareTo(BigDecimal val) | This BigDecimal is compared to the provided BigDecimal. |
BigDecimal divide(BigDecimal divisor) | Returns a BigDecimal with the value (this / divisor) and the chosen scale (this.scale() - divisor.scale()); an ArithmeticException is issued if the precise quotient cannot be represented (due to a non-terminating decimal expansion). |
BigDecimal divide(BigDecimal divisor, int roundingMode) | This method returns a BigDecimal with the value (this / divisor) and the scale this.scale(). |
BigDecimal divide(BigDecimal divisor, int scale, int roundingMode) | Returns a BigDecimal with the value (this / divisor) and the scale supplied. |
BigDecimal divide(BigDecimal divisor, MathContext mc) | Returns a BigDecimal whose value is (this / divisor), with rounding according to the context settings. |
BigDecimal divide(BigDecimal divisor, RoundingMode roundingMode) | This method returns a BigDecimal with the value (this / divisor) and the scale this. scale(). |
BigDecimal[] divideAndRemainder(BigDecimal divisor) | The result of divideToIntegralValue is followed by the result of the remainder on the two operands, resulting in a two-element BigDecimal array. |
BigDecimal[] divideAndRemainder(BigDecimal divisor, MathContext mc) | Returns a two-element BigDecimal array with the result of divideToIntegralValue followed by the result of the remainder on the two operands computed with rounding based on the context settings. |
BigDecimal divideToIntegralValue(BigDecimal divisor) | Returns a BigDecimal whose value is the rounded-down integer component of the quotient (this / divisor).. |
BigDecimal divideToIntegralValue(BigDecimal divisor, MathContext mc) | The integer component of (this / divisor) is returned as a BigDecimal. |
double doubleValue() | This BigDecimal is converted to a double. |
boolean equals(Object x) | This BigDecimal is compared to the provided Object for equality. |
float floatValue() | This BigDecimal is converted to a float. |
int hashCode() | This BigDecimal's hash code is returned. |
int intValue() | This BigDecimal is converted to an int. |
int intValueExact() | Converts this BigDecimal to an int, checking for lost information. |
long longValue() | This BigDecimal is converted to a long. |
long longValueExact() | Checks for missing information by converting this BigDecimal to a long. |
BigDecimal max(BigDecimal val) | The maximum of this BigDecimal and val is returned. |
BigDecimal min(BigDecimal val) | The minimum of this BigDecimal and val is returned. |
BigDecimal movePointLeft(int n) | Returns a BigDecimal with the decimal point shifted n places to the left of the current one. |
BigDecimal movePointRight(int n) | Returns a BigDecimal with the decimal point shifted n places to the right of this one. |
BigDecimal multiply(BigDecimal multiplicand) | This function returns a BigDecimal with the value (this multiplicand) and the scale (this.scale() + multiplicand.scale()). |
BigDecimal multiply(BigDecimal multiplicand, MathContext mc) | Returns a BigDecimal containing the value (this multiplicand), rounded to the context settings. |
BigDecimal negate() | Returns a BigDecimal with the value (-this) and the scale this. scale(). |
BigDecimal negate(MathContext mc) | Returns a BigDecimal with the value (-this), rounded to the context settings. |
BigDecimal plus() | Returns a BigDecimal with the value (+this) and the scale this.scale (). |
BigDecimal plus(MathContext mc) | Returns a BigDecimal with the value (+this) and rounding based on the context settings. |
BigDecimal pow(int n) | The power is computed accurately, to limitless accuracy, and returned as a BigDecimal. |
BigDecimal pow(int n, MathContext mc) | Returns a BigDecimal with the value (this). |
int precision() | This function returns the accuracy of this BigDecimal. |
BigDecimal remainder(BigDecimal divisor) | This method returns a BigDecimal with the value (this% divisor). |
BigDecimal remainder(BigDecimal divisor, MathContext mc) | Returns a BigDecimal with the value (this% divisor) and rounding based on the context settings. |
BigDecimal round(MathContext mc) | Returns a BigDecimal that has been rounded in accordance with the MathContext settings. |
int scale() | This function returns the scale of this BigDecimal. |
BigDecimal scaleByPowerOfTen(int n) | Returns a BigDecimal with the numerical value (this * 10n). |
BigDecimal setScale(int newScale) | Returns a BigDecimal with the given scale and a value that is numerically equivalent to this BigDecimal's. |
BigDecimal setScale(int newScale, int roundingMode) | Returns a BigDecimal with the given scale and an unscaled value calculated by multiplying or dividing the unscaled value of this BigDecimal by the appropriate power of ten to retain its overall value. |
BigDecimal setScale(int newScale, RoundingMode roundingMode) | Returns a BigDecimal whose scale is the provided value and whose unscaled value is calculated by multiplying or dividing this BigDecimal's unscaled value by the appropriate power of ten to retain its overall value. |
short shortValueExact() | Checks for missing information by converting this BigDecimal to a short. |
int signum() | This function's signum function is returned. |
BigDecimal stripTrailingZeros() | Returns a BigDecimal that is numerically equivalent to this one but with any trailing zeros removed. |
BigDecimal subtract(BigDecimal subtrahend) | This function returns a BigDecimal with the value (this - subtrahend) and the scale max(this.scale(), subtrahend.scale()). |
BigDecimal subtract(BigDecimal subtrahend, MathContext mc) | Returns a BigDecimal containing the value (this - subtrahend), rounded to the context settings. |
BigInteger toBigInteger() | This BigDecimal is converted to a BigInteger. |
BigInteger toBigIntegerExact() | Checks for missing information before converting this BigDecimal to a BigInteger. |
String toEngineeringString() | If an exponent is required, a text representation of this BigDecimal is returned, using engineering notation. |
String toPlainString() | This BigDecimal's text representation without an exponent field is returned. |
String toString() | If an exponent is required, the textual representation of this BigDecimal is returned, using scientific notation. |
BigDecimal ulp() | This BigDecimal's ulp size, a unit in the final location, is returned. |
BigInteger unscaledValue() | The unscaled value of this BigDecimal is returned as a BigInteger. |
static BigDecimal valueOf(double val) | Uses the double's canonical string representation offered by the Double.toString(double) function to convert a double to a BigDecimal. |
static BigDecimal valueOf(long val) | Converts a long number to a BigDecimal with a scale of 0. |
static BigDecimal valueOf(long unscaledVal, int scale) | A long unscaled number and an int scale are converted into a BigDecimal. |
Examples for the BigDecimal Java
Example1
In this example, we are going to Compare two BigDecimal using the equals() method.
