What is the && Operator in C?
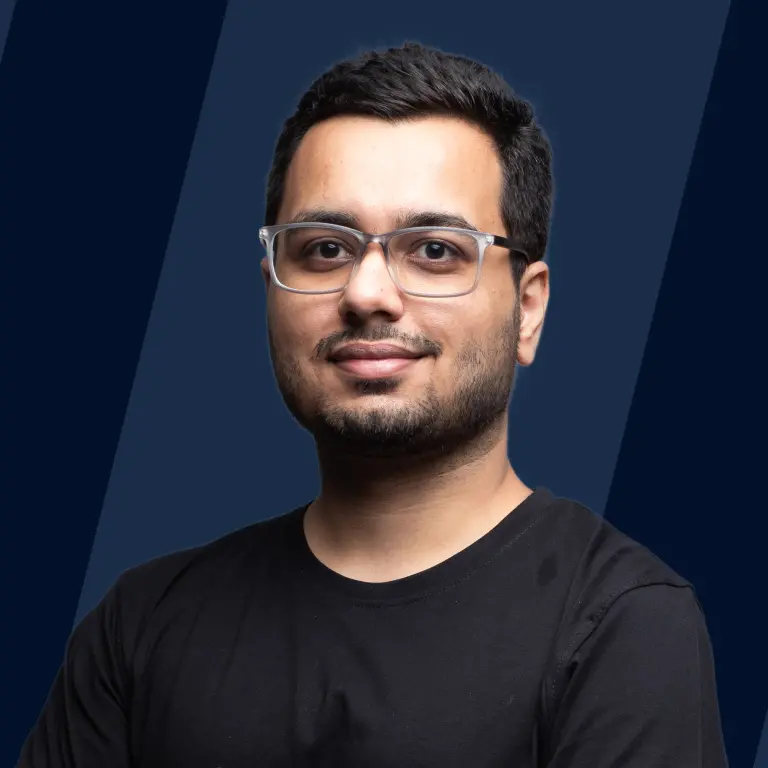
In real life, we often face a situation where the truth of a sentence depends upon more than one case or situation. For example, to get admission to the IITs, one must have cleared the minimum cut-off of each Maths, Physics, and Chemistry subject individually as well as the minimum total marks cut-off, so the true value of taking admission in IITs depends on four conditions.
Similarly, the ‘&&’ operator or double ampersand operator is a logical AND operator in C language. The ‘&&’ operator combines and checks two or more conditions or logic to see whether they are true or not. The logical expression may return 1 representing true or 0 representing the whole expression is false.
Logical AND (&&) Operator in C
While checking the conditions for the if-else cases, switch cases, etc, a programmer could need to check more than one situation combined. The programmer needs to run a code block only if conditions A and B are true; otherwise, they are not. Then, the Logical AND or ‘&&’ operator may combine the results of both conditions and give the final result.
In C language, there are three types of logical operators; all three return either 1, which indicates true, or returns 0, which indicates false. These logical operators are mainly used to get the combined result of two or more conditions.
A logical AND (&&) operator is a binary operator that takes two operands and gives the combined result. If both the operands are true, it will return true or 1; otherwise, if anyone is false, it will return false or 0.
Syntax
&& operator in C is used in two ways first, to combine two expressions, and other is just an extended version of the previous one. We combine more than two expressions using the && operator in C. Let’s check the syntax:
When there are only two operands there to be checked combined:
When there are more than two expressions that need to be combined:
Truth Table of Logical AND Operator
Until now, we have seen the basics of the && operator in C, along with its syntax of it. Now, let’s move to another topic:
The truth table shows the possible answers to different situations a logical operator can produce. Let’s see the truth table for the logical AND(&&) operator with two different operands or expressions.
Experssion 1 (A) | Expression 2 (B) | Result (A && B) |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
From the above table, we can conclude that the logical AND(&&) operator returns true only in one case when all the given conditions are true; otherwise, it will return false.
Operator Keyword for &&
The && operator in C has a few unique symbols. This is because & is a bitwise AND operator in C, whereas && is a logical AND operator in C, and they may often confuse beginners. Also, as we have seen in the above article about the logical AND operator, it works exactly like its name, ‘AND’, which means if conditions A and B are true, the final result will be true; otherwise, it will be false.
In the <iso646.h> library of C programming language, a macro, ‘and’, is defined as an alternative to the ‘&&’ operator. The best reason behind this is that it is simpler to remember. Also, in C++ programming language, ‘and’ is defined in both the <iso646.h>and<ciso646>` libraries.
Examples
- A simple example to show every case discussed in the truth table for the logical AND operator.
Code:
Output:
Explanation:
In the above code, we have taken two integer variables and assigned them values true(1) and false(0). After that, we have shown all the possible cases (which we have seen in the truth table). First, we used the ‘&&’ operator in C for two true values. We used the ‘&&’ operator in C for the true-false and false-true combination, and at last, we printed the result of the false-false combination using the && operator in C.
- In this case, we will take more than two operands, combine their result using the Logical AND operator, and include the <iso646.h>` library to use ‘and’ macro.
Code:
Output:
Explanation:
We have taken two numbers in the above code, checked some conditions, and combined their result using the Logical AND operator. Also, we have included the <iso646.h> library to use the ‘and’ macro instead of the ‘&&’ operator.
- In this example we will discuss or prove ((A && B) && C) equals to (A && (B && C)).
Code:
Output:
Explanation:
In the above code, we have shown the associative property of the Logical 'AND' operator, that is, (A and (B && C)) == ((A and B) && C).
The associative property states that if we have three expressions and the && operator in C is used to combine them, if we combine the first two and take their result value ‘&&’ with the third one, it must be equal to the case where we combine the second and third initially and then apply the && operator in C to the result of them and the first expression.
Learn More
Please refer to the link below to learn more about and explore the logical operators.
Conclusion
- ‘&&’ operator or double ampersand operator is a logical AND operator in C language.
- A logical AND (&&) operator is a binary operator that takes two operands and gives the combined result.
- If both operands are true, then the logical AND operator will return true or 1; otherwise, if any of them is false, it will return false or 0.
- In the <iso646.h> library of C programming language, a macro ‘and’ is an alternative to the ‘&&’ operator. The best reason behind this is that it is simpler to remember.
- Logical AND operator shows the associative property, which means (A && (B && C)) == ((A && B) && C).