Booleans in Java
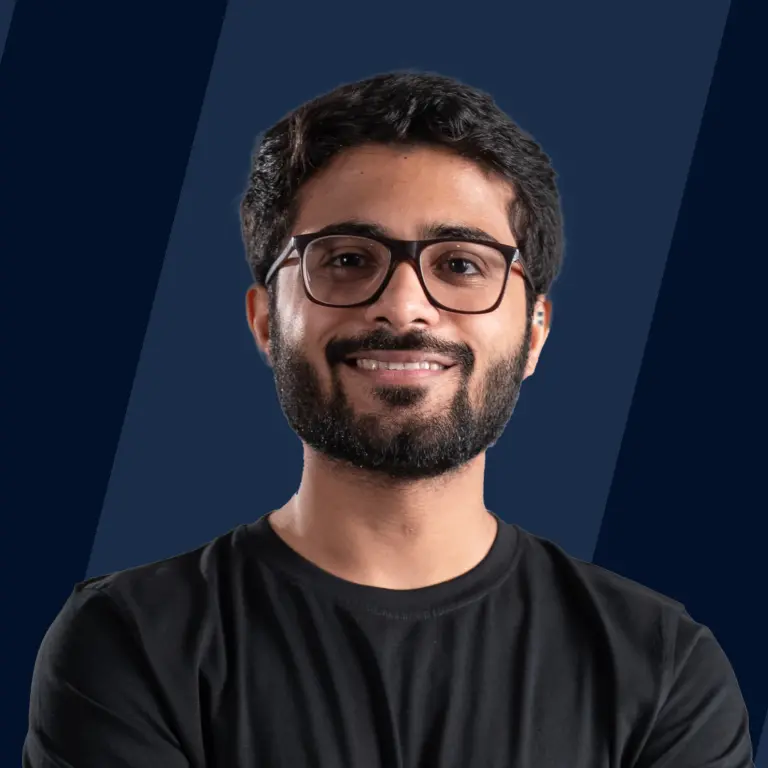
The Java Boolean class encapsulates the primitive boolean type, offering a way to treat boolean values as objects. This is crucial for collections like Lists and Maps that only accept objects. The class, part of java.lang, allows for operations like converting between boolean and String types, enriching Java's type system by bridging the gap between primitive types and object-oriented paradigms.
Boolean Values
In Java, boolean values represent two states: true or false. These are the only possible values for a boolean type, making it the most straightforward data type available in the language. Boolean values are extensively used in controlling program flow through conditional statements like if, while, and for loops, as well as in managing flags that signal the state of an application.
Examples Demonstrating Boolean Values
-
Simple Flag Usage
- Code:
- Output: The feature is active.
- Explanation: The boolean variable isActive is used as a flag to indicate whether a feature is active. The if statement checks the flag's state to decide which message to print.
- Code:
-
Result of a Relational Operation
- Code:
- Output: Is 10 greater than 5? true
- Explanation: The expression (10 > 5) directly evaluates to true, demonstrating how relational operations yield boolean values.
- Code:
-
Logical Operation Result
- Code:
- Output: Can access: false
- Explanation: This example uses logical AND (&&) to combine two boolean conditions. Since isAdmin is false, the combined condition canAccess also evaluates to false, reflecting the logical operation's outcome.
- Code:
-
Negation with Logical NOT
- Code:
- Output: Is not available: true
- Explanation: The logical NOT operator (!) is used to invert the value of isAvailable. Since isAvailable is false, negating it with ! results in true.
- Code:
Boolean Expressions
Boolean expressions in Java are fundamental constructs that evaluate to either true or false. These expressions are built using relational operators (like <, >, <=, >=, ==, !=) and logical operators (&&, ||, !). They form the backbone of control flow statements such as if, while, and for loops, allowing programs to make decisions and execute code based on certain conditions. Understanding how to construct and use these expressions is crucial for effective programming in Java.
Examples of Boolean Expressions
-
Relational Operator Example
- Code:
- Output: true
- Explanation: The expression a < b evaluates to true because the value of a (5) is indeed less than the value of b (10).
- Code:
-
Equality Operator Example
- Code:
- Output: false
- Explanation: The method x.equals(y) checks if the strings x and y are equal. Since "Java" is not equal to "Python", the output is false.
- Code:
-
Logical AND Operator Example
- Code:
- Output: false
- Explanation: The expression condition1 && condition2 uses the logical AND operator. It evaluates to false because condition2 is false, and for an AND operation, both operands must be true for the result to be true.
- Code:
-
Logical OR Operator Example
- Code:
- Output: true
- Explanation: The expression condition1 || condition2 uses the logical OR operator. It is evaluated as true because at least one of the operands (condition1) is true.
- Code:
-
Logical NOT Operator Example
- Code:
- Output: false
- Explanation: The expression !condition uses the logical NOT operator to invert the value of condition. Since condition is true, applying ! changes it to false.
- Code:
-
Combining Operators Example
- Code:
- Output: true
- Explanation: This expression combines relational and logical operators. (a < b) is true as 5 is less than 10. (condition || a == b) is also true because condition is true (the comparison a == b is false, but it's ignored due to the OR operator). Since both parts of the AND (&&) expression are true, the entire expression is evaluated to be true.
- Code:
Boolean in Java Examples
1. Simple Boolean Example
- Code:
- Output: Is Java fun? true
- Explanation: This is a straightforward example where a boolean variable isJavaFun is declared and initialized to true, indicating a positive statement about Java. The output directly reflects the value of the boolean.
2. Comparing Variables of Boolean Type
- Code:
- Output: Is Java as easy as Python? false
- Explanation: Here, two boolean variables isJavaEasy and isPythonEasy are compared using the equality operator (==). Since their values are not the same, the comparison yields false.
3. Method Returning Boolean Type
- Code:
- Output: Is 10 even? true
- Explanation: This example includes a method isEven that takes an integer as input and returns true if the number is even. The % operator checks if the remainder of number divided by 2 is 0, which is a characteristic of even numbers.
4. Finding a Prime Number
- Code:
- Output: Is 11 a prime number? true
- Explanation: This example features a method isPrime that determines whether a given number is prime. A prime number is greater than 1 and divisible only by 1 and itself. The method iterates from 2 to the square root of the number (to optimize performance) and checks for divisibility. If any divisor is found, it returns false; otherwise, it concludes the number is prime and returns true.
Conclusion
In this article, we learned about Boolean in java. Let us recap the points we discussed throughout the article:
- The Boolean class in Java is a wrapper for the primitive boolean type, enabling boolean values to be treated as objects. This feature is handy when working with Java collections like Lists and Maps, which require object types.
- Boolean values in Java are strictly binary, represented by two literals: true and false. This simplicity is fundamental to controlling program flow and managing application states through conditional statements and flags.
- Boolean expressions, constructed using relational and logical operators, are pivotal in Java programming. They enable decision-making in code, allowing for dynamic execution paths based on conditions evaluated at runtime.
- Through examples, we've seen the broad applicability of boolean values and expressions—from simple flag checks and condition evaluations to complex logic, such as determining if a number is prime.
- Using boolean values and expressions is integral to controlling the flow of Java programs. They dictate the execution of loops and conditional statements and are essential in error handling and state management.
- While the Boolean class adds object-oriented flexibility to boolean values, it's essential to be mindful of the trade-offs, such as potential overhead when boxing and unboxing between the boolean primitive and Boolean object types.
- Using boolean expressions and the Boolean class can significantly enhance the readability and maintainability of code by making conditions and states explicit and clear.