C Program to Count the Number of Occurrences of a Character in a String
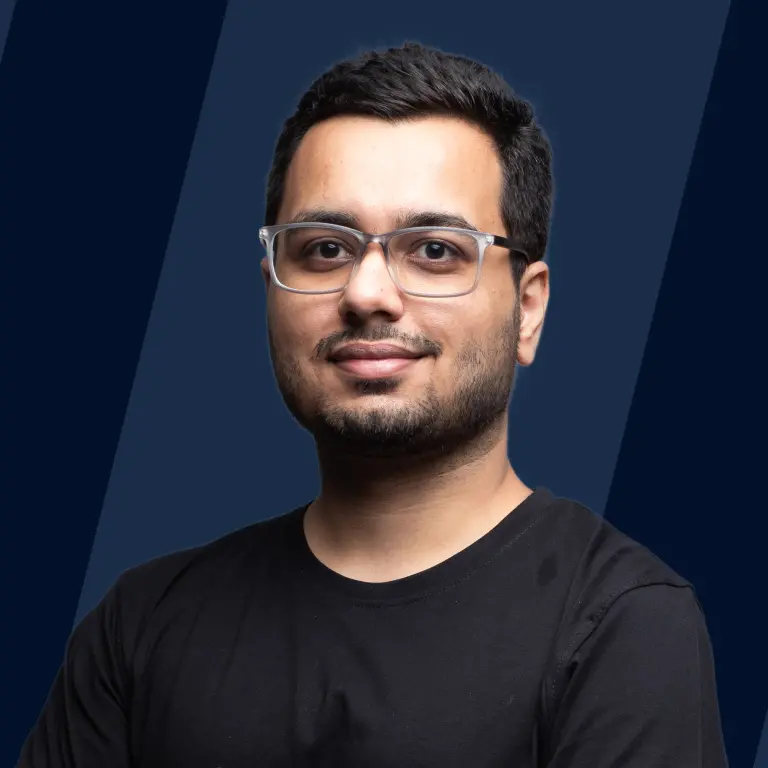
Overview
The total number of occurrences of a character in a string can be calculated by iterating through the string and comparing each character with the character whose occurrence we are counting and increasing the count when the character matches. In this article, we will learn how to write a c program to count the number of occurrences of a character in a string.
Introduction
Suppose we are given a string and we are expected to tell about the number of occurrences of vowels thus we would return the sum of the number of occurrences of {a, e, i, o, u} in the string, or suppose we are given a string and we have to check if a character is present in it or not, thus we would see if the character is present in more than 0 times and return the result accordingly.
In the above cases, there is one thing in common, we are supposed to deal with the number of occurrences of a particular character or characters in a string.
In programming, we often encounter situations where we have to tell the frequency of a character in a string. In the C language, there are many ways to obtain the character count in the given string. We can obtain the character count by looping through the string and comparing each character with our desired character (the character whose occurrence is to be counted).
In this article we will learn intuition as well as a depth explanation of how to write a c program to count the number of occurrences of a character in a string
C program to Count the Number of Occurrences of a Character in a String
Using Standard Method
Algorithm:
- Step 1: We will declare a cnt variable of integer type and assign it a value 0. This variable will keep the count of the occurrence of the character we are counting in the given string.
- **Step 2: **We will iterate through the string. On each iteration, we will compare the current character with the character whose occurrence we are counting in the given string. If the if condition is true then we will increment the count by 1.
- **Step 3: **Once the iteration is completed we will output the cnt variable.
Input:
Output:
Explanation of the example:
In the above example, we are counting the occurrence of character i in mississippi. Thus the c program to count the number of occurrences of a character in a string will go through each character of the string and compare it with i and in case it matches then it will increment cnt. Thus the final result is 4.
Using Function
Algorithm:
- Step 1: We will declare a cnt variable of integer type and assign it a value 0. This variable will keep the count of the occurrence of the character we are counting in the given string.
- Step 2: We will iterate through the string. On each iteration, we will compare the current character with the character whose occurrence we are counting in the given string. If the if condition is true then we will increment the count by 1.
- Step 3: Once the iteration is completed we will output the cnt variable.
Code:
Input:
Output:
Explanation of the example:
In the above example, we are counting the occurrence of character e in Peter. Thus the c program to count the number of occurrences of a character in a string will go through each character of the string and compare it with i and in case it matches then it will increment cnt. Thus the final result is 2.
Using Recursion
Algorithm:
- Step 1: We will declare a cnt variable of integer type and an i variable of integer type and assign them a value 0. The cnt variable will keep the count of the occurrence of the character we are counting in the given string and the i variable will refer to the current index of the string. Both cnt and i are declared static since their scope needs to be global and their value should be maintained upon each function call.
- Step 2: We will compare if str[i] is 0. This means that the iteration is completed, in that case, we will return the cnt.
- Step 3: If the if condition returns false then we will compare the current character with the character whose occurrence we are counting in the given string. If the inner if condition is true then we will increment the count by 1.
- Step 4: We will increment the value of i and call the function countNoOfOccurence recursively.
Code:
Input:
Output:
Explanation of the example:
In the above example, we are counting the occurrence of the character a in business. Thus the c program to count the number of occurrences of a character in a string will go through each character of the string and compare it with i and in case it matches then it will increment cnt. Since there is no occurrence of a in business thus the final result is 0.
Conclusion
- The occurrence of a character in a string in c is counted by looping through the string and comparing each character.
- The main idea behind a c program to count the number of occurrences of a character in a string is to keep count of the appearance of the character.
- We can write a c program to count the number of occurrences of a character in a string either by the standard method or with the use of conventional as well as recursive functions.