Output
Code Explanation In the Above code, we have created two Objects of BigDecimal using BigDecimal(String) Constructor. Then We used equals() method to check if two BigDecimal are equal or not.
Example 2
In this Example, we are going to use round() method of BigDecimal class.
Code Output
Code Explanation In Above Code We have Created BigDecimal Object and round it with precision of 3 by using round() Method with passing MathContext Object.
Mathematical Operations
Several Mathematical Operations can be Applied on BigDecimal Number for example addition, subtration, multiplication, power, division, remainder. Let us take an Example where we are going to find how to use these Mathematical operations on BigDecimal.
Output
Code Explanation In Above Code we have called the BigDecimal method to find the addition, subtraction, division, and multiplication of BigDecimal Numbers. To find the division of two BigDecimal Numbers we have passed RoundingMode as RoundUp.
Extraction of Value from BigDecimal
1) Using java.math.BigDecimal.intValue() to Get Extract Value from BigDecimal
From Below we are going to find how to extract value from BigDecimal using intValue() method
Code Output
Code Explanation In Above Code we have created two BigDecimal Objects and extracted their integer value using intValue().
2) Using java.math.BigDecimal.longValue() to Extract Value from BigDecimal
From Below we are going to find how to extract value of long type from BigDecimal using longValue() method
Output
Code Explanation In the Above code by using longValue() we have extracted BigDecimal value into long.
3) Using java.math.BigDecimal.toBigInteger() to Extract Value from BigDecimal
From Below we are going to find how to extract value of BigInteger type from BigDecimal using toBigInteger() method
Output
Code Explanation In the Above code by using toBigInteger() we have extracted the BigDecimal value into BigInteger.
Comparison
"equals()"
The Java.math.BigDecimal.equals() method determines whether a BigDecimal value and the object provided are equal. Two BigDecimal objects are only deemed equal by this method if their scale and value are the same.
To compare two BigDecimal equals() method is used.
In this example, we are going to use equal() method.
Output
Code Explanation In Above Code we have created two BigDecimal with the same value and scales and used equals() method.
"compareTo()"
Compares the provided BigDecimal to BigDecimal. This function treats two BigDecimal objects that are equal in value but differ in scale (for example, 2.0 and 2.00). In place of separate methods for each of the six boolean comparison operators (, ==, >, >=,!=, =), this method is offered. (x.compareTo(y) op> 0), where op> is one of the six comparison operators, is the proposed idiom for doing these comparisons.
In this example, we are going to use compareTo()
Output
Code Explanation In the Above code we have used b1.compareTo(b2) to compare two BigDecimal. This method will return 0 if both are equal. This method will return 1 if b1>b2. This method will return -1 if b1<b2.
BigDecimal Rounding Behavior
The built-in Java method called BigDecimal.round(MathContext m) provides a BigDecimal value that has been rounded in accordance with the settings of the MathContext. There is no rounding if the accuracy parameter is set to 0. Syntax
Parameters The method only requires one parameter, m, which specifies the context to utilise, or the amount up to which the BigDecimal result should be rounded. Return The method's return value is a BigDecimal that has been rounded in accordance with the settings of the MathContext.
In Below Example we are going to check how to Round a BigDecimal.
Code Output
Code Explanation In the above code we have created a BigDecimal Object and called round() method with a precision of 4 by passing specified MathContext.
Conclusion
- The operations for arithmetic, scale manipulation, rounding, comparison, hashing, and format conversion are provided by the BigDecimal class.
- BigDecimal class offers total control over rounding behavior to the user.
- Features of BigDecimal are Scaling and Rounding Modes, No Overloaded Operators are allowed, use CompareTo() to compare BigDecimals not equals(), and BigDecimals are immutable.
- BigDecimal provides more accuracy for large float numbers.
- BigDecimal provides various methods to perform Mathematical operations, Rounding.