Java BufferedReader Class
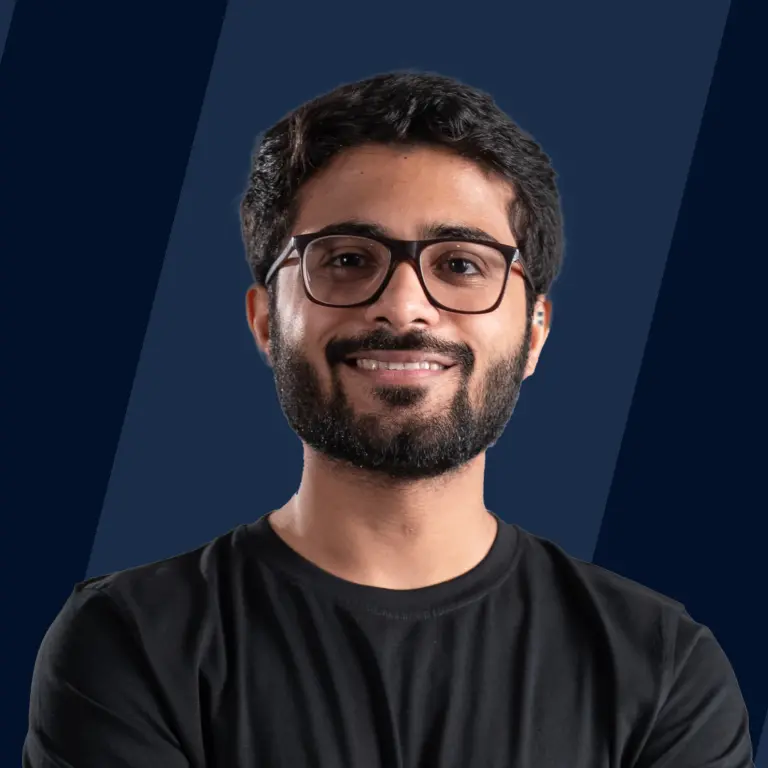
Overview
Reading data (in characters) from a file is commonly supported by many programming languages, and Java is no exception. But reading it more efficiently through Java is something every programmer should know. Java BufferedReader class, used with other readers, enables us to read data more efficiently. Let us move forward and explore it in this article.
Introduction to Java BufferedReader
BufferedReader class reads text from a character-input stream, buffering characters to provide for the efficient reading of characters, arrays, and lines. It is a part of the java.io package. We should consider the following points before using it:
- You can give a buffer size or use the default. The default is large enough for most purposes.
- It is advisable to wrap a BufferedReader around any Reader whose read() operations may be costly, such as FileReaders and InputStreamReaders.
Declaration
The Java BufferedReader class inherits the abstract Reader class because it is a specialization of the Reader class, but buffering is enabled. Which also reads text from a file that contains characters. It also provides a readLine() method which is commonly used for reading files line-by-line.
Working of BufferedReader Java
BufferedReader maintains an internal buffer of 8 KB (8192 bytes or characters), which is changeable. BufferedReader's read operation will store a chunk of characters read from the disk inside the internal buffer. Next, it would read these internal buffer characters individually. This process minimizes the number of I/O operations which are costly because of communication with the disk. Hence, it results in reading characters much faster and more efficiently.
Fields for Java BufferedReader Class
There is only one field present in Java BufferedReader class inherited from the abstract Reader class.
The use of this object is to synchronize operations on this stream.
Constructors of Java BufferedReader
Constructor | Description |
---|---|
BufferedReader(Reader in) | Creates a buffering character-input stream that uses a default-sized input buffer. |
BufferedReader(Reader in, int sz) | Creates a buffering character-input stream that uses an input buffer of the specified size. |
Constructors Details
We can create a Java BufferedReader class by importing the java.io.BufferedReader package.
a. Using the default buffer size
This creates a new BufferedReader object with a default buffer size of 8 KB.
b. Using a custom buffer size
This creates a new BufferedReader object with a custom buffer size of 4 KB.
Methods of Java BufferedReader
Modifier and Type | Method | Description |
---|---|---|
void | close() | Closes the stream and releases any system resources associated with it. |
Stream<String> | lines() | Returns a Stream, the elements of which are lines read from this BufferedReader. |
void | mark(int readAheadLimit) | Marks the present position in the stream. |
boolean | markSupported() | Tells whether this stream supports the mark() operation, which it does. |
int | read() | Reads a single character. |
int | read(char[] cbuf, int off, int len) | Reads characters into a portion of an array. |
String | readLine() | Reads a line of text. |
boolean | ready() | Tells whether this stream is ready to be read. |
void | reset() | Resets the stream to the most recent mark. |
long | skip(long n) | Skips characters. |
Methods Details
a. Using read() Method
This method implements the general contract of the corresponding read method of the Reader class. As an additional convenience, it attempts to read as many characters as possible by repeatedly invoking the read method of the underlying stream. This iterated read continues until one of the following conditions becomes true:
- The specified number of characters have been read,
- The read method of the underlying stream returns -1, indicating end-of-file, or
- The ready method of the underlying stream returns false, indicating that further input requests would block.
