What are C Expressions?
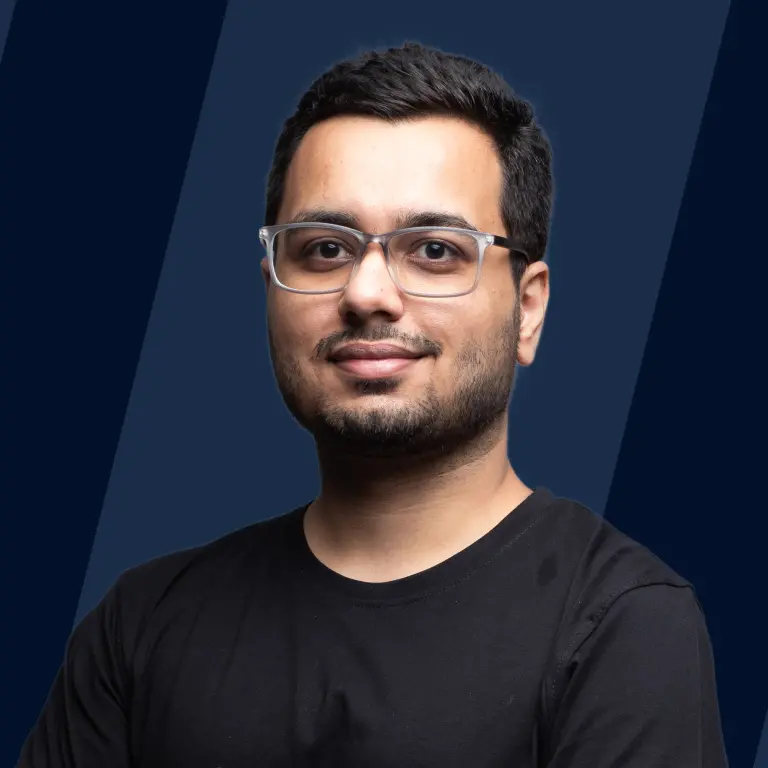
A C expression is a union of operators and operands. A C expression is used to calculate a single value that is saved in a variable. The action or operation to be carried out is indicated by the operator. The objects to which we apply the operation are known as operands.
The below diagram is an example of how a C expression looks like using the variable, operator, and operands:
Ways of Writing an Expression in C
The C Expressions can be defined by the number of operands, operators, and their positions. Discussed below are the six ways of writing an expression in the C Language:
a. Infix
When the operator lies between the operands then the expression is known as an Infix expression. Below is the representation:
operator lies between the two operands and .
and operator lies between the operands, so it is also an infix expression.
b. Postfix
When the operator lies after the operands then the expression is known as a Postfix expression. Below is the representation:
operator lies after the two operands and .
c. Prefix
When the operator lies before the operands then the expression is known as a Prefix expression. Below is the representation:
operator lies before the two operands and .
Note: Postfix and Prefix expressions can't be used directly while coding a program. These expressions are generally used by the compilers during the compilation process of a program as there is no issue of operator precedence or associativity with postfix and prefix expressions.
d. Unary
When the expression contains only one operand and one operator then the expression is known as a Unary expression. Below is the representation:
++ and -- are the increment and decrement operators that can lie before and after a single operand in a unary expression. We will see more about it later in the article using a C program.
e. Binary
When the expression contains two operands and only one operator then the expression is known as a Binary expression. Below is the representation:
An operator from +, -, *, /, %, etc. operators can lie between the operands in a Binary expression. We will see more about it later in the article using a C program.
f. Ternary
When the expression contains three operands and two operators then the expression is known as a Ternary expression. We use the ternary operator () in the ternary/conditional expressions, below is the representation:
The above ternary expression is evaluated as, if is greater than , then assign to , else assign to .
Example C Program:
Below is the C program to demonstrate the use of the above-mentioned ways of writing an expression:
Input:
Output:
Explanation: In the above C program, we have demonstrated how we can use the unary expression, binary expression, infix expression, and ternary expression. Postfix and Prefix expressions can't be used directly while coding a program, these expressions are generally used by the compilers during the compilation process of a program.
Types of Expressions
The C Expression can be classified into various types. Discussed below are the six types of expressions in the C Language:
1. Arithmetic Expression
Arithmetic expressions are used to compute the values of the int, float, or double data type variables and consist of the arithmetic operators Addition (+), Subtraction (-), Multiplication (*), and Division (/). Below is an illustration of the arithmetic expression:
Addition, Multiplication, Subtraction, and Division operation is performed on the operands and the resultant value will be stored in the variable.
2. Relational Expression
Relational expressions are used to compare two values and returns a boolean value, i.e., true () or false (). Relational expressions are made using a relational operator like greater than (>), smaller than (<), greater than or equal to (>=), smaller than (<=), equal to (==), and not equal to (!=). Below is an illustration of the relational expression:
If , true will be stored in the variable, else it will store false. Similarly, and variables will store true or false based on the relational expression validation.
3. Logical Expression
Logical expressions are generally used to combine two relational expressions and return a boolean value. Logical expressions are made using logical operators like AND (&&), OR (||), and NOT (!). Below are the tables to show the results of AND, OR, and NOT operations.
- AND operation Table
A | B | OUTPUT |
---|---|---|
false | false | false |
false | true | false |
true | false | false |
true | true | true |
- OR operation table
A | B | OUTPUT |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | true |
- NOT operation table
A | OUTPUT |
---|---|
false | true |
true | false |
Below is an illustration of the logical expressions:
Let's suppose () is true and () is false, then
4. Conditional Expression
Conditional expressions are used to execute/return a particular set of instructions based on an expression validation. Conditional expressions are made using a conditional operator (?,:). Below is an illustration of the conditional expression:
The above conditional expression is evaluated as, if is smaller than , then assign to , else assign to .
5. Pointer Expression
Pointer expressions are used to get the address of variables stored in the system's memory. Pointer expressions can be made using an ampersand operator (&), and an asterisk (*) Below is an illustration of the pointer expression:
*ptr = &arr
The above pointer expression is evaluated as the address of the arr variable will be stored in the ptr pointer variable.
6. Bitwise Expression
Bitwise expressions are used to perform operations at the bit level. Bitwise expressions are made using bitwise operators like Right Shift (>>), Left Shift <<, Complement ~, AND &, OR |, and XOR ^ Below is an illustration of the bitwise expression:
The above bitwise expression is evaluated by converting the values of the operands into bits and applying the bitwise AND, OR, XOR, etc. operations from the left side of the converted bit values.
Note: For more information on each operator check out the related articles mentioned in the next section.
Example C Program:
Below is the C program to demonstrate the above-mentioned types of expression:
Output:
Explanation: In the above C program, we have declared six variables to store the results obtained from various expressions. The result of the arithmetic expression is stored in an int a variable, relational expression in a bool b variable, logical expression in a bool c variable, conditional expression in a char d variable, and pointer expression in an int e variable, and bitwise expression in an int f variable. We have printed the values of these variables in the output.
Related articles
Conclusion
- A C expression is a union of operators and operands, and it is used to calculate a single value that is saved in a variable.
- A C Expressions can be defined by the number of operands, operators, and their positions.
- There are six ways of writing an expression in programming in general, Infix, Postfix, Prefix, Unary, Binary, and Ternary.
- There are six types of expressions in C, Arithmetic, Relational, Logical, Conditional, Pointer, and Bitwise expression.