How to Calculate Power of a Number in C++
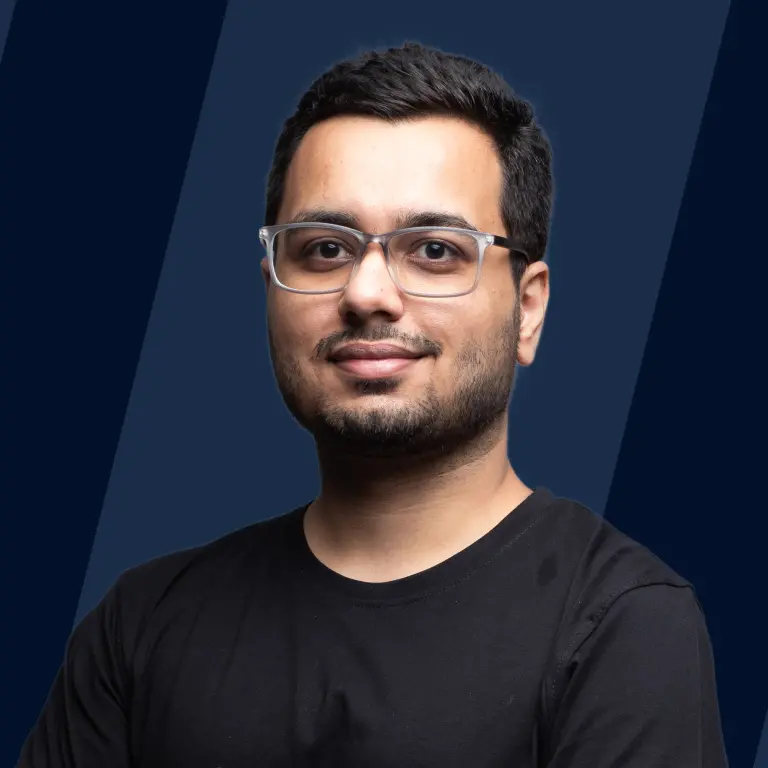
Power is defined as an expression that denotes repeated multiplication of the same factor that is represented as .
For example, let us say a=3 and n=2. Then, where is
Methods in C++ for this include utilizing the pow() function from <cmath>, employing while or do...while loops, or using recursion.
Program to Calculate Power of a Number Using pow() Function
Let us learn how to calculate the power of a number using the pow() function from the <cmath> library in C ++.
With the below code, we shall be using the pow() function which can be described as a function that helps to compute a base number raised to the power of an exponent. We shall first be declaring variables that we shall be calling during the code execution. Then we shall make it dynamic by taking input from the user for the base and exponent. Finally, we shall be using the pow() function to calculate the power of the number and print out the same as well.
Example
Output
How Does this Program Work?
In the above code example, we have started with the basic C++ syntax to describe our main function as int main(). We have declared two integer data type variables named base, and exp, and one long data type variable named power. Moving on, we have asked the user to input and enter the base and exponent for which we want to learn how to calculate power. We calculate the power of the received number by using the pow() function from the <cmath> header file library in C ++.
Finally, we print out the output as the power of the number is stored in the power-named variable that we eventually print out by the cout function.
How to Find the Power of a Number Using a loop
Let us learn how to Find the Product of Two Numbers Using a Loop in C ++.
Example
Output
Explanation: In the above code example, we have started with the basic C++ syntax to describe our main function as int main(). We have declared three float data type variables named base, exp, and power where we gave the variable power value as 1. Moving on, we have asked the user to input and enter the base and exponent for which we want to learn how to calculate power. We then calculate the power of the received number by using the for loop function where once the number received by the user is less than the variable 'exp', then it enters the for loop and the equation power = power * base gets calculated for it.
Finally, we print out the output as the power of the number is stored in the power-named variable that we eventually print out by the cout function.
Program to Find the Power of a Number using Recursion in C ++
Let us learn how to Find the Product of Two Numbers Using Recursion in C ++.
You might have heard of the divide and conquer technique which is a well-known computer programming technique. We define a recursive function as a function that makes use of the recursive technique which is a method in which a call is made to itself. This process together is known as recursion. With the help of a recursive function, we can solve the complex problem by dividing it into identical single simple cases which can then be handled easily.
In the below code example, we start by creating our main function int main() and simultaneously we need to declare two integer data type variables. We then calculate the power of the number (that is received from the user) by using the recursion technique where we define the variable power function as a recursive function that dives into the complex problem of solving and finding the power of a number by breaking it into small and simple cases. When the value of integer data type 'd' is 0 it should returns 1 else it should return c * power(c, --d).
Example
Output
Explanation:
In the above code example, we have started with the basic C++ syntax to describe our main function as int main(). We have declared two integer data type variables named base, and exp and one long data type variable named power. Moving on, we have asked the user to input and enter the base and exponent for which we want to learn how to calculate power. We then calculate the power of the received number by using the recursion technique where we define the variable power function as a recursive function that dives into the complex problem of solving and finding the power of a number by breaking it into small and simple cases. When the value of integer data type 'd' is 0 then it returns 1 else it shall return c * power(c, --d).
Finally, we print out the output as the power of the number is stored in the power-named variable which we eventually print out by the cout function.
Conclusion
-
Power is defined as an expression that denotes repeated multiplication of the same factor that is represented as a^n. The a^n can be defined as aaa...*a (n-times) where a is the base raised to power n also called the exponent
-
The three main ways to learn how to calculate the power are:
- Using pow() function from the <cmath> library in C ++.
- Using loops in C++ ( prior knowledge of C++ while and do...while Loop is considered as an added advantage to understanding ten code)
- Using the recursive method.