Caesar Cipher Program in C
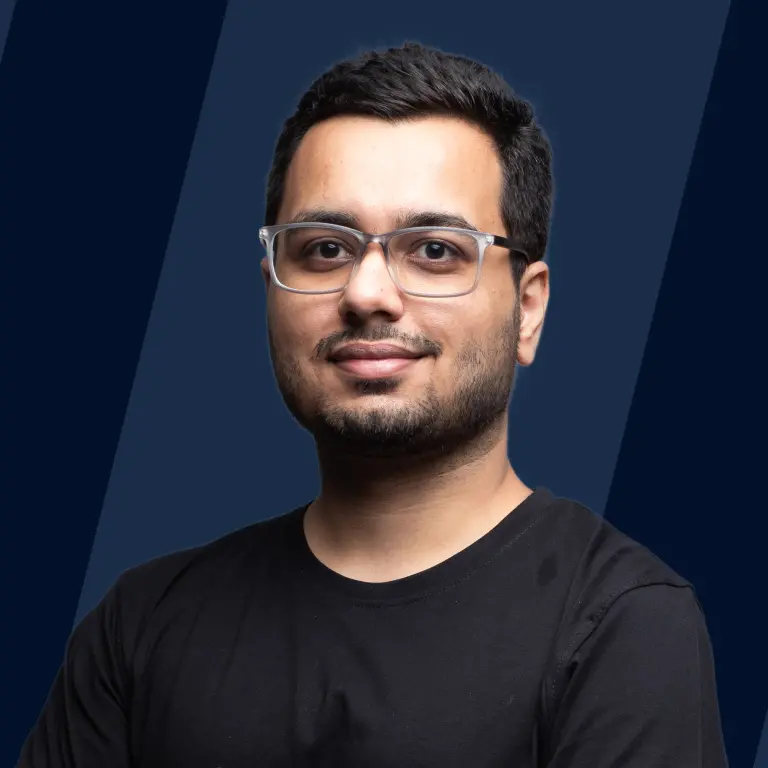
The 'Caesar Cipher,' named after Julius Caesar, stands as one of the oldest cryptography techniques, developed around 100 BC. Utilized by Caesar for secure communication with his generals, the Caesar Cipher provided a strategic advantage by encrypting messages. Despite its simplicity, the Caesar Cipher program in C remains a foundational method for both encryption and decoding. Operating as a shift cipher, it cyclically shifts characters in the data. In this article, we delve into the concept of the Caesar Cipher, exploring its encoding and decoding processes. While historically significant, the Caesar cipher's weakness lies in its vulnerability to hacking, making it easily decryptable. Despite its antiquity, understanding the Caesar cipher contributes to foundational knowledge in cryptography.
Formula for Encryption and Decryption
Let us derive the encryption formula for Caesar Cipher Program in C. Firstly, for normal cases, if the key is represented by k and the character by ch, we add both to get the encrypted message.
For extreme cases, we need to start counting from the beginning if the count exceeds z, so we first try to find the shift. To do so, we subtract a, i.e., the initial character of the series of the alphabet. Now we take modulus % with 26, i.e., the number of alphabets. Lastly, we add a to get the final answer.
So, our formula becomes (ch - 'a' + key) % 26 + 'a'.
Similarly, instead of adding the key, we subtract it for decryption. Now, there is the possibility of getting a negative answer here during subtraction. So, we add 26, and the formula becomes (ch - 'a' - key + 26) % 26 + 'a'.
In the same manner for capital letters and numbers, the formulae are:
Category | Encryption | Decryption |
---|---|---|
Small Letters | (ch - 'a' + key) % 26 + 'a' | (ch - 'a' - key + 26) % 26 + 'a' |
Capital Letters | (ch - 'A' + key) % 26 + 'A' | (ch - 'A' - key + 26) % 26 + 'A' |
Digits | (ch - '0' + key) % 10 + '0' | (ch - '0' - key + 10) % 10 + '0' |
Let's see an example of a message consisting of only digits, encryption, and decryption. The ASCII codes for all the digits are given below:
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
---|---|---|---|---|---|---|---|---|---|
48 | 49 | 50 | 51 | 52 | 53 | 54 | 55 | 56 | 57 |
Let the message be 7930 and the key be 4.
