Binary To Decimal In Python
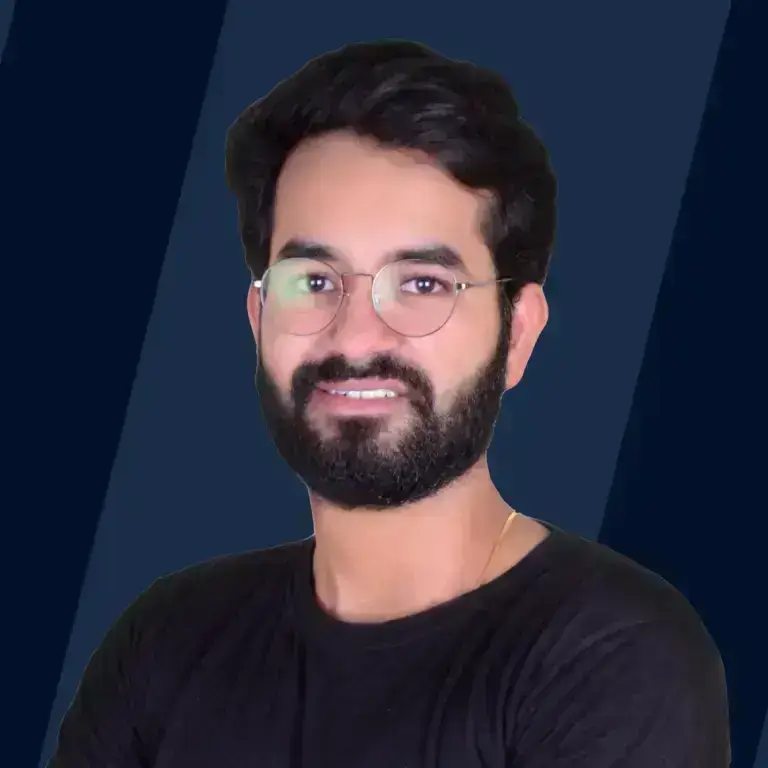
Overview
Binary Numbers is the number expressed in the base-2 numeral system or the binary numeral system which uses only two symbols 0 and 1. The decimal numeral system is the standard system for denoting integers ranging from 0 to 9.
How To Convert Binary To Decimal In Python?
Binary Number System
There are 4 available number system in our computer:
- Binary Number System
- Decimal Number System
- Octal Number System
- Hexadecimal Number System
The binary Number system is a number system which are expressed in the base-2 numeral system. They are represented by only 2 digits(1(one) or 0(zero)).
Example: (1010)2 is a binary number
Decimal Number System
The decimal Number system is also the part of number system. Decimal Numbers are integers ranging from 0 to 9. The decimal numbers are expressed in the base-10 numeral system.
Example: (5)10 or 5 is a decimal number.
Convert Binary Number to Decimal Number Let's consider a binary number (101)2, 101 in the decimal number is represented by 5.
Till now, we have seen that binary numbers are represented in the base of 2, and decimal numbers are represented by the base 10.
Steps:
-
Write down the binary number and list the powers of 2 from right to left.
Suppose we want to convert 101(binary number) to decimal i.e, 5. Now, write down the powers of two from right to left. Say , , and so on. Stop when the number of digits of a binary number is equal to number of elements of power of 2.
Example: 101 has 3 digits, so it will last for 3 elements that have the power of 2 i.e., 1, 2, 4.
-
Write the digits of the binary number below their corresponding powers of two.
Now, we have got our binary number and the power of 2 up to the given binary number i.e., for 101 we have 4, 2, and 1 as the power of 2's. So, now connect the right most element of the binary number "1" with the which is the minimum power of 2, and go on until the last element of binary.
Example:
-
Write down the final value of each power of two. Now, Connect the powers and binary digits together. Move through each digit of the binary number. If the digit is a 1, write its corresponding power of two below the line, under the digit. If the digit is a 0, write a 0 below the line, under the digit.
Example:
- In the previous step, we have multiplied all the numbers, now in the final step. Add the final values. Now, add up the numbers written below the line. Here's what you do: . This is the decimal equivalent of the binary number 101.
Examples Of Binary To Decimal In Python
Convert Binary Number to Decimal Number in python using loops
Input:
Output:
The above example shows that we have to convert the binary number to decimal number in python using loops(while loop).
Approach:
- Find the remainder of the given binary number.
- For every remainder multiply it with the power of 2.
- Store the result in the variable and add that variable with its previous value.
- Continue this until the given binary number is greater than 0(zero).
Output:
Binary Number to Decimal Number in python using Recursion
Input:
Output:
In the above example of converting binary number to decimal number in python using recursion.
Approach:
- For solving using recursion, we first need to identify the base case. In the case of binary form to decimal form, if the binary number is 0, then then the decimal number is also 0. So, our base case is if the binary number is 0, then it will return 0.
- Next step is if the binary number is not 0(zero), then we have to find the remainder of that binary number, and multiply that remainder with the power of 2.
- Now, add the remainder and call the recursive function
Output:
Binary To Decimal In Python Using int()
Input:
Output:
This example shows us how to convert binary number to decimal number in python using the inbuilt function int.
Syntax
Parameters: int(string, base)
string: string value that will be converted into an integer value.
base: base value like 2-binary form, 10-decimal number.
Output:
Conclusion
- Binary Number is the number expressed in the base-2 numeral system.
- Decimal Numbers are the numbers that are ranging from 0 to 9.
- We have to convert binary number to decimal number using the while loop, recursion method, and inbuilt int method available in python.