Caesar Cipher Program in Java
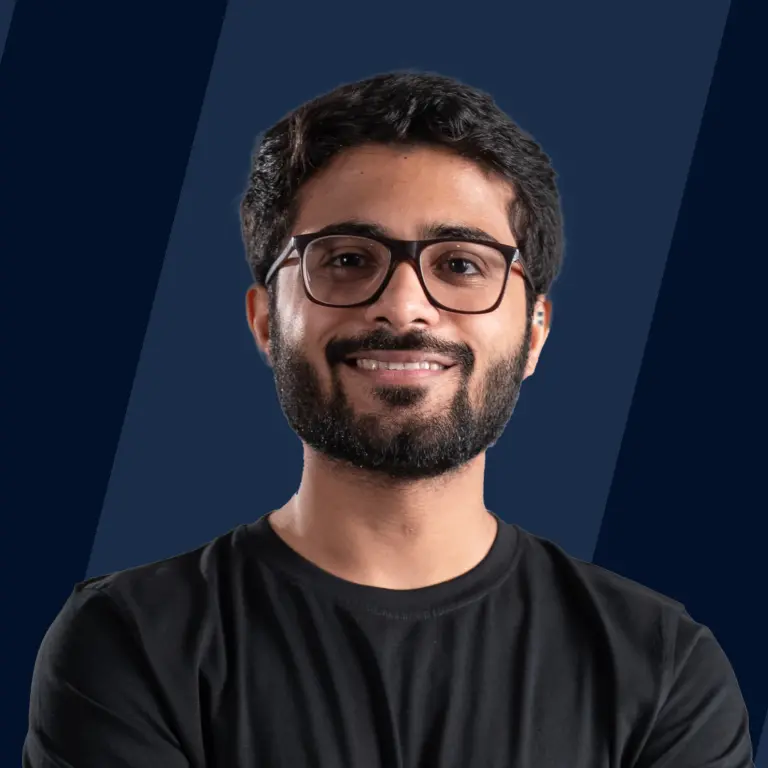
Caesar Cipher is a encryption techniques which is one of the simplest and most widely used, named after Julius Caesar.
It is a type of substitution cipher in which each letter is replaced by another letter at a fixed number of positions down the alphabet system. For example, if the fixed number is 3, A is replaced by D, B is replaced by E, C is replaced by F, and so on.
What is Caesar Cipher Technique?
What is a Cipher?
A cipher used to encrypt messages for various purposes.
Caesar cipher is a cipher that transforms a message by shifting letters by a given constant offset.
If we shift the English alphabet by 3 letters for every letter, the following transformation would occur.
And so on.
Once we reach the letter Z, we loop back to the beginning of our alphabet, and so on.
Here is the complete matching between the original and transformed letters for an offset of 3 :
And so when we shift 24 by 3, we’re shifting it by 1 ahead, and the remaining two shifts start from 0. Hence, the final value is 24 -> 2 for an offset of 3. In general,
TERMINOLOGY
- Encryption :
It’s a process of encoding a message using an encryption algorithm that can only be accessed via an authorized person. - Decryption :
It’s converting an encrypted message to its original form. - Plaintext :
The message that needs to get to the end user might or might not be formatted. - Ciphertext :
The text that results after an encryption algorithm is applied to the plaintext. - Shift :
Integer between 0-25, which tells us the number of shifts to be applied on a character.
Algorithm for Caesar Cipher
Now, let’s understand how the Caesar cipher program in Java works.
We create a CaesarCipher class, which holds the cipher() method, which takes offset and message as parameters.
Let’s suppose the message contains lowercase letters and space with a positive offset, shifting characters by the offset we have :
Output:
Explanation:
- We are providing an offset of 3. Hence, each letter of the English alphabet will shift by 3 letters, as shown in the image below.
- Hence, in the output, I has shifted to L, e has shifted to h, and so on.
Let’s try again with an offset of 10. Provide 10 as input when the program prompts:
Output:
The modulo operation can handle a larger offset for the Caesar cipher in the Java program. The value of newAlphabetPosition cannot exceed 25 as it is under modulo 26.
Encryption
We know that encryption is the process of encoding a message. As shown below, we can demonstrate encryption using modular arithmetic by transforming letters into numbers. Assuming A is represented as 0, B as 1 and so on till Z = 25.
Encryption of a letter by the shift of n can be put mathematically as:
- We understand from the formula that x equals where x is a character and n is a shift number.
- The resulting number will be taken under modulo division to make sure the character starts from the beginning of the alphabet in case it reaches the end of the alphabet.
Code
Output:
Explanation:
Note: We are only handling encryption of lowercase letters in the program above.
A final string alpha is declared to assign numbers to each lowercase of the English alphabet.
Message and shiftKey are then passed as arguments to the encrypt() function :
The provided message is then converted to lowercase using toLowerCase() as we handle only lowercase letters. We then iterate upon the provided message, and for each of its characters, we determine its position charPosition using the string alpha.
The keyVal is calculated using the En(x) = (x + n) % 26 formula, and the character at that position replaceChar is then added to the string cipherText.
Decryption
In Decryption, we’ll transform cipher text back to its original form.
If the encrypted text is CDEF and shiftKey is 2, which was used to encrypt the original message, then:
Which implies our original text was ABCD.
Decryption of a letter by the shift of n can be put mathematically as:
The if statement handles the case when the decrypted character has to come from the end of the English lowercase alphabet.
Code
Output:
Initially, the process for decrypting a text is the same as encryption: 2 strings message and shiftKey are passed as arguments to the function decrypt().
The provided message is first converted to lowercase using the toLowerCase() method, as we only handle lowercase characters. On iteration, we obtain a keyValue, which is the position of the decrypted character in alpha.
If the keyValue comes out negative, alpha's length, i.e. 26, is added to it.
Finally, the character present at keyVal i.e. replaceVal is added to message.
Conclusion
- Caesar cipher is an encryption method that shifts the letters of a message by a constant offset for producing an encrypted message.
- Encryption is a process of encoding a message using an encryption algorithm.
- Decryption is converting an encrypted message to its original form.
- A Cipher is a method that is used for message encryption for various purposes.