realloc() in C
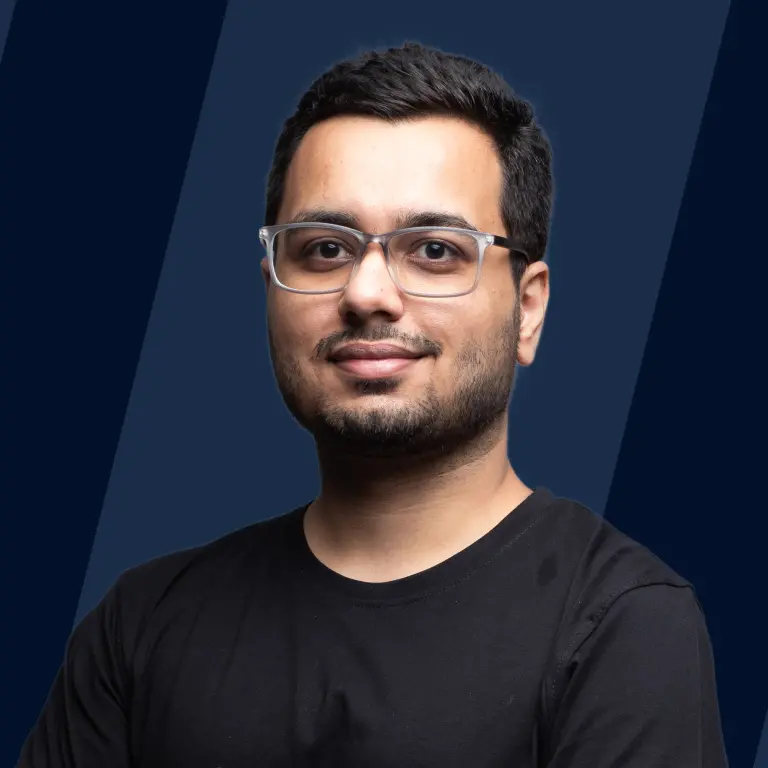
Overview
In the C programming language, along with static memory, there is a concept of dynamic memory. There are functions in C programming that are used for dynamic memory allocation, and one of them is the realloc() function.
Description
The realloc() function in the C programming language is used to resize the memory block pointed to a pointer that was previously allocated to the variable by the malloc() or calloc() function. Realloc stands for reallocation.
Malloc: malloc() is a function in C programming language that is used to dynamically allocate a single large block of memory with a specific size. Malloc stands for memory allocation.
Calloc: calloc() is a function in C programming language that is used to dynamically allocate a contiguous specific number of blocks of a specific size. Calloc stands for contiguous allocation.
Declaration
Parameters
The C realloc() Function accepts 2 parameters as an argument. The details of the parameters are given below
-
ptr − It is the pointer of the memory block which was previously allocated to the calloc(), malloc(), or realloc() function that is to be reallocated. If this pointer is NULL, then a new block is allocated and the pointer to it is returned by the realloc() function.
-
size − It is the new size of the memory block which is to be reallocated. It is passed in bytes. If the size is 0, then the memory block pointed by ptr is deallocated and a NULL pointer is returned by the realloc() function even if ptr points to an existing block of memory.
Return Value
If the realloc request is successful, then it will return a pointer to the block of newly allocated memory. If the request fails, it will return a NULL pointer.
Exceptions
The exception of the realloc() is that this function should only be used for allocating the memory dynamically. If the memory is not dynamically allocated, then it will show an undefined behavior and the program may contain garbage values.
Examples
Example 1
In this example, we will see how to use the realloc() function for int variables and pointers.
C:
Output
Example 2
In this example, we will see how to use the realloc() function for char variables and pointers.
C:
Output
Example 3
In this example, we will see how to use the realloc() function for float variables and pointers.
C:
Output
Example 4
In this example, we will see how to use the realloc() function for double variables and pointers.
C:
Output
How Does realloc() Function Work in C?
Case 1
If the pointer variable is NULL, then the realloc() function will work like the malloc() function.
C:
Output
Case 2
If the new size passed is smaller than the actual size, then the realloc() function will simply reduce the memory size of the block.
C:
Output
Case 3
If the new size passed is larger than the actual size, then the realloc() function will check whether the already available memory can be expanded or not. If yes, then it will simply reduce the memory size of the block.
C:
Output
Uses of realloc() Function in C
The realloc() function in the C programming language is mainly used for deallocating the old memory of the variable pointed to by the pointer and then it returns a pointer to a new variable that has the size specified by size. The contents of the new variable are the same as that of the old variable which was before deallocation. In simple words, we can say that it is used to resize the memory which is allocated by the malloc() or calloc() functions to a variable.
Conclusion
In this quick tutorial, we have discussed the realloc() function in the C programming language. We can extract the following conclusions from the article -
- The syntax and parameters of the C realloc() function
- The return value of the C realloc() function
- Exception of the C realloc() function
- Uses of the C realloc() function
- Working of the C realloc() function for different data types with examples