What is the Extern Keyword in C?
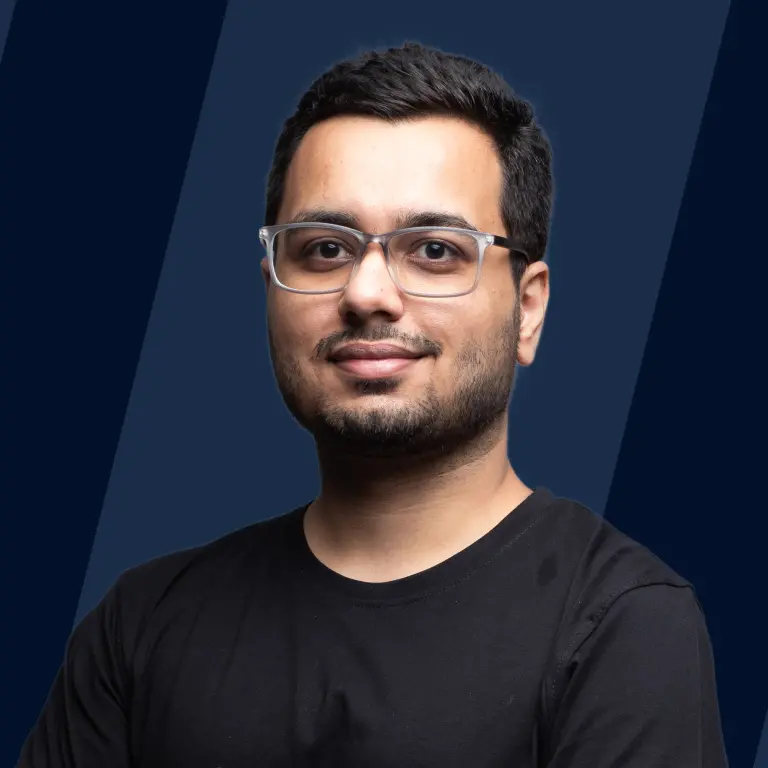
Introduction
The extern keyword in C is used to declare a variable as a global variable such that a variable declared in another scope of the same file or another file can be accessed from anywhere in the program. The variables or functions declared with the extern keyword in C will be linked externally(external linkage).
Declaration
It is important to note that the C extern keyword only declares a variable and does not define a variable.
The C extern keyword declares global variables, mostly in the header files used in other files of a long program.
Declaring a variable implies that the variable is introduced to the compiler along with the data type of the variable. Defining a variable implies that the compiler allocates the storage required for the variable A variable definition can also be considered as a declaration, but only in some cases.
Syntax
The extern keyword is used before the data type of a variable or the return data type of a function. The syntax of using the extern keyword in C is,
Uses of C extern keyword
The best use case of the C extern keyword is to declare functions or variables as global and to use them in different scopes from where they are defined.
Examples
The following example illustrates how the extern keyword is used to declare a global variable,
In the above example, the line extern int var is used to declare the variable var. At this time, no space is allocated by the compiler for the variable, var. At the line, int var=10, the variable is defined and declared again, but the compiler knows that the variable var has already been declared by using the extern keyword, therefore the compiler only defines the predeclared var variable with the value of 10. Now, the variable is a global variable and can be used from any part of the program.
Let us consider another example containing two files that have the definition and declaration of a variable using the extern keyword,
Let the file, variables.c has a variable called extern_var that has been defined,
To use the variable extern_var present in variables.c in main.c, we can declare the variable using the extern keyword,
The output of the above code is,
The "#include" syntax is used to link global data from one file to another.
Instead of following this syntax, we can remove the #include "variables.c" line from the code and link the files manually during compiling by using the following command,
What is C Extern Variable?
Introduction
As we have seen in the above section, the C extern variable is a global variable created with the extern keyword. The data type of the variable can't be changed after declaring the variable.
Uses of C Extern Variable
The main use cases of the extern variable are,
- Access a variable defined in another file.
- Create a global common variable that can be used in all files that are linked together.
Examples
The following example illustrates how the C extern keyword is used,
Let us consider a program in which there are multiple functions in different files that modify a common variable, The globals.h file is the header file and can have all the global variables,
The file named changer.c defines the extern variable and has functions to update the global variable,
The main file,main.c can also access the global variable and modify it,
Note: Only the main.c file has to be compiled to run the entire program.
The output of the above code will be,
In the above example, the variable common_variable is made declared as an extern variable and is made accessible from both files.
It is interesting to note that, the function increment() is used in the main.c file even though the function has not been declared using the C extern keyword. We will look at the reason for this in the function section of the article.
What is C Extern Function?
Introduction
A C extern function is a global function that can be declared in a file and called from another file.
Syntax
The syntax to declare a C extern function is,
We have seen in the last section about the function, void increment(){}, which was called from another file even though the function has not been declared using the extern keyword. This is because functions are always handled as global by the compiler, so there is no need to use the C extern keyword before a function. The compiler will intercept the increment() function as,
Uses of C Extern Function
The C extern function has the following use cases,
- The function can be called from any linked part of the program.
- Creating libraries in C which has many functions that can be used by importing the necessary libraries.
Examples
The following example contains a file with C extern function definition, and the function is called in another file.
The file, functions.c has the function declaration,
The main.c file from which the function is called is,
We will get the same results even if we remove the extern keyword from the functions defined in functions.c.
The output of the above program is,
FAQs
Q: How to resolve the undefined reference to error in programs using an extern and what is the cause for the error?
A: The undefined reference to error occurs when a variable is declared using an extern but not defined later or when the file in which the variable's definition is present is not included in the program.
To resolve the above error, check the #include "file_name" section of the code, and if the code is linked manually, check if you have included the extern keyword for variables.
Related articles
- Functions are used to increase the reusability of code and make debugging easy. Learn more about functions in C.
- The static keyword performs the exact opposite functions of the extern keyword. Learn more about static keyword in C.
Conclusion
- The C extern keyword is used to extend the scope of variables throughout the linked parts of the program.
- Global variables can be created and used using the C extern keyword.
- By default, functions are treated as extern functions by the compiler.
- It is best practice to declare all the extern variables and functions in the header part of the program.