Code | ASCII value (x) | x+key | x-'0' (x-48) | x%10 | x+'0' | Encoded Character |
---|---|---|---|---|---|---|
7 | 55 | 59 | 11 | 1 | 49 | 1 |
9 | 57 | 61 | 13 | 3 | 51 | 3 |
3 | 51 | 55 | 7 | 7 | 55 | 7 |
0 | 48 | 52 | 4 | 4 | 52 | 4 |
Similarly, decoding can be performed for 1374 to return the original message.
Code | ASCII value (x) | x-key | x-'0' (x-48) | x+10 | x%10 | x+'0' | Decoded Character |
---|---|---|---|---|---|---|---|
1 | 49 | 45 | -3 | 7 | 7 | 49 | 7 |
3 | 51 | 47 | -1 | 9 | 9 | 51 | 9 |
7 | 55 | 51 | 3 | 13 | 3 | 55 | 3 |
4 | 52 | 48 | 0 | 10 | 0 | 52 | 0 |
Thus, using formulae, i.e., Ceaser Cipher's method, we can encode the message safely, send it, and decode it by only authorized users.
Program for Caesar Cipher in C
Caesar Cipher Encryption (Explain, Syntax & Output)
The Caesar Cipher Program in C can be applied as given below. We will check each character one by one to see if it is a lowercase character, an uppercase character, or a digit. We have an above-derived formula for each of them which we can use to encrypt as per the key given by the user.
Apply the above-derived formulae for each category. If the given character is an alphanumeric value, only we try encoding it. Else we print an error message.
Output:
We first check if the character is alphanumeric using the isalnum() method, which returns true if the given character is an alphanumeric value, i.e., either lowercase or uppercase alphabets or numbers. Then we check if the character is lowercase, uppercase, or a number using islower(), isupper(), and isdigit(), respectively. Accordingly, conversion is processed. Lastly, the encrypted character is added to the text at its position.
Caesar Cipher Decryption (Explain, Syntax & Output)
Similarly, we can write Caesar Cipher Program in C for decoding. Instead of adding the key subtract it and add 26 so that we don't get a negative value as our answer.
Output:
The example above returns the value we inserted to encode in the above encoding C code in the previous section.
Advantages of Caesar Cipher
Caesar Cipher Program in C has various advantages due to its simplicity as follows:
- It is a simple algorithm and very easy to implement.
- Only a small amount of computing resources are required.
- Single key is used for the entire message, which makes it less complex.
- It is very much suitable for non-complex systems.
Disadvantages of Caesar Cipher
Caesar Cipher Program in C also has a few disadvantages as it is simple and old such as:
- If the key is broken, the entire message can be decrypted.
- As it is simple, it can be decoded using some brute force algorithm.
- There is the possibility of the message being hacked.
FAQs of Caesar Cipher Program in C
Q: What are Encryption and Decryption?
A: Encryption is the process of translating plaintext data into codes. These codes appear random and meaningless to unauthorized people. Decryption converts the ciphertext, i.e., coded text, back to plaintext.
Q: What is Cryptography?
A: Cryptography is a technique of securing information and communications using codes and patterns that can be processed to original data only by those persons for whom the information is intended.
Q: What is the difference between ciphertext and plain text?
A: The ciphertext is encoded text, whereas plaintext is the raw information or communication.
Q: How many different shifts are possible using cipher shifts in the context of alphabets?
A: There is 26 alphabet in the English language. The number of possible shifts has to be one less than the total number of alphabets. Thus, a total of 25 shifts are possible. 26^th^ shift will be where the key is 0; thus, it doesn't change the message, and technically we are not shifting anything.
Q: Is it possible to derive decryption using encryption?
A: Yes, it is possible to derive decryption using encryption. The cyclic property of Caesar Cipher Program in C under modulo can be used to decrypt the message by using the encryption formula itself. The formula for decryption is: Decryption = Encryption(26-n)
Conclusion
- The Caesar Cipher program in C is a simple and old method to convert data into secret code. It provides a method to encrypt and decrypt the given information.
- It shifts the current character to a couple of characters in a cyclic manner.
- As it is simple, it is easy to implement and, thus, also easy to crack. It is friendly for non-complex systems.