Python bytes() Function
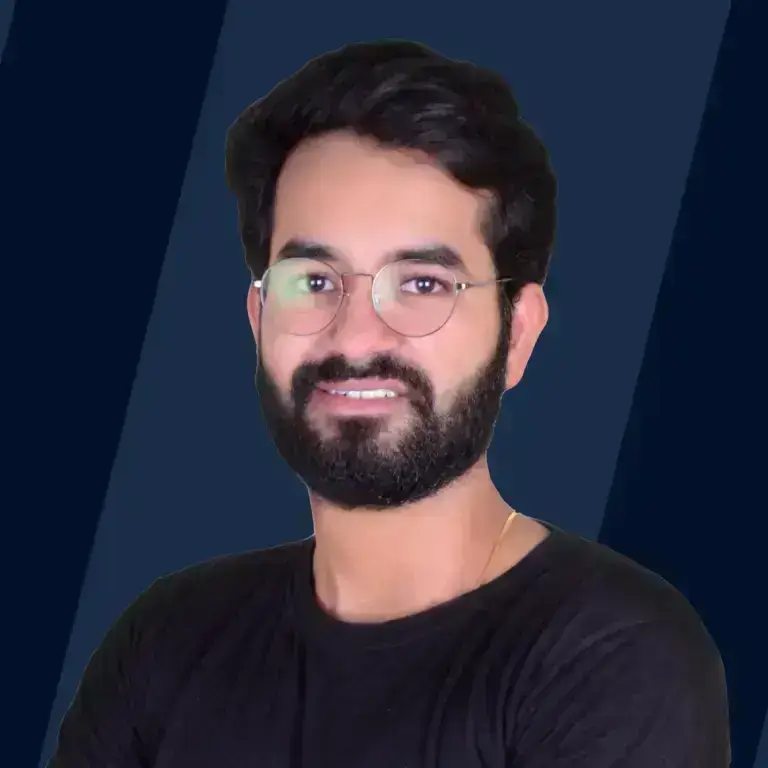
Overview
The bytes Python function is essential for working with binary data. It allows for creating immutable sequences of bytes from various data types, like strings or integers. This function is pivotal in file I/O or network connections, demonstrating Python's capability in handling binary data.
Syntax of Python bytes() Function
The bytes Python function is fundamental for handling binary data. It's crucial to grasp its syntax to harness its power effectively.
The syntax is straightforward:
Parameters of Python bytes() Function
The parameters of the bytes Python function are as follows:
- source (optional): This parameter allows the developer to specify the source of data that a developer wants to convert into bytes. It can be a string, iterable, or a buffer-like object.
- encoding (optional): When the source is a string, the developer can provide the encoding parameter to specify the character encoding used for the conversion. Common encodings include 'utf-8' and 'ascii'.
- errors (optional): If encoding errors occur during the conversion, the developer can control their handling by setting the errors parameter. Options include strict, ignore, and replace.
Return Value of Python bytes() Function
The return value of the bytes Python function is simple: it returns a new, unchanged bytes object holding the binary representation of the supplied data. This function is handy for converting text to bytes or manipulating binary data.
Exceptions of Python bytes() Function
-
The bytes Python function expects its input to be either an iterable of integers in the range 0-255 or a single integer. Passing any other data types will result in a TypeError. It will also be thrown if a developer attempts to build a bytes object with non-integer components or float values.
-
The iterable integers must be inside the provided range. Extending the range will result in a ValueError.
-
The bytes() function does not support mixed-type iterables, so if a developer tries to mix integers and non-integers, it will raise a TypeError.
How does the Python bytes() Function work?
You can pass it a string, a sequence of integers, or an iterable of integers ranging from 0 to 255. When you pass a string, you specify the encoding to convert the characters into bytes. For example, bytes("hello", "utf-8") would encode the string "hello" into bytes using the UTF-8 encoding.
Passing a sequence of integers creates a byte object where each integer represents a byte. For instance, bytes([65, 66, 67]) would create a bytes object with the bytes b'A', b'B', and b'C'.
Examples
Let us now take some examples to understand the usage of the bytes() function for more clarity.
1. Creating Bytes from a String:
Output
Explanation:
- In this example, a string text is defined as "Hello, Python!".
- The bytes() function converts the string into bytes, specifying the encoding as 'utf-8'. This encoding ensures that the string is converted into a sequence of bytes representing the characters in UTF-8 encoding.
- The resulting byte_data contains the bytes representation of the string.
2. Converting a List of Integers to Bytes:
Output
Explanation:
- Here, a list of integers is defined, containing the values [65, 66, 67, 68].
- The bytes() function is used again, but without specifying an encoding. It directly converts the list of integers into a byte object where each integer corresponds to a byte.
- The byte_data contains the bytes representation of the integers.
3. Extracting Bytes from a File:
Output
Explanation:
- This example involves reading binary data from a file named 'binary_file.bin'.
- The open() function is used with the 'rb' mode to open the file in binary read mode.
- file.read() reads the binary data from the file, and the bytes() function converts it into a bytes object.
- The resulting byte_data contains the bytes read from the file, which depends on the actual content of 'binary_file.bin'.
- The output will vary based on the content of the file.
The bytes Python function allows you to work easily with binary data in Python, making it a useful tool for various applications. Whether you're managing network packets or reading binary files, understanding its capabilities may improve your coding skills.
Conclusion
- The bytes Python function is a flexible tool for converting data types such as texts, integers, and byte sequences.
- Because Bytes objects are immutable, their values cannot be modified after creation. They are appropriate for circumstances where data integrity is critical.
- Bytes may be readily encoded into or decoded from strings using character encodings such as UTF-8 or ASCII, allowing for smooth data handling.
- Because bytes are often employed for low-level binary operations and binary data handling, they are critical in file I/O, network communication, and cryptography.
- Python's bytes() function enables efficient binary data storage and processing, ensuring that your programs utilize memory resources sensibly when working with raw data.
See Also
Please check out other functions of Python like: