Bisect Python
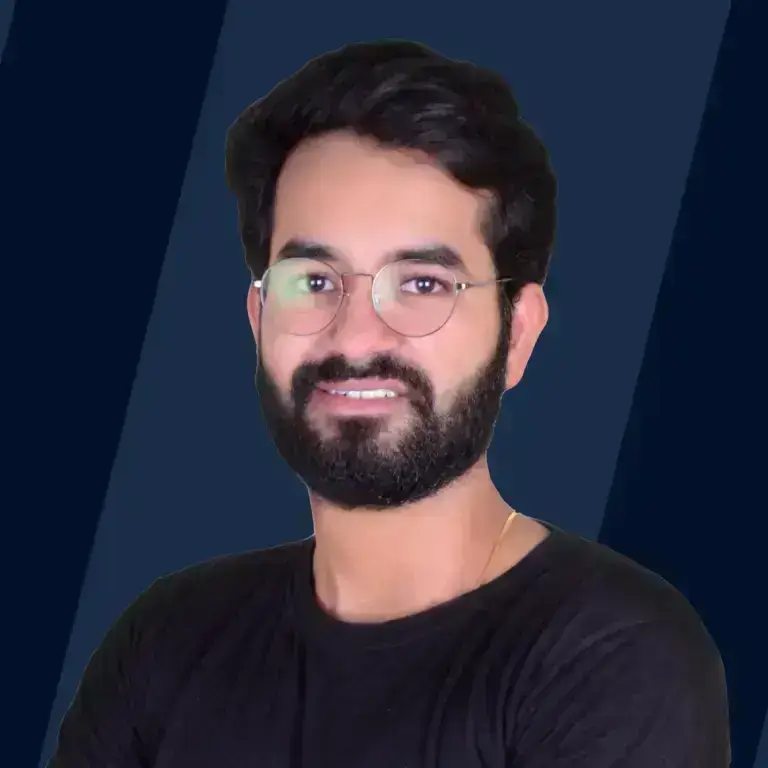
Overview
Bisect is a module in python which uses the bisection algorithm. By using this bisect module we can easily find a correct index position for a new element that has to be added to the already sorted list so that after adding the new element list will remain sorted. When the value that is to be inserted is already present in the sorted list, the functions of the bisect module still work. A suitable function, such as bisect left() or bisect right(), is used to locate an insertion point before or after any entries that already contain the same value. The behavior of the bisect() and bisect right() functions is the same.
Bisect Python Module
The Bisect algorithm's goal is to identify a location in the list in which a data item must be put to maintain the list in a sorted manner.
After inserting each data item, we can maintain the list's sorted order using the bisect algorithm. This is essential because it cuts down on the extra time needed to continually sort the list after inserting each data item. According to its definition, Python provides bisect algorithms through the bisect module. For example, the bisect function will return an index at which a new element can be placed in a way that ensures the list will remain sorted after the insertion.
Example
Output
As you can see in the diagram above 7 will get inserted in the 5th index of the list and the other item which is there at the 5th index will shift to the right index and so on.
Important Functions under Bisect Python Module
Let's explore some functions of the bisect module:
1. bisect(list, num, beg, end):- The index in the sorted list where the number can be placed to keep the output list in sorted order is returned by this function. The rightmost location where the element is to be inserted is returned if an element is already there in the list. This function requires four arguments: a list to work with, a number to insert, a starting position in the list, and a finishing position in the list. By default, the last 2 arguments are 0 and the last index of the list.
Syntax- bisect(list, num, beg, end)
2. bisect_left(list, num, beg, end):- The index in the sorted list where the passed number can be placed to keep the output list in sorted order is returned by this function. The leftmost location where the element is to be inserted is returned if the element is already present in the list. This function requires four arguments: a list to work with, a number to insert, a starting position in the list, and a finishing position in the list. By default, the last 2 arguments are 0 and the last index of the list. Syntax- bisect_left(list, num, beg, end)
3. bisect_right(list, num, beg, end) :- The bisect() function and this one operates similarly. Syntax- bisect_right(list, num, beg, end)
4. insert(list, num, beg, end):- This function provides the sorted list after inserting the number in the proper position, if an element is already present there in the list, then the element is inserted at the rightmost possible position. This function requires four arguments: a list to work with, a number to insert, a starting position in the list, and a finishing position in the list. By default, the last 2 arguments are 0 and the last index of the list. Syntax- insort(list, num, beg, end)
5. insort_left(list, num, beg, end):- This function provides the sorted list after inserting the number in the proper position, if an element is already present there in the list, then the element is inserted at the leftmost possible position. By default, the last 2 arguments are 0 and the last index of the list. This function requires four arguments: a list to work with, a number to insert, a starting position in the list, and a finishing position in the list. Syntax- insort_left(list, num, beg, end)
6. insort_right(list, num, beg, end) :- The "insort()" function and this one operates similarly. Syntax- insort_left(list, num, beg, end)
Inserting an Element into a List Using the Bisect Method
Output
In the above code the bisect() function is used to insert 40 as a new value inside the list, this function will find the rightmost appropriate index to insert 40 by which the sorted order of the list will not get disturbed.
Inserting an Element into a List from Left Using the Bisect Method
Output
In the above code the bisect_left() function is used to insert 40 as a new value inside the list, this function will find the leftmost appropriate index to insert 40 by which the sorted order of the list will not get disturbed.
Insort Method Bisect Python
Output
Important Examples to Understand Bisect Python Module
Example 1
Output
We have imported the necessary library bisect in the code sample above. Then, a list was created with some elements in it. Then we will insert 30 in this pre-sorted list using the bisect function, now this bisect is for the rightmost correct index where this 30 can be inserted so that the list will remain sorted.
Example 2
Output
We have imported the necessary library in the code sample above. Then, a list was made and some statements were printed. Following that, we inserted and printed the value using the bisect_left() method while specifying the list and number.
Example 3
Output
We have imported the necessary library in the code sample above. Then, a list was made and some statements were printed. Then, to insert and print the value, we used the bisect right() method, specifying the list and number.
Conclusion
- The Bisect module offers ways to change a list by adding components while maintaining the sort order.
- A significant amount of repetitious code that required sorting lists after changes has been removed.
- The official Python documentation claims that the bisect algorithm improves other widely-used methods, particularly when a list has a lot of members.