C Program to Convert Octal to Decimal
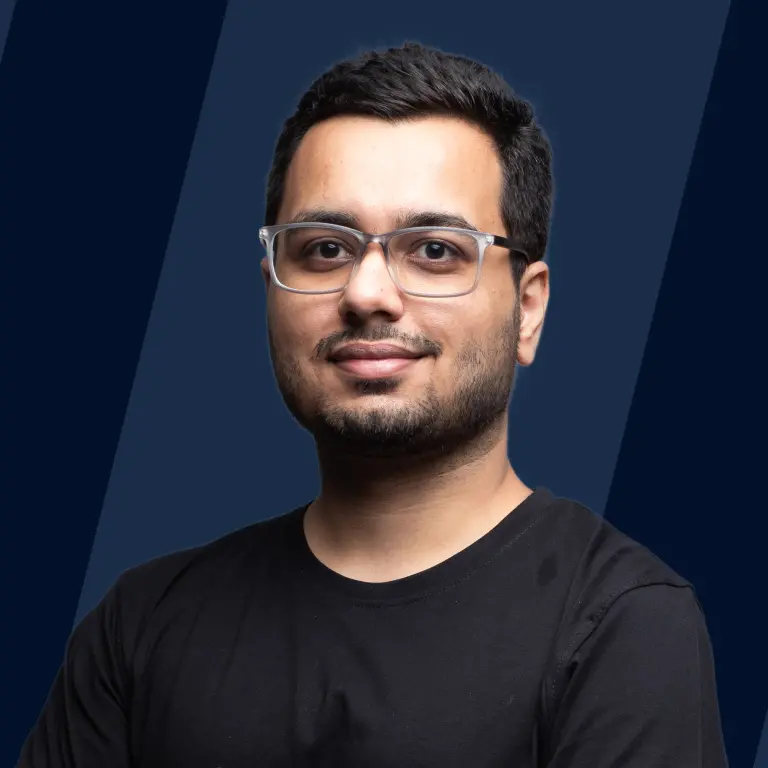
Overview
In this article, we are going to discuss the conversion of octal to decimal in C. The term "octal to decimal conversion" refers to the process of converting an octal number with an 8-base to a decimal number with a 10-base.
Octal Number System: Octal is a base-eight number system. There are eight different digits to work with, from 0 to 7. These eight digits make the octal system a base-eight system.
Decimal Number System: In the decimal system, we work with ten different digits, 0 to 9. These ten digits make the decimal system a base-ten system.
We need to write a program that takes an octal number as input and converts it to a decimal equivalent.
Example:
Introduction
The base number is used to convert octal to decimal in C, as it is for any other number conversion. To convert an octal number system to a decimal number system, multiply each octal digit by the power of 8, starting on the rightmost digit and multiplying it with 8 to the power zero then move left, for every next digit increase power of 8. Sum up all the products to get the decimal number.
Consider the diagram below to see how to convert octal to decimal.
Algorithm to Convert Octal to Decimal
The concept is to extract the digits of an octal number starting with the rightmost digit and store them in a variable called ans. Multiply the digit with the appropriate base (Power of 8) and add it to the variable ans when extracting digits from an octal number. The variable ans will ultimately hold the requisite decimal number.
Steps: Assume that num is the input octal number.
- Initialize x = 0, ans = 0.
- Repeat the following steps while num > 0.
- Set -> y = num % 10, y will have last digit.
- Remove last digit of number in num variable -> num = num / 10.
- ans = ans + y * 8^x
- updated vale of x varable by 1 till num > 0 -> x = x + 1
- Return ans.
Program to Convert Octal to Decimal in C
Output:
Explanation:
In OctalToDecimal function, While loop will traverse over the number and extract the digits from it then add the product of the digit and appropriate power of 8 to the ans. In the end, ans will have the converted decimal number which OctalToDecimal() returns.
Conclusion
- We have discussed the algorithm of C program to convert octal to decimal.
- We also have discussed how to convert octal to decimal numbers.
- Octal number is a base-eight number system.
- Decimal number is a base-ten number system.
- If the Octal Number input is a char array, we must first convert it to integer form.