FCFS Scheduling Program in C
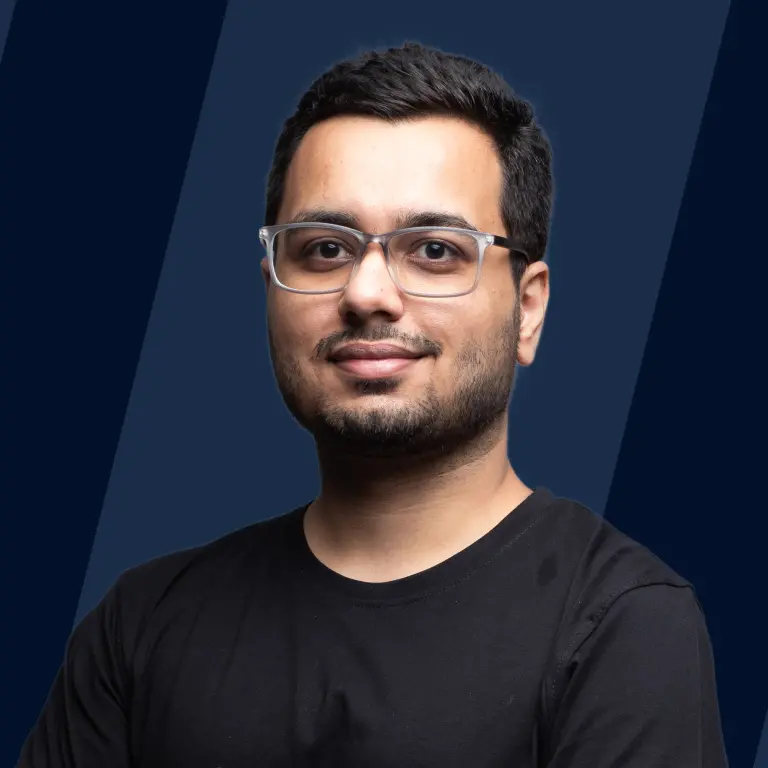
Overview
FCFS stands for 'first-come-first-serve'. As the name suggests, the request which comes first will be processed first and the request that comes after will be served after. FCFS algorithm is a non-preemptive scheduling algorithm that means if there is one process processing in the CPU, then all the other processes will be in a waiting queue and the processes have to wait until the CPU finishes the execution of the current process entirely.
Terms In First Come First Serve Scheduling
To learn First Come First Serve Scheduling in C language, we need to understand some basic terminologies related to this algorithm. Some of them are as follows:
Completion Time
Completion Time is defined as the time required by a process to get executed.
- Completion Time= (Turn Around Time - Waiting Time)
Turn Around Time
The Turn around time is defined as the time taken by a process to complete after the arrival time. Or simply we can say that turnaround time is the difference between the arrival and completion time of a process.
Waiting Time
The waiting time is defined as the difference between the turnaround time and burst time of a process.
What is FCFS Scheduling?
Disk Scheduling Algorithms in an operating system can be referred to as a manager of a grocery store that manages all the incoming and outgoing requests for goods of that store. He keeps a record of what is available in-store, what we need further, and manages the timetable of transaction of goods. Just like that, a 'Disk Scheduling Algorithm' is an algorithm that keeps and manages incoming and outgoing requests from a disk in a system.
And the FCFS ( First Come First Serve) algorithm is one of the simple and easy types of disk scheduling algorithms for an operating system. Suppose there is an n number of processes named P1, P2, P3, P4..., Pn. Then all these processes will be processed at the CPU in a sequential manner first the process P1 will be scheduled by the algorithm and this will get executed and then process P2. and so on. Sometimes, Starvation can be seen in this algorithm if the burst time of a particular job is the longest among all the given jobs.
Algorithm
The algorithm for the FCFS Scheduling program in C is as follows:
- At first, the process of execution algorithm starts
- Then, we declare the size of an array
- Then we get the number of processes that need to insert into the program
- Getting the value
- Then the first process starts with its initial position and the other processes are in a waiting queue.
- The total number of burst times is calculated.
- The value is displayed
- At last, the process stops.
Example
Let us see an example of some processes that are arriving in a sequence. Let's say there are four processes named P2, P3, and P1. Each of these tasks is arriving in accordance with their execution time. Given below is a table showing the jobs, execution time, and order of arrival.
Process | Order of Arrival | Execution Time (in sec) |
---|---|---|
P1 | 3 | 15 |
P2 | 1 | 3 |
P3 | 2 | 3 |
According to the above table, the waiting time of process P2 is 0, the waiting time of process P3 is 3 and the waiting time of process P1 is 6. So we will calculate the average time as: Average time = (0 + 3 + 6)/3 = 3m sec Here the arrival time is taken as 0 so the turnaround time and the completion time are the same.
Code Implementation
Now, let us see how the concept of the algorithm of the FCFS Scheduling program in C can be implemented in the c language.
Output:
Explanation
In the above example, we saw how the FCFS scheduling algorithm can be implemented using the C language. At first, the total number of processes is taken as input by the user. After entering the number of processes, we need to provide the burst time for every number of processes that we entered first. And the waiting time is calculated according to the given inputs. The waiting time is calculated with the help of the burst time provided.
Then the turnaround time is calculated. For each of the given burst times and waiting times, the turnaround time is calculated by adding them. Then the average turnaround time andwaiting time is calculated in this part:
This calculation gives us the total number of processes present there. For getting the average time, the sum of waiting times and turnaround times are divided. This is the working of the algorithm of the FCFS Scheduling program in C.
Advantages of FCFS Scheduling
- Implementation is easy the concept of FCFS is that the job that comes first will be served first.
- In FCFS Scheduling program in C, there is no chance of starvation.
- FCFS Scheduling program in C is the simplest form of scheduling algorithm in an operating system.
Disadvantages of FCFS Scheduling
- In the algorithm of the FCFS Scheduling program in C, the 'Seek time' increases.
- This algorithm is not so efficient because of its simplicity.
Conclusion
- An operating system manages and handles all the processes and works related to the process.
- The operating system used various algorithms for scheduling and executing a task to the CPU.
- The FCFS (First Come First Serve) algorithm used the concept that the jobs will be gets executed on the basis of the timing of their arrival.
- The job that arrives first will gets executed first and the job that arrives late will get executed later.
- The algorithm of the FCFS Scheduling program in C is easy and simple to implement.
- In this algorithm, the Seek Time increases.
- The average waiting time of FCFS is not optimal.
- This algorithm can not use the CPU parallelly for multiple jobs.
Learn More
To learn more about various disk scheduling algorithms, you can click here: Scheduling Algorithms in OS