If the first read on the underlying stream returns -1 to indicate end-of-file then this method returns -1. Otherwise, this method returns the number of characters actually read.
The read() method can be called in the following ways:
- read() - Reads a single character
- read(char[] array) - Stores the characters read from the reader into the passed array
- read(char[] array, int start, int length) - Reads the length no of characters from the reader starting from the position start and stores it in the given array
Example: Consider a file named input.txt which contains the following text:
Output:
Explanation: In this example, we create a method that uses the BufferedReader Java class' read method to read a file's contents into an array. The BufferedReader's read method can throw an IOException hence we add a throws keyword to the method containing the logic to read the file. We start by creating an array that will store the file's contents. A BufferedReader wraps an object of a Reader which is a FileReader in this case. An object of FileReader is created by passing the file's location to its constructor. Then, we use the object in the constructor call of BufferedReader. read method is invoked after the object is created. It accepts an array that will hold the file contents on a successful read operation. We close the reader object when it's no longer needed. The returned array is then utilized in the main method to print the file contents on the console.
b. Skipping Characters
A certain number of characters can be skipped (ignored) to be read by using the skip(long n) method. The below example shows how.
Example:
Output:
Explanation: The above example uses a StringReader that creates a string source from where we read the characters individually. The BufferedReader Java class is flexible enough and can be attached to any character reader. So we again make use of BufferedReader by wrapping it around the StringReader object created from a string that contains a number character after every three characters. To read only numerical characters, we use the skip(long n) method to skip three characters after reading each number character. We read the characters until we reach the end of the string, which is detected when the read method returns -1 (which signifies end-of-file). We close the reader object when it's no longer needed. The result obtained is a string of numerical characters.
Java BufferedReader Class Examples
a. Reading Data from a File via the readLine() method
The readLine() method reads a line of text. A line is considered to be terminated by any one of a line feed ('\n'), a carriage return ('\r'), or a carriage return followed immediately by a linefeed. It returns a string containing the contents of the line, not including any line-termination characters, or null if the end of the stream has been reached.
Example: Consider a sample input.txt file which contains the following two lines:
Output:
Explanation: In this example, we use the readLine() method to read file line by line. We create the FileReader object wrapped inside the object of the BufferedReader Java class by passing it in the constructor. We use a while loop to keep reading every line of the file (excluding new line characters) until we obtain a null value which signifies no more lines to read. A string is prepared by concatenating each line with the previous one. At last, we return the resulting string to the main method for printing on the console. We close the reader object when it's no longer needed. Notice how the two lines print on the same line after reading via the readLine() method.
b. Reading Data From Console By InputStreamReader And BufferedReader in Java
Output:
Explanation: Another example shows the flexible nature of the BufferedReader Java class. It can also work in conjunction with an InputStreamReader object. We connect to an input device, such as a keyboard, by creating an object of InputStreamReader through a constructor that takes in an InputStream like the standard input stream System.in. Then, we create a BufferedReader object through the newly created object of InputStream. Next, we prompt the user to enter some string on the console that we read via the readline() method. Finally, we display the entered string by the user and close the reader object when it's no longer needed.
Difference Between Scanner And BufferedReader
BufferedReader | Scanner |
---|---|
BufferedReader is synchronized (thread-safe) | Scanner is not synchronized |
BufferedReader uses buffering to read a sequence of characters from a character-input stream | Scanner can parse primitive types and strings using regular expressions |
BufferedReader allows changing the size of the buffer | Scanner has a fixed buffer size |
BufferedReader has a larger default buffer size (8 KB) | Scanner has a smaller buffer size (1 KB) |
BufferedReader explicitly throws IOException and forces us to handle it | Scanner hides IOException |
BufferedReader is faster and efficient | Scanner is slower and less efficient |
No ambiguity related to nextLine() method | There are problems with nextLine() method |
Conclusion
- BufferedReader Java class simplifies reading text from a character-input stream. It inherits the abstract class Reader.
- It has a default buffer size of 8 KB. But this size can be modified by using one of its overloaded constructors.
- It maintains an internal character buffer to minimize the number of I/O operations which enables efficient and faster reading.
- BufferedReader Java class provides flexibility to programmers by enabling connection with the available Reader implementations.
- It provides various methods like read(), readLine(), skip(), mark(), reset(), etc.
- The read() method is used to read one character at a time.
- The readLine() method is used to read a line without including the new line character.
- The skip() method is used to discard or skip a given number of characters.
- BufferedReader Java class can be used in a multi-threaded environment because it is synchronized.