C Dereference Pointer
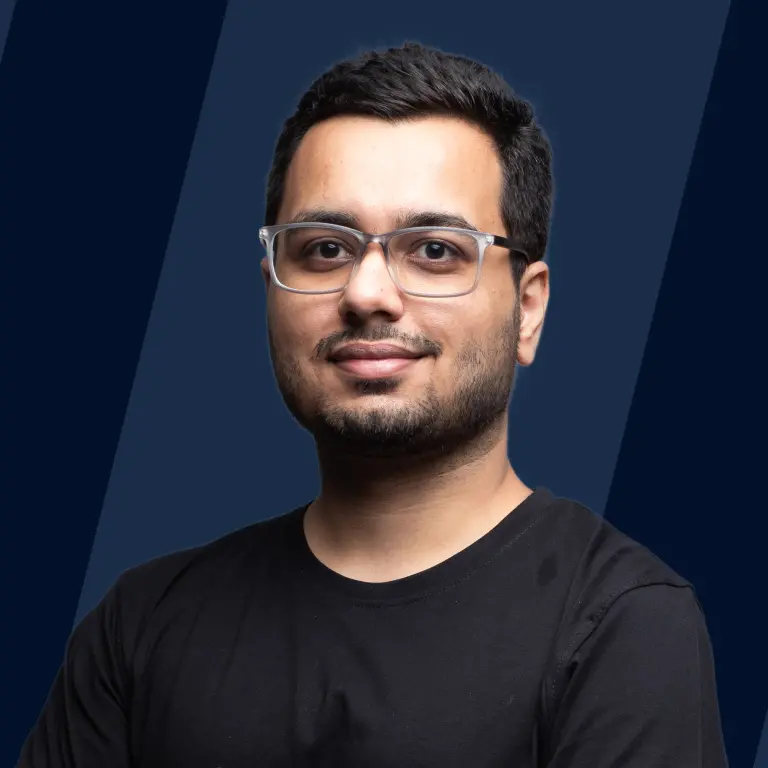
How to Dereference Pointer in C?
What is a Pointer?
A pointer is a special variable that is used to store the memory address of another variable (which is of the same data type as the pointer) as its value rather than storing the direct values. A pointer variable stores the value of another variable of the same data type, for example, if the variable is of int data type, then the pointer which will store the memory address of this variable is also of the int data type. In C language, a pointer can be declared using the * (asterisk) operator. By using this operator, the address of the variable (on which we are working) is assigned to the pointer variable.
What is Dereferencing?
Dereferencing is used to access or find out the data which is contained in the memory location which is pointed by the pointer. The * (asterisk) operator which is also known as the C dereference pointer is used with the pointer variable to dereference the pointer. When we dereference a pointer, the data of the variable which is pointed by this pointer is returned.
How to Dereference a Pointer?
The dereferencing of a pointer is done by using the * (asterisk) symbol in front of the pointer making it a C dereference pointer.
Output
Steps to dereference the pointer
The above code can be understood by the following steps, in which we have created a C dereference pointer and dereferenced the pointer.
- First, we created simple int variables x and deref, and initialized x with an integer 19.
- Then we created a pointer ptr, and assigned the address of x to this pointer by using the & which is known as the address of operator.
- Finally, we dereferenced the ptr pointer by using the * (asterisk) operator (which is also known as the dereferencing operator) and we put the value of this pointer in the deref variable.
Why do We Dereference a Pointer in C?
Below are the reasons for which we dereference a pointer in C
- It can be used to access or find the data of the variable which is stored at the memory address of the pointer which reduces the storage space and complexity of the program.
- Any operation which is applied to the dereferenced pointer will directly affect the value of the variable whose address is stored in the pointer, so it enhances the execution speed of a program because now we do not need to make changes in the original variables.
Examples
Creating a C Dereference Pointer of Integer Data Type
Output
In the above example, we can see the case of int data type. In this case, we created a variable of int data type and we created the pointer variable which is also of int data type. Then we dereferenced the pointer using the steps shown above and finally accessed the data of the x variable.
Creating a C Dereference Pointer of Character Data Type
Output
In the above example, we can see the case of the char data type. In this case, we created a variable of char data type and we created the pointer variable which is also of char data type. Then we dereferenced the pointer using the steps shown above and finally accessed the data of the ch variable.
Creating a C Dereference Pointer of Float Data Type
Output
In the above example, we can see the case of the float data type. In this case, we created a variable of float data type and we created the pointer variable which is also of float data type. Then we dereferenced the pointer using the steps shown above and finally accessed the data of the num variable.
Creating a C Dereference Pointer of Double Pointer
Output
In the above example, we can see the case of double pointer dereferencing with the help of int data type. This is a special case in the topic of pointers, which is also known as pointer to pointer. This is because the first pointer stores the address of the variable, and the second pointer stores the address of the first pointer. So if we use ** (double asterisk) operator, it will first dereference the first pointer, and then dereference the second pointer.
Conclusion
This quick tutorial was about the dereferencing of a pointer in C, and we can conclude the following
- The dereferencing of the pointer in C is used to extract the data stored in the address of that pointer.
- We can also use double pointer in order to store the address of another pointer, and for extracting the data of the double-pointer, we have to use double dereferencing.
Frequently Asked Questions (FAQs)
Q: Can I dereference a null pointer in C?
A: No, it is not safe to dereference a null pointer in C. Dereferencing a null pointer leads to undefined behavior and can result in crashes or unexpected program behavior. Always ensure that a pointer is valid and points to a valid memory location before dereferencing it.
Q: Can we store the address of the variable in the pointer of a data type that is different from the data type of the variable?
A: No, we cannot store the address of the variable in the pointer of a data type that is different from the data type of the variable. This is because the pointer stores the address of the variable, and the memory allocated to the variable is according to the data type of the variable. So the pointer is also required to be of the same data type because the size of the pointer must be of the same size as that of the variable.
Q: How can I modify the value of a variable through a dereferenced pointer?
A: To modify the value of a variable through a dereferenced pointer, you assign a new value to the dereferenced pointer. For example, if 'ptr' is a pointer to an integer, '*ptr = 42;' assigns the value 42 to the memory location pointed to by 'ptr', effectively modifying the original variable.
Q: Can I dereference a pointer to a structure in C?
A: Yes, you can dereference a pointer to a structure in C. By dereferencing the pointer, you gain access to the individual members of the structure, allowing you to read or modify their values using the dot operator (e.g., '(*ptr).member' or 'ptr->member').
To learn more about Pointers in C, visit the article Pointers in C