Reading Binary Files in Python
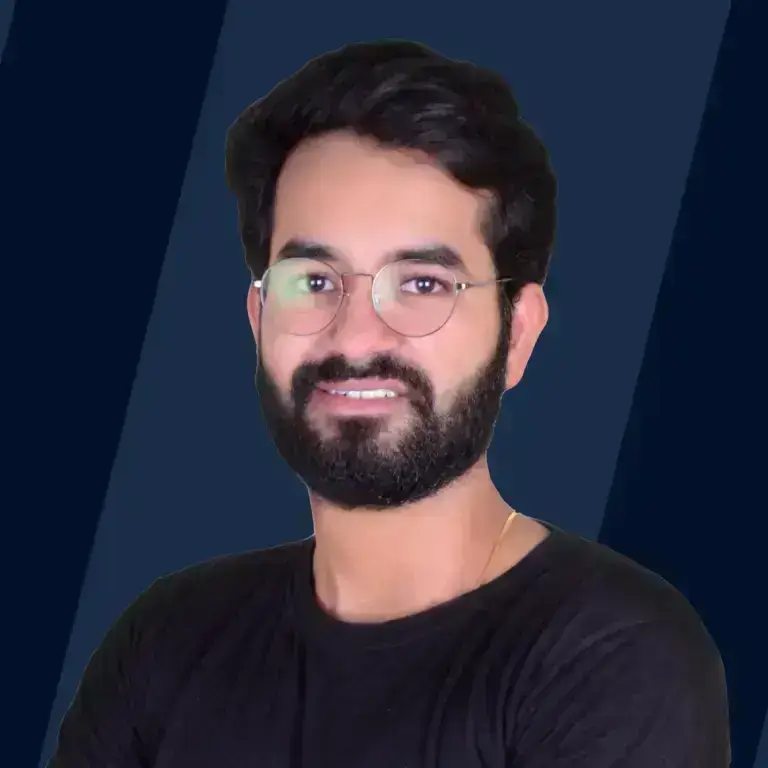
A computer file that includes data in a format made up of 0s and 1s is referred to as a binary file in Python. binary file in Python retains data in a more condensed and machine-readable format than text files, which store information in a format that is legible by humans. You can read and write raw binary data using Python's built-in open() function to communicate with binary files. Binary files are often read and written using the rb and wb modes, respectively. For operations such as managing photographs, films, or any other non-textual data, manipulating binary files is essential. When working with binary files, make sure you are precise to prevent data corruption.
Different file modes for Binary Files in Python
A binary file in Python is important in programming because they provide a different approach to handling data than standard text files. In contrast to text files, binary files use a sequence of bytes to represent information and store it in a format that isn't readable by humans. However, why is it necessary to delve into the complexities of Python's binary files?
Before we can solve this puzzle, let's examine what a binary file in Python is all about. A binary file is a type of Python storage that contains data in an unprocessed, machine-readable format. Binary files maintain the precise order of bits that make up the data, in contrast to text files that store information as a string of letters. Because of this feature, binary files are perfect for managing complex data structures such as images, videos, or executable programs.
What makes a binary file in Python preferable to text files, then? Versatility and efficiency hold the key to the solution. Because binary files do not require the overhead of encoding newline characters and characters, they take up less space than text files. Because of this, they are ideal for managing huge databases where every byte counts. Furthermore, binary files are flexible since they may represent any kind of data, including multimedia material and quantitative numbers.
Reading and writing raw bytes when working with binary files in Python allows for more precise control over data modification. When working with file formats that need accuracy, such as picture formats or proprietary data structures, this degree of control is essential.
Let's delve into the different file modes tailored for binary files in Python.
Reading Binary Files (rb):
When you have to read data from a binary file in Python, you should always use the rb mode. You can access the content in its raw binary form by using this mode, which guarantees that the file is opened in binary read mode. Using rb facilitates fluid reading operations, regardless of whether you're working with binary data—pictures, audio files, or anything else.
Writing Binary Files (wb):
On the other side, when you're ready to create or overwrite a binary file in Python, the wb mode comes into play. This mode ensures that the file is opened in binary write mode, letting you input raw binary data directly into the file.
Appending to Binary Files (ab):
The ab mode is used to append to a binary file without erasing any previous content. By opening a file in binary append mode, you can add additional binary data to the end of the file using this mode.
Reading and Writing Binary Files (r+b and w+b)
Use r+b for reading and updating current content and w+b for creating new files or overwriting existing ones if your program has to do both writing and reading operations on the same binary file.
Finally, exploring the world of binary file in Python reveals a robust toolkit for effectively managing a variety of complex and varied data types. Python programmers may fully use their programs, optimise storage, and guarantee seamless interaction with a variety of data sources by comprehending the significance of binary files.
Learning the specifics of Python's many file modes for binary files enables developers to work with a variety of binary data with ease. Python is a useful tool for manipulating binary data as it offers efficient and seamless interaction with binary files regardless of the mode you choose—whether you're reading, writing, or appending.
Reading Binary Files in Python
One of the most important programming skills that any Python developer should have is handling binary files. To traverse and handle binary data with ease, this article will take you through the fundamentals of reading binary files in Python.
Basic Method to Read a Binary File
Now let's begin by exploring the fundamental method of reading a binary file in Python. Using the open() method, you may enter the world of binary data. To make sure Python recognizes that you're working with binary data, use it in conjunction with the rb mode (read binary). Use the read() function to obtain the binary content once the file has been opened. To prevent resource leaks, don't forget to shut the file afterwards.
Reading Binary Data into a Byte Array
Now let's use byte arrays to our advantage and step up our programming.
Python's array module comes to the rescue, providing a dedicated array type for handling binary data efficiently. Create a byte array using the array module, specifying the 'B' type code for unsigned bytes. Read the binary data into this byte array using the fromfile() method, and you're all set to manipulate the data with ease.
Loading Binary File Data into an Array
Data from binary files can be advantageously loaded into a standard array for more complex applications. To do this, the numpy package provides an easy solution. If you haven't already, start by running pip install numpy to install numpy. After installation, import numpy and load binary data straight into a numpy array using the fromfile() method. This technique offers a flexible and effective way to handle binary data in a well-known array structure.
Reading a binary file in Pythonis a foundational ability that opens up a world of possibilities for working with various kinds of data. Gaining proficiency in both the fundamental approach and more complex methods such as byte arrays and numpy arrays will prepare you to handle situations requiring binary data in the real world. Take advantage of Python's capability to improve your programming abilities as you explore more about binary file manipulation.
Writing to a Binary File
Working with a binary file in Python is an essential skill in Python programming that leads to endless possibilities. Understanding how to write to a binary file is an essential part of your coding journey, regardless of whether you're working with sophisticated data structures, processing images, or just trying to maximize storage.
To begin our binary exploration, we must first open a file in binary mode. To do this, use the open() method and append b to the file mode. wb is a widely used mode for writing. This is an easy example:
Now that we have our binary file in Python open and ready, it's time to write some data. Python provides the write() method for this purpose. The write() method expects a bytes-like object as an argument. You can convert a string to bytes using the encode() method.
In this example, the string Hello, Binary World! is converted to bytes and then written to the binary file.
Managing binary files becomes more effective when working with custom objects, dictionaries, and other complicated data structures. The struct module in Python allows you to pack and unpack data in a binary format. Here's a snippet to give you a taste:
In this example, struct.pack() is used to pack an integer, a string of two bytes, and a float into a binary format before writing it to the file.
Remember that working with binary data gives up a wide range of options as we wrap up our investigation into writing to a binary file in Python. Understanding binary file management is an important coding ability, whether you're working with unique data structures, managing multimedia files, or optimising storage.
Conclusion
- binary file in Python, in essence, store data in a format that is not human-readable but is highly efficient for computers. Python provides robust methods to handle these files, optimizing performance and resource utilization in data-intensive applications.
- In Python, the built-in open() function acts as the gateway to binary files. Employing the right mode (rb for reading binary, wb for writing binary), developers gain direct access to the underlying binary data, paving the way for seamless interaction with files at the byte level.
- Navigation within a binary file in Python is an important aspect of efficient data processing. Python aids its developers with the seek() and tell() methods, enabling precise movement within the file. seek() allows positioning at a specific byte, while tell() reports the current position, offering fine-grained control over file exploration.
- Python simplifies the intricate process of reading and writing binary data with the read() and write() methods. These functions empower developers to extract data in chunks or inject new information directly into the binary stream. This granular control is invaluable when dealing with large datasets and complex file structures.
- Python's struct module emerges as a useful companion in handling binary data structures. This module makes it easier to convert between Python data types and packed binary data, whether you're processing binary data or building intricate structures. When used by developers seeking accuracy in data representation, it's a potent tool.
- When working with a binary file in Python, one must be alert to possible mistakes. Python has strong exception-handling techniques to promote traditional programming. Try-except blocks allow developers to handle errors gracefully, strengthening their code against unanticipated problems and guaranteeing binary file interactions.
- The final stroke in the binary file function series is the close() method. Often overlooked, this step is essential in freeing up system resources and preventing potential data corruption. When operations are finished, it's recommended practice to carefully shut the file to provide a neat and safe end to binary file manipulation in Python.